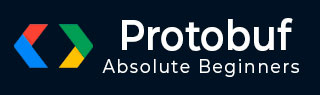
- Protocol Buffers 教程
- Protocol Buffers - 首页
- Protocol Buffers - 简介
- Protocol Buffers - 基本应用
- Protocol Buffers - 结构
- Protocol Buffers - 消息
- Protocol Buffers - 字符串
- Protocol Buffers - 数字
- Protocol Buffers - 布尔值
- Protocol Buffers - 枚举
- Protocol Buffers - 重复字段
- Protocol Buffers - 映射
- Protocol Buffers - 嵌套类
- Protocol Buffers - 可选字段与默认值
- Protocol Buffers - 语言无关性
- Protocol Buffers - 复合数据类型
- Protocol Buffers - 命令行使用
- Protocol Buffers - 更新定义规则
- Protocol Buffers - 与 Kafka 集成
- Protocol Buffers - 其他语言支持
- Protocol Buffers 有用资源
- Protocol Buffers - 快速指南
- Protocol Buffers - 有用资源
- Protocol Buffers - 讨论
Protocol Buffers - 枚举
概述
enum 数据类型是 Protobuf 的复合数据类型之一。它在使用的语言(例如 Java 等)中转换为枚举。
继续我们从 Protocol Buffers - 字符串章节的剧院示例,以下是我们需要使用的语法,以指示 Protobuf 我们将创建一个枚举:
theater.proto
syntax = "proto3"; package theater; option java_package = "com.tutorialspoint.theater"; message Theater { enum PAYMENT_SYSTEM{ CASH = 0; CREDIT_CARD = 1; DEBIT_CARD = 2; APP = 3; } PAYMENT_SYSTEM payment = 7; }
现在我们的消息类包含一个枚举属性。它还有一个位置,这是 Protobuf 在序列化和反序列化时使用的。每个成员属性都需要分配一个唯一的数字。
我们定义了枚举,并在下面将其用作数据类型以及“payment”属性。请注意,尽管我们在消息类内部定义了枚举,但它也可以位于消息类外部。
从 Proto 文件创建 Java 类
要使用 Protobuf,我们现在必须使用protoc二进制文件从这个“.proto”文件创建所需的类。让我们看看如何操作:
protoc --java_out=. theater.proto
这将在当前目录的com > tutorialspoint > theater文件夹中创建一个TheaterOuterClass.java类。我们在应用程序中使用此类,类似于Protocol Buffers - 基本应用章节中所做的。
使用从 Proto 文件创建的 Java 类
TheaterWriter.java
package com.tutorialspoint.theater; import java.io.FileOutputStream; import java.io.IOException; import com.tutorialspoint.theater.TheaterOuterClass.Theater; import com.tutorialspoint.theater.TheaterOuterClass.Theater.PAYMENT_SYSTEM; public class TheaterWriter{ public static void main(String[] args) throws IOException { Theater theater = Theater.newBuilder() .setPayment(PAYMENT_SYSTEM.CREDIT_CARD) .build(); String filename = "theater_protobuf_output"; System.out.println("Saving theater information to file: " + filename); try(FileOutputStream output = new FileOutputStream(filename)){ theater.writeTo(output); } System.out.println("Saved theater information with following data to disk: \n" + theater); } }
接下来,我们将有一个读取器来读取剧院信息:
TheaterReader.java
package com.tutorialspoint.theater; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import com.tutorialspoint.greeting.Greeting.Greet; import com.tutorialspoint.theater.TheaterOuterClass.Theater; import com.tutorialspoint.theater.TheaterOuterClass.Theater.Builder; public class TheaterReader{ public static void main(String[] args) throws IOException { Builder theaterBuilder = Theater.newBuilder(); String filename = "theater_protobuf_output"; System.out.println("Reading from file " + filename); try(FileInputStream input = new FileInputStream(filename)) { Theater theater = theaterBuilder.mergeFrom(input).build(); System.out.println(theater.getPayment()); System.out.println(theater); } } }
编译项目
现在我们已经设置了读取器和写入器,让我们编译项目。
mvn clean install
序列化 Java 对象
现在,编译后,让我们首先执行写入器:
> java -cp .\target\protobuf-tutorial-1.0.jar com.tutorialspoint.theater.TheaterWriter Saving theater information to file: theater_protobuf_output Saved theater information with following data to disk: payment: CREDIT_CARD
反序列化已序列化的对象
现在,让我们执行读取器以从同一文件读取:
java -cp .\target\protobuf-tutorial-1.0.jar com.tutorialspoint.theater.TheaterReader Reading from file theater_protobuf_output CREDIT_CARD payment: CREDIT_CARD
因此,正如我们所看到的,我们能够通过将二进制数据反序列化为Theater对象来读取序列化的枚举。在下一章Protocol Buffers - 重复字段中,我们将了解重复字段,这是一种复合类型。
广告