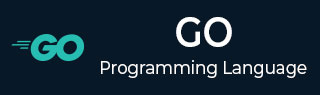
- Go 教程
- Go - 首页
- Go - 概述
- Go - 环境设置
- Go - 程序结构
- Go - 基本语法
- Go - 数据类型
- Go - 变量
- Go - 常量
- Go - 运算符
- Go - 决策
- Go - 循环
- Go - 函数
- Go - 作用域规则
- Go - 字符串
- Go - 数组
- Go - 指针
- Go - 结构体
- Go - 切片
- Go - 范围
- Go - 映射
- Go - 递归
- Go - 类型转换
- Go - 接口
- Go - 错误处理
- Go 有用资源
- Go - 常见问题解答
- Go - 快速指南
- Go - 有用资源
- Go - 讨论
Go - switch语句
switch 语句允许将变量与一系列值进行相等性测试。每个值称为一个 case,系统会检查正在进行切换的变量与每个switch case 是否相等。
在 Go 编程中,switch 语句有两种类型:
表达式 switch - 在表达式 switch 中,case 包含表达式,这些表达式将与 switch 表达式的值进行比较。
类型 switch - 在类型 switch 中,case 包含类型,这些类型将与特殊注释的 switch 表达式的类型进行比较。
表达式 switch
Go 编程语言中表达式 switch 语句的语法如下:
switch(boolean-expression or integral type){ case boolean-expression or integral type : statement(s); case boolean-expression or integral type : statement(s); /* you can have any number of case statements */ default : /* Optional */ statement(s); }
switch 语句适用以下规则:
switch 语句中使用的表达式必须是整数或布尔表达式,或者属于某个类类型,其中该类具有单个转换为整数或布尔值的转换函数。如果未传递表达式,则默认值为 true。
在一个 switch 中可以包含任意数量的 case 语句。每个 case 后面跟着要比较的值和一个冒号。
case 的常量表达式的数据类型必须与 switch 中的变量相同,并且必须是常量或字面量。
当正在进行切换的变量等于某个 case 时,该 case 后面的语句将执行。case 语句中不需要break。
switch 语句可以有一个可选的default case,它必须出现在 switch 的末尾。当没有一个 case 为 true 时,可以使用 default case 来执行任务。default case 中不需要break。
流程图
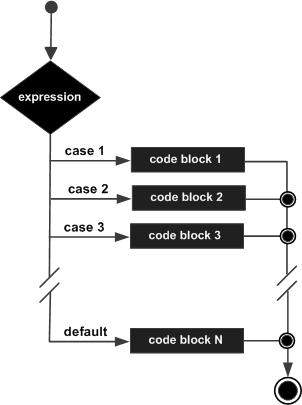
示例
package main import "fmt" func main() { /* local variable definition */ var grade string = "B" var marks int = 90 switch marks { case 90: grade = "A" case 80: grade = "B" case 50,60,70 : grade = "C" default: grade = "D" } switch { case grade == "A" : fmt.Printf("Excellent!\n" ) case grade == "B", grade == "C" : fmt.Printf("Well done\n" ) case grade == "D" : fmt.Printf("You passed\n" ) case grade == "F": fmt.Printf("Better try again\n" ) default: fmt.Printf("Invalid grade\n" ); } fmt.Printf("Your grade is %s\n", grade ); }
编译并执行上述代码后,将产生以下结果:
Excellent! Your grade is A
类型 switch
Go 编程中类型 switch 语句的语法如下:
switch x.(type){ case type: statement(s); case type: statement(s); /* you can have any number of case statements */ default: /* Optional */ statement(s); }
switch 语句适用以下规则:
switch 语句中使用的表达式必须是 interface{} 类型的变量。
在一个 switch 中可以包含任意数量的 case 语句。每个 case 后面跟着要比较的值和一个冒号。
case 的类型必须与 switch 中的变量的数据类型相同,并且必须是有效的数据类型。
当正在进行切换的变量等于某个 case 时,该 case 后面的语句将执行。case 语句中不需要 break。
switch 语句可以有一个可选的 default case,它必须出现在 switch 的末尾。当没有一个 case 为 true 时,可以使用 default case 来执行任务。default case 中不需要 break。
示例
package main import "fmt" func main() { var x interface{} switch i := x.(type) { case nil: fmt.Printf("type of x :%T",i) case int: fmt.Printf("x is int") case float64: fmt.Printf("x is float64") case func(int) float64: fmt.Printf("x is func(int)") case bool, string: fmt.Printf("x is bool or string") default: fmt.Printf("don't know the type") } }
编译并执行上述代码后,将产生以下结果:
type of x :<nil>