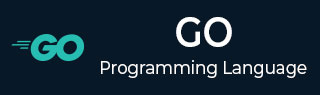
- Go 教程
- Go - 首页
- Go - 概述
- Go - 环境搭建
- Go - 程序结构
- Go - 基本语法
- Go - 数据类型
- Go - 变量
- Go - 常量
- Go - 运算符
- Go - 决策语句
- Go - 循环
- Go - 函数
- Go - 作用域规则
- Go - 字符串
- Go - 数组
- Go - 指针
- Go - 结构体
- Go - 切片
- Go - 范围
- Go - 映射
- Go - 递归
- Go - 类型转换
- Go - 接口
- Go - 错误处理
- Go 有用资源
- Go - 常见问题解答
- Go - 快速指南
- Go - 有用资源
- Go - 讨论
Go - for循环
for 循环是一种重复控制结构。它允许你编写需要执行特定次数的循环。
语法
Go 编程语言中for循环的语法如下:
for [condition | ( init; condition; increment ) | Range] { statement(s); }
for循环的控制流程如下:
如果存在条件,则 for 循环会在条件为真时执行。
如果存在for子句,即( init; condition; increment ),则:
init步骤首先执行,并且只执行一次。此步骤允许你声明和初始化任何循环控制变量。只要有分号,你不需要在这里放置语句。
接下来,评估condition。如果为真,则执行循环体。如果为假,则不执行循环体,控制流跳转到for循环后的下一条语句。
for循环体执行完毕后,控制流跳转回increment语句。此语句允许你更新任何循环控制变量。只要条件后有分号,此语句可以留空。
现在再次评估条件。如果为真,则执行循环并重复此过程(循环体、然后递增步骤、然后再次条件)。条件变为假后,for循环终止。
如果存在range,则for循环会为范围中的每个项目执行。
流程图
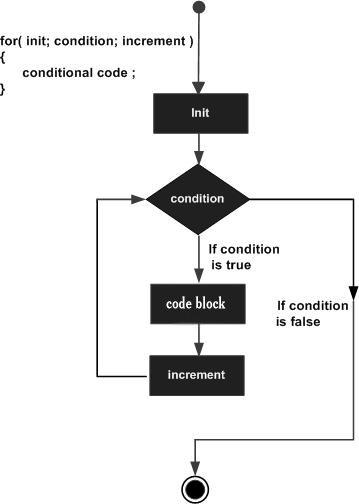
示例
package main import "fmt" func main() { var b int = 15 var a int numbers := [6]int{1, 2, 3, 5} /* for loop execution */ for a := 0; a < 10; a++ { fmt.Printf("value of a: %d\n", a) } for a < b { a++ fmt.Printf("value of a: %d\n", a) } for i,x:= range numbers { fmt.Printf("value of x = %d at %d\n", x,i) } }
编译并执行上述代码后,将产生以下结果:
value of a: 0 value of a: 1 value of a: 2 value of a: 3 value of a: 4 value of a: 5 value of a: 6 value of a: 7 value of a: 8 value of a: 9 value of a: 1 value of a: 2 value of a: 3 value of a: 4 value of a: 5 value of a: 6 value of a: 7 value of a: 8 value of a: 9 value of a: 10 value of a: 11 value of a: 12 value of a: 13 value of a: 14 value of a: 15 value of x = 1 at 0 value of x = 2 at 1 value of x = 3 at 2 value of x = 5 at 3 value of x = 0 at 4 value of x = 0 at 5
go_loops.htm
广告