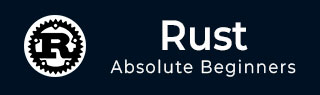
- Rust 教程
- Rust - 首页
- Rust - 简介
- Rust - 环境搭建
- Rust - HelloWorld 示例
- Rust - 数据类型
- Rust - 变量
- Rust - 常量
- Rust - 字符串
- Rust - 运算符
- Rust - 决策
- Rust - 循环
- Rust - 函数
- Rust - 元组
- Rust - 数组
- Rust - 所有权
- Rust - 借用
- Rust - 切片
- Rust - 结构体
- Rust - 枚举
- Rust - 模块
- Rust - 集合
- Rust - 错误处理
- Rust - 泛型
- Rust - 输入输出
- Rust - 文件输入/输出
- Rust - 包管理器
- Rust - 迭代器和闭包
- Rust - 智能指针
- Rust - 并发
- Rust 有用资源
- Rust - 快速指南
- Rust - 有用资源
- Rust - 讨论
Rust - 并发
在并发编程中,程序的不同部分独立执行。另一方面,在并行编程中,程序的不同部分同时执行。随着越来越多的计算机利用其多处理器优势,这两种模型都同等重要。
线程
我们可以使用线程来同时运行代码。在当前的操作系统中,已执行程序的代码在一个进程中运行,操作系统同时管理多个进程。在您的程序中,您还可以拥有同时运行的独立部分。运行这些独立部分的功能称为线程。
创建线程
thread::spawn 函数用于创建新线程。spawn 函数接受闭包作为参数。闭包定义了线程应该执行的代码。以下示例从主线程打印一些文本,从新线程打印其他文本。
//import the necessary modules use std::thread; use std::time::Duration; fn main() { //create a new thread thread::spawn(|| { for i in 1..10 { println!("hi number {} from the spawned thread!", i); thread::sleep(Duration::from_millis(1)); } }); //code executed by the main thread for i in 1..5 { println!("hi number {} from the main thread!", i); thread::sleep(Duration::from_millis(1)); } }
输出
hi number 1 from the main thread! hi number 1 from the spawned thread! hi number 2 from the main thread! hi number 2 from the spawned thread! hi number 3 from the main thread! hi number 3 from the spawned thread! hi number 4 from the spawned thread! hi number 4 from the main thread!
主线程打印从 1 到 4 的值。
注意 - 主线程结束后,新线程将停止。该程序的输出每次可能略有不同。
thread::sleep 函数强制线程停止执行一小段时间,允许其他线程运行。线程可能会轮流运行,但这并非保证——这取决于操作系统如何调度线程。在这个运行中,主线程首先打印,即使来自派生线程的打印语句在代码中先出现。此外,即使派生线程被编程为打印到 9 的值,它在主线程关闭之前只运行到 5。
连接句柄
派生的线程可能没有机会运行或完全运行。这是因为主线程很快就完成了。函数 spawn<F, T>(f: F) -> JoinHandlelt;T> 返回一个 JoinHandle。JoinHandle 上的 join() 方法等待关联的线程完成。
use std::thread; use std::time::Duration; fn main() { let handle = thread::spawn(|| { for i in 1..10 { println!("hi number {} from the spawned thread!", i); thread::sleep(Duration::from_millis(1)); } }); for i in 1..5 { println!("hi number {} from the main thread!", i); thread::sleep(Duration::from_millis(1)); } handle.join().unwrap(); }
输出
hi number 1 from the main thread! hi number 1 from the spawned thread! hi number 2 from the spawned thread! hi number 2 from the main thread! hi number 3 from the spawned thread! hi number 3 from the main thread! hi number 4 from the main thread! hi number 4 from the spawned thread! hi number 5 from the spawned thread! hi number 6 from the spawned thread! hi number 7 from the spawned thread! hi number 8 from the spawned thread! hi number 9 from the spawned thread!
主线程和派生线程继续切换。
注意 - 由于调用了 join() 方法,主线程等待派生线程完成。
广告