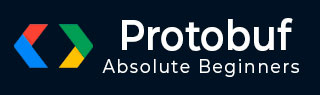
- Protocol Buffers 教程
- Protocol Buffers - 首页
- Protocol Buffers - 简介
- Protocol Buffers - 基本应用
- Protocol Buffers - 结构
- Protocol Buffers - 消息
- Protocol Buffers - 字符串
- Protocol Buffers - 数字
- Protocol Buffers - 布尔值
- Protocol Buffers - 枚举
- Protocol Buffers - 重复字段
- Protocol Buffers - 映射
- Protocol Buffers - 嵌套类
- Protocol Buffers - 可选字段和默认值
- Protocol Buffers - 语言独立性
- Protocol Buffers - 复合数据类型
- Protocol Buffers - 命令行使用
- Protocol Buffers - 更新定义规则
- Protocol Buffers - 与 Kafka 集成
- Protocol Buffers - 在其他语言中
- Protocol Buffers 有用资源
- Protocol Buffers - 快速指南
- Protocol Buffers - 有用资源
- Protocol Buffers - 讨论
Protocol Buffers - 语言独立性
概述
到目前为止,我们一直在使用 Java 来序列化和反序列化电影院数据。但是,Google Protocol Buffers 提供的关键功能之一是“语言独立性”。在本章中,我们将了解如何使用 Java 进行序列化,并使用 Python 进行反序列化。
继续我们从Protocol Buffers - 字符串章节开始的theater示例,以下是我们需要使用的语法,以指示 Protobuf 我们将创建不同的数据类型:
theater.proto
syntax = "proto3"; package theater; option java_package = "com.tutorialspoint.theater"; message Theater { string name = 1; string address = 2; int32 total_capcity = 3; int64 mobile = 4; float base_ticket_price = 5; bool drive_in = 6; enum PAYMENT_SYSTEM { CASH = 0; CREDIT_CARD = 1; DEBIT_CARD = 2; APP = 3; } PAYMENT_SYSTEM payment = 7; repeated string snacks = 8; map<string, int32> movieTicketPrice = 9; TheaterOwner owner = 10; } message TheaterOwner{ string name = 1; string address = 2; }
使用 Java 进行序列化
要使用 Protobuf,我们现在必须使用protoc二进制文件从这个“.proto”文件创建所需的类。让我们看看如何做到这一点:
protoc --java_out=. theater.proto
这将在当前目录的com > tutorialspoint > theater文件夹中创建一个TheaterOuterClass.java类。我们在应用程序中使用此类,就像在Protocol Buffers - 基本应用章节中所做的那样。
使用从 Proto 文件创建的 Java 类
首先,我们将创建一个写入器来写入剧院信息:
TheaterWriter.java
package com.tutorialspoint.theater; import java.io.FileOutputStream; import java.io.IOException; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; import com.tutorialspoint.theater.TheaterOuterClass.Theater; import com.tutorialspoint.theater.TheaterOuterClass.TheaterOwner; import com.tutorialspoint.theater.TheaterOuterClass.Theater.PAYMENT_SYSTEM; public class TheaterWriter { public static void main(String[] args) throws IOException { TheaterOwner owner = TheaterOwner.newBuilder() .setName("Anthony Gonsalves") .setAddress("513, St Paul Street, West Coast, California") .build(); List<String> snacks = new ArrayList<>(); snacks.add("Popcorn"); snacks.add("Coke"); snacks.add("Chips"); snacks.add("Soda"); Map<String, Integer> ticketPrice = new HashMap<>(); ticketPrice.put("Avengers Endgame", 700); ticketPrice.put("Captain America", 200); ticketPrice.put("Wonder Woman 1984", 400); Theater theater = Theater.newBuilder() .setName("Silver Screener") .setAddress("212, Maple Street, LA, California") .setDriveIn(true) .setTotalCapcity(320) .setMobile(98234567189L) .setBaseTicketPrice(22.45f) .setPayment(PAYMENT_SYSTEM.CREDIT_CARD) .putAllMovieTicketPrice(ticketPrice) .addAllSnacks(snacks) .setOwner(owner) .build(); String filename = "E:/theater_protobuf_output"; System.out.println("Saving theater information to file: " + filename); try(FileOutputStream output = new FileOutputStream(filename)){ theater.writeTo(output); } System.out.println("Saved theater information with following data to disk: \n" + theater); } }
让我们编译项目。
mvn clean install
序列化 Java 对象
现在,编译后,让我们执行writer:
> java -cp .\target\protobuf-tutorial-1.0.jar com.tutorialspoint.theater.TheaterWriter Saving theater information to file: E:/theater_protobuf_output Saved theater information with following data to disk: name: "Silver Screener" address: "212, Maple Street, LA, California" total_capcity: 320 mobile: 98234567189 base_ticket_price: 22.45 drive_in: true payment: CREDIT_CARD snacks: "Popcorn" snacks: "Coke" snacks: "Chips" snacks: "Soda" movieTicketPrice { key: "Avengers Endgame" value: 700 } movieTicketPrice { key: "Captain America" value: 200 } movieTicketPrice { key: "Wonder Woman 1984" value: 400 } owner { name: "Anthony Gonsalves" address: "513, St Paul Street, West Coast, California" }
使用 Python 反序列化序列化对象
从 proto 文件生成 Python 类
让我们为 Theater 类生成 Python 代码:
protoc --python_out=. theater.proto
执行此命令后,您会在当前目录中注意到一个自动生成的类theater_pb2.py。此类将帮助我们反序列化Theater对象。
使用生成的 Python 类
现在,让我们编写数据读取器,它将使用 Java 读取包含序列化对象的文件:
theaterReader.py
import theater_pb2 filename = "E:/theater_protobuf_output"; print("Reading from file: " + filename) theater = theater_pb2.Theater() f = open(filename, "rb") theater.ParseFromString(f.read()) f.close() print("Read theater from disk: \n" + str(theater))
然后,让我们执行reader。
python theaterReader.py Reading from file: E:/greeting_protobuf_output Read theater from disk: name: "Silver Screener" address: "212, Maple Street, LA, California" total_capcity: 320 mobile: 98234567189 base_ticket_price: 22.45 drive_in: true payment: CREDIT_CARD snacks: "Popcorn" snacks: "Coke" snacks: "Chips" snacks: "Soda" movieTicketPrice { key: "Wonder Woman 1984" value: 400 } movieTicketPrice { key: "Captain America" value: 200 } movieTicketPrice { key: "Avengers Endgame" value: 700 } owner { name: "Anthony Gonsalves" address: "513, St Paul Street, West Coast, California" }
因此,正如我们所看到的,Java 客户端写入的所有值都被正确地反序列化并由我们的 Python 客户端读取,这有效地意味着 Protobuf 是语言独立的。
广告