如何在NextJS中添加弹窗?
我们可以使用`react-modal`或`reactjs-popup`之类的库在NextJS中添加弹窗。这将允许我们创建一个模态组件,可以轻松地集成到我们的NextJS应用程序中。我们也可以使用CSS来设置弹窗的样式,使其与我们网站的设计相匹配。
让我们首先了解什么是Next.js。Next.js是一个开源的Web开发框架。Next.js是一个基于React的框架,具有服务器端渲染功能。速度和SEO都非常出色。您可以使用Next.js轻松构建和测试复杂的基于React的应用程序。
Next.js是用TypeScript编写的。它提供了一个`Link`组件,用于将其他组件链接在一起,并具有一个`prefetch`属性,允许后台预取页面资源。它支持React组件和JavaScript模块的动态导入。此外,它还允许您导出Web应用程序的整个静态站点。
Next.js允许您从JavaScript文件中导入CSS文件。这是可能的,因为Next.js扩展了`import`语句在JavaScript中的概念。
在Web开发中,弹窗是指出现在当前网页顶部的的小窗口,通常包含其他信息或用户选项。
弹窗可以由各种操作触发,例如单击按钮或链接,悬停在元素上,或通过定时事件。弹窗可以用于各种目的,例如显示消息、表单或广告。它们还可以用于在继续操作之前收集信息或确认操作。可以使用JavaScript和各种库或框架(例如jQuery、AngularJS、React和Vue.js)来实现弹窗。
首先创建一个新的NextJS应用程序,并在我们的开发服务器上运行它,方法如下:
npx create-next-app popup-app cd phone-input npm start
方法
安装`react-modal`包:在您的终端中运行命令`npm install react-modal`。
在您的Next.js组件中导入`react-modal`组件:
import Modal from 'react-modal'
创建一个状态变量来控制模态的可见性:
const [isOpen, setIsOpen] = useState(false)
添加一个按钮或链接来触发模态打开:
<button onClick={() => setIsOpen(true)}>Open Modal</button>
将`react-modal`组件添加到您的JSX:
<Modal isOpen={isOpen} onRequestClose={() => setIsOpen(false)}>
将模态的内容添加到`react-modal`组件中:
<Modal isOpen={isOpen} onRequestClose={() => setIsOpen(false)}> <h1>Modal Content</h1> </Modal>
添加一个按钮或链接来关闭模态:
<button onClick={() => setIsOpen(false)}>Close Modal</button>
使用`style`属性为模态添加样式:
<Modal isOpen={isOpen} onRequestClose={() => setIsOpen(false)} style={customStyles}>
在您的组件中添加`customStyles`变量:
const customStyles = { overlay: { backgroundColor: 'rgba(0, 0, 0, 0.6)' }, content: { top: '50%', left: '50%', right: 'auto', bottom: 'auto', marginRight: '-50%', transform: 'translate(-50%, -50%)' } }
通过单击“打开模态”按钮测试模态,并确保它按预期打开和关闭。
模态正常工作的最终代码将是:
示例
MyComponent.js: import React, { useState } from 'react' import Modal from 'react-modal' const MyComponent = () => { const [isOpen, setIsOpen] = useState(false) const customStyles = { overlay: { backgroundColor: 'rgba(0, 0, 0, 0.6)' }, content: { top: '50%', left: '50%', right: 'auto', bottom: 'auto', marginRight: '-50%', transform: 'translate(-50%, -50%)' } } return ( <div> <button onClick={() => setIsOpen(true)}>Open Modal</button> <Modal isOpen={isOpen} onRequestClose={() => setIsOpen(false)} style={customStyles}> <h1>Modal Content</h1> <button onClick={() => setIsOpen(false)}>Close Modal</button> </Modal> </div> ) } export default MyComponent
Index.js
import { StrictMode } from "react"; import { createRoot } from "react-dom/client"; import MyComponent from "./MyComponent"; const rootElement = document.getElementById("root"); const root = createRoot(rootElement); root.render( <StrictMode> <MyComponent /> </StrictMode> );
输出
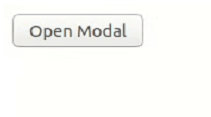