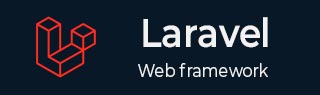
- Laravel 教程
- Laravel - 首页
- Laravel - 概述
- Laravel - 安装
- Laravel - 应用结构
- Laravel - 配置
- Laravel - 路由
- Laravel - 中间件
- Laravel - 命名空间
- Laravel - 控制器
- Laravel - 请求
- Laravel - Cookie
- Laravel - 响应
- Laravel - 视图
- Laravel - Blade 模板
- Laravel - 重定向
- Laravel - 数据库操作
- Laravel - 错误与日志
- Laravel - 表单
- Laravel - 本地化
- Laravel - Session
- Laravel - 验证
- Laravel - 文件上传
- Laravel - 发送邮件
- Laravel - Ajax
- Laravel - 错误处理
- Laravel - 事件处理
- Laravel - 门面 (Facades)
- Laravel - 契约 (Contracts)
- Laravel - CSRF 保护
- Laravel - 身份验证
- Laravel - 授权
- Laravel - Artisan 命令行
- Laravel - 加密
- Laravel - 散列
- 理解发布流程
- Laravel - 游客用户权限
- Laravel - Artisan 命令
- Laravel - 分页自定义
- Laravel - Dump Server
- Laravel - Action URL
- Laravel 有用资源
- Laravel - 快速指南
- Laravel - 有用资源
- Laravel - 讨论
Laravel - 门面 (Facades)
门面提供了一个静态接口,用于访问应用服务容器中提供的类。Laravel 的门面充当服务容器中底层类的静态代理,在保持比传统静态方法更高的可测试性和灵活性的同时,提供了简洁、富有表现力的语法。
如何创建门面
以下是创建 Laravel 门面的步骤:
步骤 1 - 创建 PHP 类文件。
步骤 2 - 将该类绑定到服务提供者。
步骤 3 - 将服务提供者注册到
Config\app.php 作为提供者。
步骤 4 - 创建一个类,该类继承自
Illuminate\Support\Facades\Facade。
步骤 5 - 将步骤 4 中的类注册到 Config\app.php 作为别名。
门面类参考
Laravel 自带许多门面。下表显示了内置的门面类参考:
门面 | 类 | 服务容器绑定 |
---|---|---|
App | Illuminate\Foundation\Application | app |
Artisan | Illuminate\Contracts\Console\Kernel | artisan |
Auth | Illuminate\Auth\AuthManager | auth |
Auth (实例) | Illuminate\Auth\Guard | |
Blade | Illuminate\View\Compilers\BladeCompiler | blade.compiler |
Bus | Illuminate\Contracts\Bus\Dispatcher | |
Cache | Illuminate\Cache\Repository | cache |
Config | Illuminate\Config\Repository | config |
Cookie | Illuminate\Cookie\CookieJar | cookie |
Crypt | Illuminate\Encryption\Encrypter | encrypter |
DB | Illuminate\Database\DatabaseManager | db |
DB (实例) | Illuminate\Database\Connection | |
Event | Illuminate\Events\Dispatcher | events |
File | Illuminate\Filesystem\Filesystem | files |
Gate | Illuminate\Contracts\Auth\Access\Gate | |
Hash | Illuminate\Contracts\Hashing\Hasher | hash |
Input | Illuminate\Http\Request | request |
Lang | Illuminate\Translation\Translator | translator |
Log | Illuminate\Log\Writer | log |
Illuminate\Mail\Mailer | mailer | |
Password | Illuminate\Auth\Passwords\PasswordBroker | auth.password |
Queue | Illuminate\Queue\QueueManager | queue |
Queue (实例) | Illuminate\Queue\QueueInterface | |
Queue (基类) | Illuminate\Queue\Queue | |
Redirect | Illuminate\Routing\Redirector | redirect |
Redis | Illuminate\Redis\Database | redis |
Request | Illuminate\Http\Request | request |
Response | Illuminate\Contracts\Routing\ResponseFactory | |
Route | Illuminate\Routing\Router | router |
Schema | Illuminate\Database\Schema\Blueprint | |
Session | Illuminate\Session\SessionManager | session |
Session (实例) | Illuminate\Session\Store | |
Storage | Illuminate\Contracts\Filesystem\Factory | filesystem |
URL | Illuminate\Routing\UrlGenerator | url |
Validator | Illuminate\Validation\Factory | validator |
Validator (实例) | Illuminate\Validation\Validator | |
View | Illuminate\View\Factory | view |
View (实例) | Illuminate\View\View |
示例
步骤 1 - 通过执行以下命令创建名为TestFacadesServiceProvider的服务提供者。
php artisan make:provider TestFacadesServiceProvider
步骤 2 - 成功执行后,您将收到以下输出:
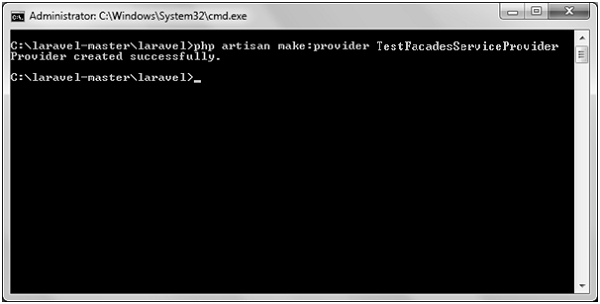
步骤 3 - 在App/Test目录下创建名为TestFacades.php的类。
App/Test/TestFacades.php
<?php namespace App\Test; class TestFacades{ public function testingFacades() { echo "Testing the Facades in Laravel."; } } ?>
步骤 4 - 在“App/Test/Facades”目录下创建名为“TestFacades.php”的门面类。
App/Test/Facades/TestFacades.php
<?php namespace app\Test\Facades; use Illuminate\Support\Facades\Facade; class TestFacades extends Facade { protected static function getFacadeAccessor() { return 'test'; } }
步骤 5 - 在App/Test/Facades目录下创建名为TestFacadesServiceProviders.php的门面类。
App/Providers/TestFacadesServiceProviders.php
<?php namespace App\Providers; use App; use Illuminate\Support\ServiceProvider; class TestFacadesServiceProvider extends ServiceProvider { public function boot() { // } public function register() { App::bind('test',function() { return new \App\Test\TestFacades; }); } }
步骤 6 - 在config/app.php文件中添加服务提供者,如下图所示。
config/app.php
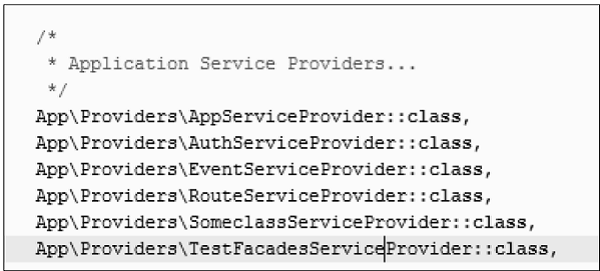
步骤 7 - 在config/app.php文件中添加别名,如下图所示。
config/app.php
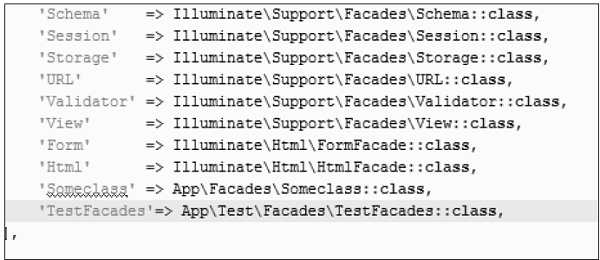
步骤 8 - 在app/Http/routes.php文件中添加以下代码。
app/Http/routes.php
Route::get('/facadeex', function() { return TestFacades::testingFacades(); });
步骤 9 - 访问以下 URL 来测试门面。
https://127.0.0.1:8000/facadeex
步骤 10 - 访问 URL 后,您将收到以下输出:
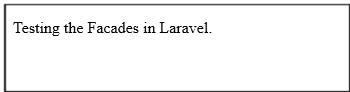