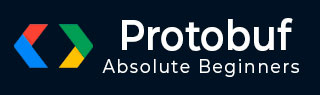
- Protocol Buffers 教程
- Protocol Buffers - 首页
- Protocol Buffers - 简介
- Protocol Buffers - 基本应用
- Protocol Buffers - 结构
- Protocol Buffers - 消息
- Protocol Buffers - 字符串
- Protocol Buffers - 数字
- Protocol Buffers - 布尔值
- Protocol Buffers - 枚举
- Protocol Buffers - 重复字段
- Protocol Buffers - map
- Protocol Buffers - 嵌套类
- Protocol Buffers - 可选字段和默认值
- Protocol Buffers - 语言无关性
- Protocol Buffers - 复合数据类型
- Protocol Buffers - 命令行使用
- Protocol Buffers - 更新定义规则
- Protocol Buffers - 与 Kafka 集成
- Protocol Buffers - 其他语言支持
- Protocol Buffers 有用资源
- Protocol Buffers - 快速指南
- Protocol Buffers - 有用资源
- Protocol Buffers - 讨论
Protocol Buffers - map
概述
map 数据类型是 Protobuf 的复合数据类型之一。它在 Java 中转换为 java.util.Map 接口。
继续我们从 Protocol Buffers - 字符串章节的 theater 示例,以下是我们需要使用的语法,以指示 Protobuf 我们将创建一个 repeated(重复)字段:
theater.proto
syntax = "proto3"; package theater; option java_package = "com.tutorialspoint.theater"; message Theater { map<string, int32> movieTicketPrice = 9; }
现在我们的 message 类包含一个电影及其票价的 map。请注意,虽然我们有 “string -> int” map,但我们也可以使用数字、布尔值和自定义数据类型。但是,请注意我们不能嵌套 map。它还有一个 position 属性,这是 Protobuf 在序列化和反序列化时使用的。每个成员属性都需要分配一个唯一的数字。
从 Proto 文件创建 Java 类
要使用 Protobuf,我们现在必须使用 protoc 二进制文件从此“.proto”文件创建所需的类。让我们看看如何做到这一点:
protoc --java_out=. theater.proto
这将在当前目录的 com > tutorialspoint > theater 文件夹中创建一个 TheaterOuterClass.java 类。我们在应用程序中使用此类,类似于 Protocol Buffers - 基本应用章节中所做的。
使用从 Proto 文件创建的 Java 类
TheaterWriter.java
package com.tutorialspoint.theater; import java.io.FileOutputStream; import java.io.IOException; import java.util.HashMap; import java.util.Map; import com.tutorialspoint.theater.TheaterOuterClass.Theater; public class TheaterWriter{ public static void main(String[] args) throws IOException { Map<String, Integer> ticketPrice = new HashMap<>(); ticketPrice.put("Avengers Endgame", 700); ticketPrice.put("Captain America", 200); ticketPrice.put("Wonder Woman 1984", 400); Theater theater = Theater.newBuilder() .putAllMovieTicketPrice(ticketPrice) .build(); String filename = "theater_protobuf_output"; System.out.println("Saving theater information to file: " + filename); try(FileOutputStream output = new FileOutputStream(filename)){ theater.writeTo(output); } System.out.println("Saved theater information with following data to disk: \n" + theater); } }
接下来,我们将有一个 reader 来读取 theater 信息:
TheaterReader.java
package com.tutorialspoint.theater; import java.io.FileInputStream; import java.io.IOException; import com.tutorialspoint.theater.TheaterOuterClass.Theater; import com.tutorialspoint.theater.TheaterOuterClass.Theater.Builder; public class TheaterReader{ public static void main(String[] args) throws IOException { Builder theaterBuilder = Theater.newBuilder(); String filename = "theater_protobuf_output"; System.out.println("Reading from file " + filename); try(FileInputStream input = new FileInputStream(filename)) { Theater theater = theaterBuilder.mergeFrom(input).build(); System.out.println(theater); } } }
编译项目
现在我们已经设置了 reader 和 writer,让我们编译项目。
mvn clean install
序列化 Java 对象
现在,编译后,让我们首先执行 writer:
> java -cp .\target\protobuf-tutorial-1.0.jar com.tutorialspoint.theater.TheaterWriter Saving theater information to file: theater_protobuf_output Saved theater information with following data to disk: movieTicketPrice { key: "Avengers Endgame" value: 700 } movieTicketPrice { key: "Captain America" value: 200 } movieTicketPrice { key: "Wonder Woman 1984" value: 400 }
反序列化已序列化的对象
现在,让我们执行 reader 从同一文件中读取:
java -cp .\target\protobuf-tutorial-1.0.jar com.tutorialspoint.theater.TheaterReader Reading from file theater_protobuf_output movieTicketPrice { key: "Avengers Endgame" value: 700 } movieTicketPrice { key: "Captain America" value: 200 } movieTicketPrice { key: "Wonder Woman 1984" value: 400 }
因此,正如我们所看到的,我们能够通过将二进制数据反序列化为 Theater 对象来读取已序列化的 map。在下一章 Protocol Buffers - 嵌套类中,我们将了解嵌套类。
广告