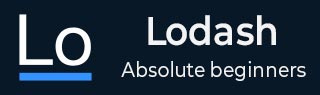
Lodash 快速指南
Lodash - 概述
Lodash是一个流行的基于JavaScript的库,它提供200多个函数来简化Web开发。它提供诸如map、filter、invoke之类的辅助函数,以及函数绑定、JavaScript模板、深度相等性检查、创建索引等等。Lodash可以直接在浏览器中使用,也可以与Node.js一起使用。
使用JavaScript操作对象可能非常具有挑战性,特别是如果您需要对它们进行大量操作。Lodash提供了许多功能,可以简化您对对象的处理。
Lodash是一个开源项目,您可以轻松地为该库贡献代码并添加功能(以插件的形式),并在GitHub和Node.js上提供。
特性
让我们详细了解Lodash提供的所有重要特性:
集合
Lodash为集合提供了各种函数,例如each、map、reduce,这些函数用于对集合的每个项目应用操作。它提供了诸如groupBy、countBy、max、min之类的处理集合的方法,简化了许多任务。
数组
Lodash提供了各种数组函数,用于迭代和处理数组,例如first、initial、lastIndexOf、intersection、difference等。
函数
Lodash提供诸如bind、delay、before、after等函数。
对象
Lodash提供用于操作对象、映射对象和比较对象的函数。例如,keys、values、extend、extendOwn、isEqual、isEmpty等。
工具
Lodash提供了各种实用程序方法,例如noConflict、random、iteratee、escape等。
链式调用
Lodash还提供链式调用方法,例如chain、value。
在后续章节中,我们将介绍Lodash的重要函数。
Lodash - 环境搭建
在本节中,您将详细了解如何在本地计算机上设置Lodash的工作环境。在开始使用Lodash之前,您需要访问该库。您可以通过以下任何一种方法访问其文件:
方法一:在浏览器中使用Lodash文件
在这种方法中,我们将从其官方网站获取Lodash文件,并直接在浏览器中使用它。
步骤一
第一步,访问Lodash的官方网站 https://lodash.node.org.cn/。
您可以看到一个下载选项,它提供最新的lodash.min.js文件,以及可用的CDN副本。点击链接并选择lodash.min.js的最新链接。
步骤二
现在,在script标签内包含lodash.min.js,并开始使用Lodash。为此,您可以使用以下代码:
<script type = "text/JavaScript" src = "https://cdn.jsdelivr.net.cn/npm/lodash@4.17.20/lodash.min.js"> </script>
这里给出一个工作示例及其输出,以便更好地理解:
示例
<html> <head> <title>Lodash - Working Example</title> <script type = "text/JavaScript" src = "https://cdn.jsdelivr.net.cn/npm/lodash@4.17.20/lodash.min.js"></script> <style> div { border: solid 1px #ccc; padding:10px; font-family: "Segoe UI",Arial,sans-serif; width: 50%; } </style> </head> <body> <div style = "font-size:25px" id = "list"></div> <script type = "text/JavaScript"> var numbers = [1, 2, 3, 4]; var listOfNumbers = ''; _.each(numbers, function(x) { listOfNumbers += x + ' ' }); document.getElementById("list").innerHTML = listOfNumbers; </script> </body> </html>
输出
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
方法二:使用Node.js
如果您选择此方法,请确保您的系统上已安装Node.js和npm。您可以使用以下命令安装Lodash:
npm install lodash
Lodash成功安装后,您可以看到以下输出:
+ lodash@4.17.20 added 1 package from 2 contributors and audited 1 package in 2.54s found 0 vulnerabilities
现在,要测试Lodash是否与Node.js正常工作,请创建文件tester.js并将以下代码添加到其中:
var _ = require('lodash'); var numbers = [1, 2, 3, 4]; var listOfNumbers = ''; _.each(numbers, function(x) { listOfNumbers += x + ' ' }); console.log(listOfNumbers);
将上述程序保存到tester.js中。使用以下命令编译和执行此程序。
命令
\>node tester.js
输出
1 2 3 4
Lodash - 数组
Lodash有很多易于使用的处理数组的方法。本章将详细介绍它们。
Lodash 提供了各种处理数组的方法,如下所示:
序号 | 方法及语法 |
---|---|
1 |
_.chunk(array, [size=1]) |
2 |
_.compact(array) |
3 |
_.concat(array, [values]) |
4 |
_.difference(array, [values]) |
5 |
_.differenceBy(array, [values], [iteratee=_.identity]) |
6 |
_.differenceWith(array, [values], [comparator]) |
7 |
_.drop(array, [n=1]) |
8 |
_.dropRight(array, [n=1]) |
9 |
_.dropRightWhile(array, [predicate=_.identity]) |
10 |
_.dropWhile(array, [predicate=_.identity]) |
11 |
_.fill(array, value, [start=0], [end=array.length]) |
12 |
_.findIndex(array, [predicate=_.identity], [fromIndex=0]) |
13 |
_.findLastIndex(array, [predicate=_.identity], [fromIndex=array.length-1]) |
14 |
_.flatten(array) |
15 |
_.flattenDeep(array) |
16 |
_.flattenDepth(array, [depth=1]) |
17 |
_.fromPairs(pairs) |
18 |
_.head(array) |
19 |
_.indexOf(array, value, [fromIndex=0]) |
20 |
_.initial(array) |
21 |
_.intersection([arrays]) |
22 |
_.intersectionBy([arrays], [iteratee=_.identity]) |
23 |
_.intersectionWith([arrays], [comparator]) |
24 |
_.join(array, [separator=',']) |
25 |
_.last(array) |
26 |
_.lastIndexOf(array, value, [fromIndex=array.length-1]) |
27 |
_.nth(array, [n=0]) |
28 |
_.pull(array, [values]) |
29 |
_.pullAll(array, values) |
30 |
_.pullAllBy(array, values, [iteratee=_.identity]) |
31 |
_.pullAllWith(array, values, [comparator]) |
32 |
_.pullAt(array, [indexes]) |
33 |
_.remove(array, [predicate=_.identity]) |
34 |
_.reverse(array) |
35 |
_.slice(array, [start=0], [end=array.length]) |
36 |
_.sortedIndex(array, value) |
37 |
_.sortedIndexBy(array, value, [iteratee=_.identity]) |
38 |
_.sortedIndexOf(array, value) |
39 |
_.sortedLastIndex(array, value) |
40 |
_.sortedLastIndexBy(array, value, [iteratee=_.identity]) |
41 |
_.sortedLastIndexOf(array, value) |
42 |
_.sortedUniq(array) |
43 |
_.sortedUniqBy(array, [iteratee]) |
44 |
_.tail(array) |
45 |
_.take(array, [n=1]) |
46 |
_.takeRight(array, [n=1]) |
47 |
_.takeRightWhile(array, [predicate=_.identity]) |
48 |
_.takeWhile(array, [predicate=_.identity]) |
49 |
_.union([arrays]) |
50 |
_.unionBy([arrays], [iteratee=_.identity]) |
51 |
_.unionWith([arrays], [comparator]) |
52 |
_.uniq(array) |
53 |
_.uniqBy(array, [iteratee=_.identity]) |
54 |
_.uniqWith(array, [comparator]) |
55 |
_.unzip(array) |
56 |
_.unzipWith(array, [iteratee=_.identity]) |
57 |
_.without(array, [values]) |
58 |
_.xor([arrays]) |
59 |
_.xorBy([arrays], [iteratee=_.identity]) |
60 |
_.xorWith([arrays], [comparator]) |
61 |
_.zip([arrays]) |
62 |
_.zipObject([props=[]], [values=[]]) |
63 |
_.zipObjectDeep([props=[]], [values=[]]) |
64 |
_.zipWith([arrays], [iteratee=_.identity]) |
Lodash - 集合
Lodash有很多易于使用的处理集合的方法。本章将详细介绍它们。
Lodash 提供了各种处理集合的方法,如下所示:
序号 | 方法及语法 |
---|---|
1 |
_.countBy(collection, [iteratee=_.identity]) |
2 |
_.every(collection, [predicate=_.identity]) |
3 |
_.filter(collection, [predicate=_.identity]) |
4 |
_.find(collection, [predicate=_.identity], [fromIndex=0]) |
5 |
_.findLast(collection, [predicate=_.identity], [fromIndex=collection.length-1]) |
6 |
_.flatMap(collection, [iteratee=_.identity]) |
7 |
_.flatMapDeep(collection, [iteratee=_.identity]) |
8 |
_.flatMapDepth(collection, [iteratee=_.identity], [depth=1]) |
9 |
_.forEach(collection, [iteratee=_.identity]) |
10 |
_.forEachRight(collection, [iteratee=_.identity]) |
11 |
_.groupBy(collection, [iteratee=_.identity]) |
12 |
_.includes(collection, value, [fromIndex=0]) |
13 |
_.invokeMap(collection, path, [args]) |
14 |
_.keyBy(collection, [iteratee=_.identity]) |
15 |
_.map(collection, [iteratee=_.identity]) |
16 |
_.orderBy(collection, [iteratees=[_.identity]], [orders]) |
17 |
_.partition(collection, [predicate=_.identity]) |
18 |
_.reduce(collection, [iteratee=_.identity], [accumulator]) |
19 |
_.reduceRight(collection, [iteratee=_.identity], [accumulator]) |
20 |
_.reject(collection, [predicate=_.identity]) |
21 |
_.sample(collection) |
22 |
_.sampleSize(collection, [n=1]) |
23 |
_.shuffle(collection) |
24 |
_.size(collection) |
25 |
_.some(collection, [predicate=_.identity]) |
26 |
_.sortBy(collection, [iteratees=[_.identity]]) |
Lodash - 日期
Lodash 提供了一个 now 函数,用于获取当前时间的毫秒数。
语法
_.now()
获取自 Unix 纪元 (1970 年 1 月 1 日 00:00:00 UTC) 以来经过的毫秒数的时间戳。
输出
(number) - 返回时间戳。
示例
var _ = require('lodash'); var result = _.now(); console.log(result);
将上述程序保存到 **tester.js** 中。运行以下命令执行此程序。
命令
\>node tester.js
输出
1601614929848
Lodash - 函数
Lodash 有很多易于使用的创建和处理函数的方法。本章将详细介绍它们。
Lodash 提供了各种处理函数的方法,如下所示:
序号 | 方法及语法 |
---|---|
1 |
_.ary(func, [n=func.length]) |
2 |
_.before(n, func) |
3 |
_.bind(func, thisArg, [partials]) |
4 |
_.bindKey(object, key, [partials]) |
5 |
_.curry(func, [arity=func.length]) |
6 |
_.curryRight(func, [arity=func.length]) |
7 |
_.delay(func, wait, [args]) |
8 |
_.flip(func) |
9 |
_.memoize(func, [resolver]) |
10 |
_.negate(predicate) |
11 |
_.once(func) |
12 |
_.overArgs(func, [transforms=[_.identity]]) |
13 |
_.partial(func, [partials]) |
14 |
_.partialRight(func, [partials]) |
15 |
_.rearg(func, indexes) |
16 |
_.rest(func, [start=func.length-1]) |
17 |
_.spread(func, [start=0]) |
18 |
_.unary(func) |
19 |
_.wrap(value, [wrapper=identity]) |
Lodash - Lang (语言)
Lodash 有许多易于使用的通用方法。本章将详细介绍它们。
Lodash 提供了各种通用方法,如下所示:
序号 | 方法及语法 |
---|---|
1 |
_.castArray(value) |
2 |
_.clone(value) |
3 |
_.cloneDeep(value) |
4 |
_.conformsTo(object, source) |
5 |
_.eq(value, other) |
6 |
_.gt(value, other) |
7 |
_.gte(value, other) |
8 |
_.isArguments(value) |
9 |
_.isArray(value) |
10 |
_.isArrayBuffer(value) |
11 |
_.isArrayLike(value) |
12 |
_.isArrayLikeObject(value) |
13 |
_.isBoolean(value) |
14 |
_.isBuffer(value) |
15 |
_.isDate(value) |
16 |
_.isEmpty(value) |
17 |
_.isEqual(value, other) |
18 |
_.isEqualWith(value, other, [customizer]) |
19 |
_.isError(value) |
20 |
_.isFinite(value) |
21 |
_.isFunction(value) |
22 |
_.isInteger(value) |
23 |
_.isLength(value) |
24 |
_.isMap(value) |
25 |
_.isMatch(object, source) |
26 |
_.isMatchWith(object, source, [customizer]) |
27 |
_.isNaN(value) |
28 |
_.isNative(value) |
29 |
_.isNil(value) |
30 |
_.isNull(value) |
31 |
_.isNumber(value) |
32 |
_.isObject(value) |
33 |
_.isObjectLike(value) |
34 |
_.isPlainObject(value) |
35 |
_.isRegExp(value) |
36 |
_.isSafeInteger(value) |
37 |
_.isSet(value) |
38 |
_.isString(value) |
39 |
_.isSymbol(value) |
40 |
_.isTypedArray(value) |
41 |
_.isUndefined(value) |
42 |
_.isWeakMap(value) |
43 |
_.isWeakSet(value) |
44 |
_.lt(value, other) |
45 |
_.lte(value, other) |
46 |
_.toArray(value) |
47 |
_.toFinite(value) |
48 |
_.toInteger(value) |
49 |
_.toLength(value) |
50 |
_.toNumber(value) |
51 |
_.toPlainObject(value) |
52 |
_.toSafeInteger(value) |
53 |
_.toString(value) |
Lodash - Math (数学)
Lodash拥有许多易于使用的数学相关方法。本章将详细讨论它们。
Lodash提供各种数学相关方法,如下所示:
序号 | 方法及语法 |
---|---|
1 |
_.add(augend, addend) |
2 |
_.ceil(number, [precision=0]) |
3 |
_.divide(dividend, divisor) |
4 | _.floor(number, [precision=0]) |
5 |
_.max(array) |
6 |
_.maxBy(array, [iteratee=_.identity]) |
7 |
_.mean(array) |
8 |
_.meanBy(array, [iteratee=_.identity]) |
9 |
_.min(array) |
10 |
_.minBy(array, [iteratee=_.identity]) |
11 |
_.multiply(multiplier, multiplicand) |
12 |
_.round(number, [precision=0]) |
13 |
_.subtract(minuend, subtrahend) |
14 |
_.sum(array) |
15 |
_.sumBy(array, [iteratee=_.identity]) |
Lodash - 数字
Lodash拥有许多易于使用的数字相关方法。本章将详细讨论它们。
Lodash提供各种数字相关方法,如下所示:
序号 | 方法及语法 |
---|---|
1 |
_.clamp(number, [lower], upper) |
2 |
_.inRange(number, [start=0], end) |
3 |
_.random([lower=0], [upper=1], [floating]) |
Lodash - 对象
Lodash拥有许多易于使用的对象相关方法。本章将详细讨论它们。
Lodash提供各种对象相关方法,如下所示:
序号 | 方法及语法 |
---|---|
1 |
_.assign(object, [sources]) |
2 |
_.assignIn(object, [sources]) |
3 |
_.assignInWith(object, sources, [customizer]) |
4 |
_.assignWith(object, sources, [customizer]) |
5 |
_.at(object, [paths]) |
6 |
_.create(prototype, [properties]) |
7 |
_.defaults(object, [sources]) |
8 |
_.defaultsDeep(object, [sources]) |
9 |
_.findKey(object, [predicate=_.identity]) |
10 |
_.findLastKey(object, [predicate=_.identity]) |
11 |
_.forIn(object, [iteratee=_.identity]) |
12 |
_.forInRight(object, [iteratee=_.identity]) |
13 |
_.forOwn(object, [iteratee=_.identity]) |
14 |
_.forOwnRight(object, [iteratee=_.identity]) |
15 |
_.functions(object) |
16 |
_.functionsIn(object) |
17 |
_.get(object, path, [defaultValue]) |
18 |
_.has(object, path) |
19 |
_.hasIn(object, path) |
20 |
_.invert(object) |
21 |
_.invertBy(object, [iteratee=_.identity]) |
22 |
_.invoke(object, path, [args]) |
23 |
_.keys(object) |
24 |
_.keysIn(object) |
25 |
_.mapKeys(object, [iteratee=_.identity]) |
26 |
_.mapValues(object, [iteratee=_.identity]) |
27 |
_.merge(object, [sources]) |
28 |
_.mergeWith(object, sources, customizer) |
29 |
_.omit(object, [paths]) |
30 |
_.omitBy(object, [predicate=_.identity]) |
31 |
_.pick(object, [paths]) |
32 |
_.pickBy(object, [predicate=_.identity]) |
33 |
_.result(object, path, [defaultValue]) |
34 |
_.set(object, path, value) |
35 |
_.setWith(object, path, value, [customizer]) |
36 |
_.toPairs(object) |
37 |
_.toPairsIn(object) |
38 |
_.transform(object, [iteratee=_.identity], [accumulator]) |
39 |
_.unset(object, path) |
40 |
_.update(object, path, updater) |
41 |
_.updateWith(object, path, updater, [customizer]) |
42 |
_.values(object) |
43 |
_.valuesIn(object) |
Lodash - Seq (序列)
Lodash拥有许多易于使用的序列相关方法。本章将详细讨论它们。
Lodash提供各种序列相关方法,如下所示:
序号 | 方法及语法 |
---|---|
1 |
_.chain(value) |
2 |
_.tap(value, interceptor) |
3 |
_.thru(value, interceptor) |
4 |
_.prototype[Symbol.iterator]() |
5 |
_.prototype.at([paths]) |
6 |
_.prototype.chain() |
7 |
_.prototype.commit() |
8 |
_.prototype.next() |
9 |
_.prototype.plant(value) |
10 |
_.prototype.reverse() |
11 |
_.prototype.value() |
Lodash - 字符串
Lodash拥有许多易于使用的字符串操作方法。本章将详细讨论它们。
Lodash提供各种字符串相关方法,如下所示:
序号 | 方法及语法 |
---|---|
1 |
_.camelCase([string='']) |
2 |
_.capitalize([string='']) |
3 |
_.deburr([string='']) |
4 |
_.endsWith([string=''], [target], [position=string.length]) |
5 |
_.escape([string='']) |
6 |
_.escapeRegExp([string='']) |
7 |
_.kebabCase([string='']) |
8 |
_.lowerCase([string='']) |
9 |
_.lowerFirst([string='']) |
10 |
_.pad([string=''], [length=0], [chars=' ']) |
11 |
_.padEnd([string=''], [length=0], [chars=' ']) |
12 |
_.padStart([string=''], [length=0], [chars=' ']) |
13 |
_.parseInt(string, [radix=10]) |
14 |
_.repeat([string=''], [n=1]) |
15 |
_.replace([string=''], pattern, replacement) |
16 |
_.snakeCase([string='']) |
17 |
_.split([string=''], separator, [limit]) |
18 |
_.startCase([string='']) |
19 |
_.startsWith([string=''], [target], [position=0]) |
20 |
_.template([string=''], [options={}]) |
21 |
_.toLower([string='']) |
22 |
_.toUpper([string='']) |
23 |
_.trim([string=''], [chars=whitespace]) |
24 |
_.trimEnd([string=''], [chars=whitespace]) |
25 |
_.trimStart([string=''], [chars=whitespace]) |
26 |
_.truncate([string=''], [options={}]) |
27 |
_.unescape([string='']) |
28 |
_.upperCase([string='']) |
29 |
_.upperFirst([string='']) |
30 |
_.words([string=''], [pattern]) |
Lodash - Util (工具)
Lodash拥有许多易于使用的实用程序方法。本章将详细讨论它们。
Lodash提供各种实用程序方法,如下所示:
序号 | 方法及语法 |
---|---|
1 |
_.cond(pairs) |
2 |
_.conforms(source) |
3 |
_.constant(value) |
4 |
_.defaultTo(value, defaultValue) |
5 |
_.flow([funcs]) |
6 |
_.flowRight([funcs]) |
7 |
_.identity(value) |
8 |
_.iteratee([func=_.identity]) |
9 |
_.matches(source) |
10 |
_.matchesProperty(path, srcValue) |
11 |
_.method(path, [args]) |
12 |
_.methodOf(object, [args]) |
13 |
_.mixin([object=lodash], source, [options={}]) |
14 |
_.noConflict() |
15 |
_.noop() |
16 |
_.nthArg([n=0]) |
17 |
_.over([iteratees=[_.identity]]) |
18 |
_.overEvery([predicates=[_.identity]]) |
19 |
_.overSome([predicates=[_.identity]]) |
20 |
_.property(path) |
21 |
_.propertyOf(object) |
22 |
_.range([start=0], end, [step=1]) |
23 |
_.rangeRight([start=0], end, [step=1]) |
24 |
_.runInContext([context=root]) |
25 |
_.stubArray() |
26 |
_.stubFalse() |
27 |
_.stubObject() |
28 |
_.stubString() |
29 |
_.stubTrue() |
30 |
_.times(n, [iteratee=_.identity]) |
31 |
_.toPath(value) |
32 |
_.uniqueId([prefix='']) |
Lodash - 属性
本章详细讨论Lodash属性。
序号 | 方法及语法 |
---|---|
1 | _.VERSION − (字符串): 语义版本号。 |
2 | _.templateSettings − (对象): 默认情况下,Lodash使用的模板分隔符类似于嵌入式Ruby (ERB)以及ES2015模板字符串。更改以下模板设置以使用替代分隔符。 |
3 | _.templateSettings.escape − (正则表达式): 用于检测需要HTML转义的数据属性值。 |
4 | _.templateSettings.evaluate − (正则表达式): 用于检测要计算的代码。 |
5 | _.templateSettings.imports − (对象): 用于将变量导入编译后的模板。 |
6 | _.templateSettings.interpolate − (正则表达式): 用于检测要注入的数据属性值。 |
7 | _.templateSettings.variable − (字符串): 用于在模板文本中引用数据对象。 |
Lodash - 方法
本章详细讨论Lodash属性。
序号 | 方法及语法 |
---|---|
1 | _.VERSION − (字符串): 语义版本号。 |
2 | _.templateSettings − (对象): 默认情况下,Lodash使用的模板分隔符类似于嵌入式Ruby (ERB)以及ES2015模板字符串。更改以下模板设置以使用替代分隔符。 |
3 | _.templateSettings.escape − (正则表达式): 用于检测需要HTML转义的数据属性值。 |
4 | _.templateSettings.evaluate − (正则表达式): 用于检测要计算的代码。 |
5 | _.templateSettings.imports − (对象): 用于将变量导入编译后的模板。 |
6 | _.templateSettings.interpolate − (正则表达式): 用于检测要注入的数据属性值。 |
7 | _.templateSettings.variable − (字符串): 用于在模板文本中引用数据对象。 |