如何在 Python 中自动化 Excel 表格?
Excel 常用于数据存储、分析和展示。另一方面,流行的编程语言 Python 以其易用性、适应性和多功能性而闻名。Python 提供了许多库,可用于与 Excel 电子表格交互并实现各种任务自动化。我们可以轻松地利用 Python 自动化 Excel 电子表格。在本文中,我们将介绍几种不同的方法。
方法
使用 openpyxl 库
使用 pandas 库
使用 xlwings 库
方法 1:使用 openpyxl 库
我们可以使用 Python 的 Openpyxl 包来与 Excel 文件交互。此库允许我们读取、写入和编辑 Excel 文件。
我们可以使用以下命令安装 openpyxl 模块:
pip install openpyxl
假设有一个 Excel 文件,其内容如下:
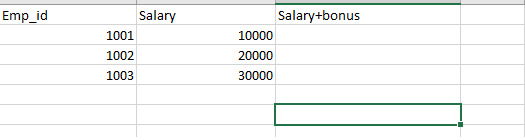
现在,我们想自动为特定员工添加一个新字段,用于存储奖金 + 工资。为此,我们将编写一些基于以下算法的代码。
算法
导入必要的模块
使用 openpyxl.load_workbook() 函数加载 Excel 工作簿 'python_worksheet.xlsx',并将其赋值给变量 'wb'。
使用 wb['Sheet1'] 选择工作簿的第一个工作表,并将其赋值给名为 'sheet' 的变量。
循环遍历工作表中的每一行(从第二行开始)。
使用 sheet.cell(row, 2) 访问当前行的第二列单元格,并将其赋值给变量 'cell'。
使用 sheet.cell(row, 3) 访问当前行的第三列单元格,并将其赋值给变量 'salary_bonus_cell'。
使用 int(cell.value) + 500 将 500 加到 'cell' 的值上,并将结果赋值给 'salary_bonus_cell' 的值,使用 salary_bonus_cell.value = int(cell.value) + 500。
使用 wb.save('python-spreadsheet2.xlsx') 保存修改后的工作簿,并使用新文件名 'python-spreadsheet2.xlsx'。
步骤 1 - 导入必要的模块
import openpyxl as xl from openpyxl.chart import BarChart, Reference
步骤 2 - 加载工作簿并选择工作表
wb = xl.load_workbook('python_worksheet.xlsx') sheet = wb['Sheet1']
步骤 3 - 从第二行开始循环遍历每一行,并根据需要执行计算。
for row in range(2, sheet.max_row + 1): cell = sheet.cell(row, 2) salary_bonus_cell = sheet.cell(row, 3) salary_bonus_cell.value = int(cell.value) + 500
步骤 4 - 使用新文件名保存修改后的工作簿
wb.save('python-spreadsheet2.xlsx')
示例
# Importing the necessary modules import openpyxl as xl # Loading the workbook and selecting the sheet wb = xl.load_workbook('python_worksheet.xlsx') sheet = wb['Sheet1'] # Looping through each row of the sheet starting from row 2 for row in range(2, sheet.max_row + 1): # Accessing the cell in the second column of the current row cell = sheet.cell(row, 2) # Accessing the cell in the third column of the current row salary_bonus_cell = sheet.cell(row, 3) # Adding 500 to the value in the cell from column 2 and updating the value in column 3 salary_bonus_cell.value = int(cell.value) + 500 # Saving the modified workbook with a new filename wb.save('python-spreadsheet2.xlsx')
openpyxl 方法涉及使用 openpyxl 模块直接从 Excel 文件读取数据并写入 Excel 文件。我们了解了如何打开 Excel 文件、编辑其数据并创建新的列值。虽然此方法可用于处理单个单元格以及单元格范围,但由于此库的一些限制,对于比第二种方法更大的数据集,代码可能过于冗长且效率较低。
输出
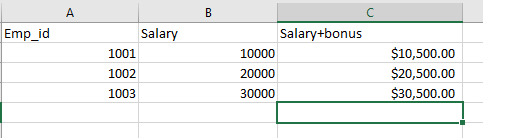
方法 2:使用 pandas 库
Pandas 是一个主要用于数据分析的 Python 包。但是,它也提供了读取和写入 Excel 文件的功能。下面给出了一个使用 pandas 自动化 Excel 表格的示例。
我们可以使用以下命令在 python 中安装 pandas:
pip install pandas
假设有一个 Excel 文件,其内容如下:
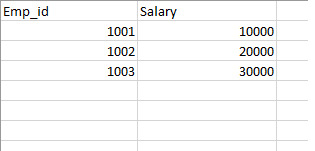
现在,我们想执行与上一个示例中描述的相同的操作,我们将遵循以下算法:
算法
将 pandas 库导入为 pd。
使用 pd.read_excel() 方法从 Excel 文件读取数据。
指定工作表名称“Sheet1”以从 Excel 文件读取数据。
使用 pandas 中提供的各种方法根据需要操作数据。
在 DataFrame 中创建一个名为“Bonus”的新列,该列将 1000 加到“Salary”列。
使用 to_excel() 方法将更新后的数据写回新的 Excel 文件,并指定 index=False 以防止 pandas 将 DataFrame 的索引写入输出文件。
步骤 1 - 使用语句“import pandas as pd”导入 pandas 库。
import pandas as pd
步骤 2 - 使用 pd.read_excel() 方法读取输入 Excel 文件“python_worksheet.xlsx”,并将其存储在名为“data”的变量中。
data = pd.read_excel('python_worksheet.xlsx')
步骤 3 - 在 pd.read_excel() 方法中将工作表名称指定为“Sheet1”,以从输入 Excel 文件的 Sheet1 工作表读取数据。
data = pd.read_excel('python_worksheet.xlsx', sheet_name='Sheet1')
步骤 4 - 通过将 1000 加到现有的“Salary”列,在“data”DataFrame 中创建一个名为“Bonus”的新列。可以使用“+”运算符执行此操作,并将结果保存到新列中。
data['Bonus'] = data['Salary'] + 1000
步骤 5 - 使用 to_excel() 方法将更新后的数据写回名为“python-spreadsheet2.xlsx”的新 Excel 文件。将 DataFrame“data”作为第一个参数传递,并指定 index=False 以防止 DataFrame 的索引被写入输出文件。
data.to_excel('python-spreadsheet2.xlsx', index=False)
步骤 6 - 运行代码并检查输出 Excel 文件“python-spreadsheet2.xlsx”,以验证“Bonus”列是否已添加到原始数据中。
示例
# Import the pandas library import pandas as pd # Read the input Excel file "python_worksheet.xlsx" into a DataFrame called "data" # and specify the sheet name as "Sheet1" data = pd.read_excel('python_worksheet.xlsx', sheet_name='Sheet1') # Create a new column in the "data" DataFrame called "Bonus" by adding 1000 to the existing "Salary" column data['Bonus'] = data['Salary'] + 1000 # Write the updated data back to a new Excel file called "python-spreadsheet2.xlsx" # Pass the DataFrame "data" as the first argument and specify index=False to prevent the DataFrame's index from being written to the output file data.to_excel('python-spreadsheet2.xlsx', index=False)
pandas 方法涉及使用 DataFrame 读取和写入 Excel 文件中的数据。在我们的示例中,我们展示了如何从 Excel 文件中获取数据,自动化添加新列,然后将所有新数据放入另一个 Excel 文件中。由于 pandas 提供了大量用于操作、清理和分析数据的功能,因此即使处理大型数据集,此方法也很有用。
输出
在此示例中,我们使用 python 自动化了 Excel 表格以填充“Bonus”列。
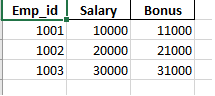
方法 3:使用 xlwings 库
Xlwings 是一个 python 库,它为用户提供了一种从 Python 与 Microsoft Excel 通信和自动化流程的方法。它使用户能够使用 Python 自动化 Excel 活动,例如创建和编辑 Excel 图表和表格,以及编写 Excel 公式和函数。
此外,我们可以使用 Python 函数和库对 Excel 数据进行计算和分析,然后将结果写回 Excel。
我们可以使用以下命令在 python 中安装 **xlwings**:
pip install xlwings
假设有一个 Excel 文件,其内容如下:
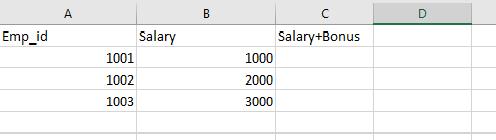
现在,我们想执行与上一个示例中描述的相同的操作,我们将遵循以下算法:
算法
导入 **xlwings** 模块。
将 Excel 文件加载到 **xlwings** Workbook 对象中。
选择工作表并确定“Salary”列的最后一行。
将 500 加到“Salary”列中的每个值以创建一个新的“Bonus”列。
将结果写入相应的“Bonus”单元格。
使用新文件名保存修改后的工作簿。
关闭工作簿
步骤 1 - 导入 **xlwings** 模块。
import xlwings as xw
步骤 2 - 将 Excel 文件加载到 xlwings Workbook 对象中
wb = xw.Book('python_worksheet.xlsx')
步骤 3 - 选择工作表并将 500 加到“Salary”列以创建一个新的“Bonus”列
sheet = wb.sheets['Sheet1'] last_row = sheet.range('B' + str(sheet.cells.last_cell.row)).end('up').row sheet.range('C2:C' + str(last_row)).value = [[cell.value + 500] for cell in sheet.range('B2:B' + str(last_row))]
步骤 4 - 使用新文件名保存修改后的工作簿
wb.save('python-spreadsheet2.xlsx')
步骤 5 - 关闭工作簿
wb.close()
示例
# Import the xlwings module import xlwings as xw # Load the Excel file into an xlwings Workbook object wb = xw.Book('python_worksheet.xlsx') # Select the Worksheet and add 500 to the 'Salary' column to create a new 'Bonus' column sheet = wb.sheets['Sheet1'] last_row = sheet.range('B' + str(sheet.cells.last_cell.row)).end('up').row sheet.range('C2:C' + str(last_row)).value = [[cell.value + 500] for cell in sheet.range('B2:B' + str(last_row))] # Save the modified workbook with a new filename wb.save('python-spreadsheet2.xlsx') # Close the workbook wb.close()
此方法涉及使用 **xlwings** 库,该库允许您通过 Python 代码自动化和操作 Excel。由于此库专门用于处理 Excel 文件,因此我们可以轻松地使用此方法来自动化我们的大型 Excel 任务。
输出
我们已成功在 Excel 表格中添加了“Salary+Bonus”列。
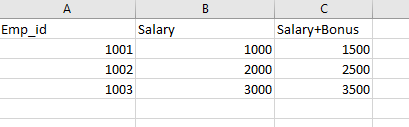
结论
使用 Python 自动化 Excel 表格可以成为处理大型数据集、简化冗余和重复性任务以及生成更大报告的有用工具。在本文中,我们介绍了三种流行的 Python 自动化 Excel 表格的方法。最终,您选择的方法将取决于任务的要求以及数据的数量和复杂性。如果您使用正确的策略和工具,使用 Python 自动化 Excel 表格可以帮助您节省时间、减少人为错误并提高生产力。