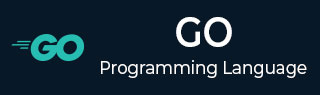
Go - 地图
Go 提供了一种名为 map 的重要数据类型,它将唯一键映射到值。键是你用来在稍后某个时间检索值的物体。给定某个键和值,你可以将值存储在 Map 对象中。存储该值后,你可以使用其键对其进行检索。
定义映射
你必须使用**make**函数来创建映射。
/* declare a variable, by default map will be nil*/ var map_variable map[key_data_type]value_data_type /* define the map as nil map can not be assigned any value*/ map_variable = make(map[key_data_type]value_data_type)
示例
以下示例说明如何创建和使用映射 −
package main import "fmt" func main() { var countryCapitalMap map[string]string /* create a map*/ countryCapitalMap = make(map[string]string) /* insert key-value pairs in the map*/ countryCapitalMap["France"] = "Paris" countryCapitalMap["Italy"] = "Rome" countryCapitalMap["Japan"] = "Tokyo" countryCapitalMap["India"] = "New Delhi" /* print map using keys*/ for country := range countryCapitalMap { fmt.Println("Capital of",country,"is",countryCapitalMap[country]) } /* test if entry is present in the map or not*/ capital, ok := countryCapitalMap["United States"] /* if ok is true, entry is present otherwise entry is absent*/ if(ok){ fmt.Println("Capital of United States is", capital) } else { fmt.Println("Capital of United States is not present") } }
编译并执行上述代码时,其会产生以下结果 −
Capital of India is New Delhi Capital of France is Paris Capital of Italy is Rome Capital of Japan is Tokyo Capital of United States is not present
delete() 函数
delete() 函数用于从映射中删除某个条目。它需要映射和相应的要删除的键。例如 −
package main import "fmt" func main() { /* create a map*/ countryCapitalMap := map[string] string {"France":"Paris","Italy":"Rome","Japan":"Tokyo","India":"New Delhi"} fmt.Println("Original map") /* print map */ for country := range countryCapitalMap { fmt.Println("Capital of",country,"is",countryCapitalMap[country]) } /* delete an entry */ delete(countryCapitalMap,"France"); fmt.Println("Entry for France is deleted") fmt.Println("Updated map") /* print map */ for country := range countryCapitalMap { fmt.Println("Capital of",country,"is",countryCapitalMap[country]) } }
编译并执行上述代码时,其会产生以下结果 −
Original Map Capital of France is Paris Capital of Italy is Rome Capital of Japan is Tokyo Capital of India is New Delhi Entry for France is deleted Updated Map Capital of India is New Delhi Capital of Italy is Rome Capital of Japan is Tokyo
广告