使用 Prewitt、Scharr 和 Sobel 算子进行边缘检测
在数字图像处理中,用于突出显示数字图像中物体边界/轮廓的技术称为边缘检测。我们可以使用 MATLAB 执行此操作。为此,MATLAB 库中定义了几个不同的内置函数和算子。
在本教程中,我将解释三个这样的算子,即“Prewitt 算子”、“Scharr 算子”和“Sobel 算子”。这三个算子都是 MATLAB 库中常用的基于梯度的边缘检测算子。
在讨论这些用于边缘检测的算子之前,让我们首先简要概述一下边缘检测技术。
什么是边缘检测?
边缘检测是数字图像处理中用于检测数字图像中物体边界的过程。
在边缘检测过程中,图像中发生快速强度变化的区域会被突出显示。
在图像处理领域,边缘检测非常重要,因为它有助于物体识别、图像分割、提取图像中的重要特征、图像质量增强、图像压缩等等。
它常用于各种领域,例如工业应用中的质量控制、医学成像中的肿瘤检测、计算机视觉中的物体检测(例如自动驾驶汽车、机器人等)、遥感、图像编辑等等。
现在,让我们讨论如何在 MATLAB 中使用“Prewitt 算子”、“Scharr 算子”和“Sobel 算子”进行边缘检测。
在 MATLAB 中使用 Prewitt 算子进行边缘检测
Prewitt 算子是一种基于梯度的边缘检测算子,用于检测数字图像中物体的边界。为了检测和突出显示边缘,Prewitt 算子近似于每个像素处的图像强度的梯度。
从技术上讲,Prewitt 算子使用两个 3×3 卷积核来检测边缘。一个用于检测水平边缘,另一个用于检测图像中物体的垂直边缘。这些核如下所示。
水平核
-1 0 1 -1 0 1 -1 0 1
垂直核
0 0 0 1 1 1
这些 Prewitt 核与图像进行卷积以计算每个像素处的梯度强度。其中,水平核计算从左到右的梯度强度变化,即沿水平方向。而垂直核计算从上到下的梯度强度变化,即沿垂直方向。最后,将两个梯度图像组合起来,得到具有突出边缘的结果图像。
Prewitt 算子语法
在 MATLAB 中,要将 Prewitt 算子应用于图像以检测边缘,可以使用“imfilter”函数。它将具有以下语法
out_img = imfilter(in_img, prewitt_kernel);
这里,“in_img”是要执行边缘检测的输入图像。“kernel”是 Prewitt 算子核。
示例 (1)
以下示例演示了 Prewitt 算子在检测图像中边缘的实现。
% MATLAB code to edge detection using Prewitt operator % Load the input image in_img = imread('https://tutorialspoint.com/assets/questions/media/14304-1687425236.jpg'); % Convert input image to grayscale gray_img = rgb2gray(in_img); % Convert the image to double precision for calculation gray_img = double(gray_img); % Specify the Prewitt operator kernels h_kernel = [-1, 0, 1; -1, 0, 1; -1, 0, 1]; % Horizontal Kernel v_kernel = [-1, -1, -1; 0, 0, 0; 1, 1, 1]; % Vertical Kernel % Use the Prewitt operator kernels to calculate gradient intensities h_gradient = imfilter(gray_img, h_kernel); v_gradient = imfilter(gray_img, v_kernel); % Calculate the gradient magnitude gradient_magnitude = sqrt(h_gradient.^2 + v_gradient.^2); % Display the orignal and edge detected images figure; subplot(2, 1, 1); imshow(in_img); title('Original Image'); subplot(2, 1, 2); imshow(uint8(gradient_magnitude)); title('Prewitt Edge Detected Image');
输出
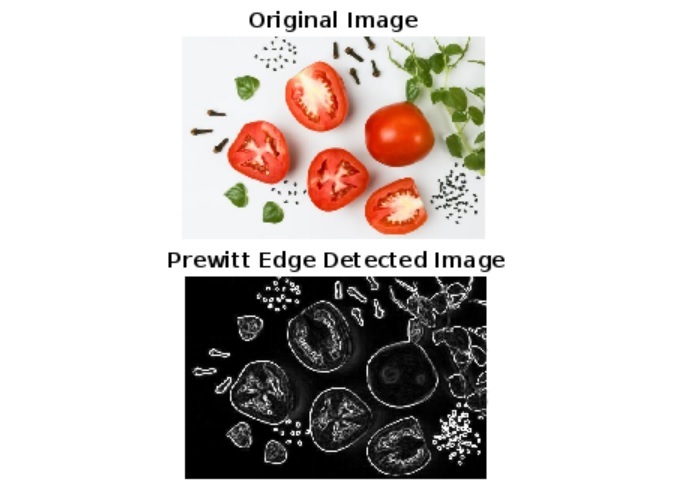
代码说明
在这个 MATLAB 示例中,我们首先读取输入图像,然后将其转换为灰度图和双精度以进行计算。之后,我们定义水平和垂直 Prewitt 核,并使用“imfilter”函数将 Prewitt 算子应用于输入图像以进行边缘检测。
接下来,我们计算梯度幅值,这基本上是图像中检测到的物体边缘的可视化。最后,我们使用“imshow”函数显示原始图像和边缘检测图像。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
在 MATLAB 中使用 Scharr 算子进行边缘检测
与 Prewitt 算子类似,Scharr 算子是 MATLAB 中用于检测图像中物体边界的另一种边缘检测算子。
此算子特别用于必须检测对角线边缘的情况,因为它具有更多旋转对称的边缘检测特性。因此,Scharr 算子在边缘检测中提供了相对更好的结果。
但是,它也是一个基于梯度的边缘检测算子,并使用两个 3×3 卷积核来近似图像每个像素处的梯度强度。这里,一个 Scharr 核用于检测水平边缘,而另一个用于检测垂直边缘。这些 Scharr 核如下所示。
水平 Scharr 核
-3 0 3 -10 0 10 -3 0 3
垂直 Scharr 核
-3 -10 -3 0 0 0 3 10 3
这两个核与图像进行卷积以检测输入图像中物体的边缘。
Scharr 算子语法
在 MATLAB 中,Scharr 算子是使用“imfilter”函数应用的,该函数具有以下语法:
out_img = imfilter(in_img, scharr_kernel);
现在,让我们了解如何在 MATLAB 中实现代码以使用 Scharr 算子执行边缘检测。
示例 (2)
% MATLAB code to perform edge detection using Scharr operator % Load the input image in_img = imread('https://tutorialspoint.com/assets/questions/media/14304-1687425236.jpg'); % Convert the input image to grayscale gray_img = rgb2gray(in_img); % Convert the image to double precision for better calculation gray_img = double(gray_img); % Specify the Scharr operator kernels h_kernel = [-3, 0, 3; -10, 0, 10; -3, 0, 3]; % Horizontal kernel v_kernel = [-3, -10, -3; 0, 0, 0; 3, 10, 3]; % Vertical kernel % Use the Scharr operator kernels to calculate gradients in image h_gradient = imfilter(gray_img, h_kernel); v_gradient = imfilter(gray_img, v_kernel); % Calculate the gradient magnitude gradient_magnitude = sqrt(h_gradient.^2 + v_gradient.^2); % Display the original image and the edge detected image figure; subplot(2, 1, 1); imshow(in_img); title('Original Image'); subplot(2, 1, 2); imshow(uint8(gradient_magnitude)); title('Scharr Edge Detected Image');
输出
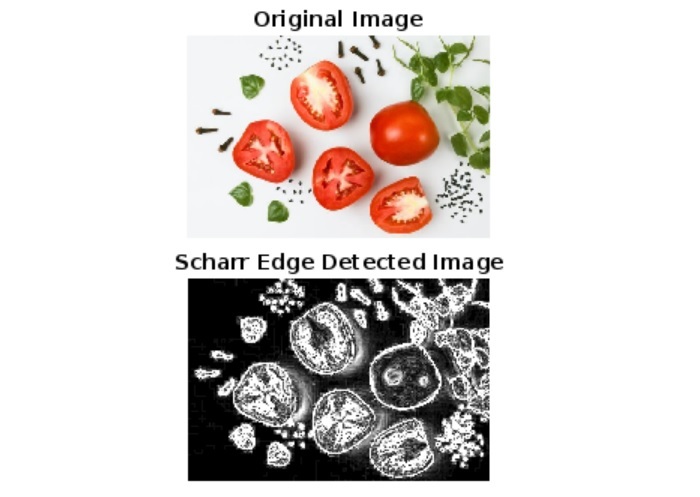
代码说明
此代码的实现类似于 Prewitt 算子的代码。唯一的区别在于,在此示例中,用于边缘检测的核是 Scharr 算子核。从输出图像可以清楚地看出,Scharr 算子提供了更好的结果。
现在让我们了解如何使用 Sobel 算子来检测图像中物体的边缘。
在 MATLAB 中使用 Sobel 算子进行边缘检测
与 Prewitt 和 Scharr 算子类似,Sobel 算子也是数字图像处理中用于检测图像中物体边界的基于梯度的边缘检测算子。此算子也使用两个 3×3 卷积核,即水平 Sobel 核和垂直 Sobel 核。这些 Sobel 核如下所示。
水平 Sobel 核
-1 0 1 -2 0 2 -1 0 1
垂直 Sobel 核
-1 -2 -1 0 0 0 1 2 1
使用 Sobel 算子检测边缘的过程与 Prewitt 和 Scharr 算子的过程类似。
Sobel 算子语法
在 MATLAB 中,Sobel 算子是使用“imfilter”函数应用的。为此,使用以下语法:
out_img = imfilter(in_img, sobel_kernel);
现在让我们举一个例子来了解 Sobel 算子在检测图像中边缘的使用。
示例 (3)
% MATLAB code to detect edges using Sobel operator % Load the input image in_img = imread('https://tutorialspoint.com/assets/questions/media/14304-1687425236.jpg'); % Convert the input image to grayscale gray_img = rgb2gray(in_img); % Convert the grayscale image to double precision gray_img = double(gray_img); % Specify the Sobel operator kernels h_kernel = [-1, 0, 1; -2, 0, 2; -1, 0, 1]; % Horizontal kernel v_kernel = [-1, -2, -1; 0, 0, 0; 1, 2, 1]; % Vertical kernel % Use the Sobel operator kernels to calculate gradients h_gradient = imfilter(gray_img, h_kernel); v_gradient = imfilter(gray_img, v_kernel); % Calculate the gradient magnitude gradient_magnitude = sqrt(h_gradient.^2 + v_gradient.^2); % Display the original image and the edge detected image figure; subplot(2, 1, 1); imshow(in_img); title('Original Image'); subplot(2, 1, 2); imshow(uint8(gradient_magnitude)); title('Sobel Edge Detected Image');
输出
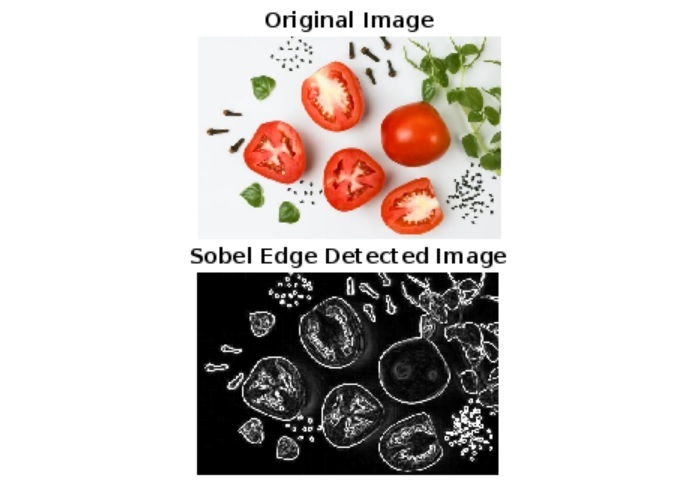
代码说明
代码实现和执行与上述两个示例类似。在这里,我们仅使用 Sobel 算子核来检测边缘。
结论
在本教程中,我借助示例程序解释了所有这些算子。总之,Prewitt、Scharr 和 Sobel 算子用于使用 MATLAB 对数字图像中物体的边缘进行检测。