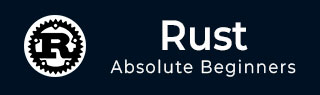
- Rust 教程
- Rust - 首页
- Rust - 简介
- Rust - 环境设置
- Rust - HelloWorld 示例
- Rust - 数据类型
- Rust - 变量
- Rust - 常量
- Rust - 字符串
- Rust - 运算符
- Rust - 决策制定
- Rust - 循环
- Rust - 函数
- Rust - 元组
- Rust - 数组
- Rust - 所有权
- Rust - 借用
- Rust - 切片
- Rust - 结构体
- Rust - 枚举
- Rust - 模块
- Rust - 集合
- Rust - 错误处理
- Rust - 泛型类型
- Rust - 输入输出
- Rust - 文件输入/输出
- Rust - 包管理器
- Rust - 迭代器和闭包
- Rust - 智能指针
- Rust - 并发
- Rust 有用资源
- Rust - 快速指南
- Rust - 有用资源
- Rust - 讨论
Rust - 循环
可能有些情况下,需要重复执行一段代码块。通常,程序指令是顺序执行的:函数中的第一条语句首先执行,然后是第二条,依此类推。
编程语言提供各种控制结构,允许更复杂的执行路径。
循环语句允许我们多次执行一条语句或一组语句。下面是在大多数编程语言中循环语句的一般形式。
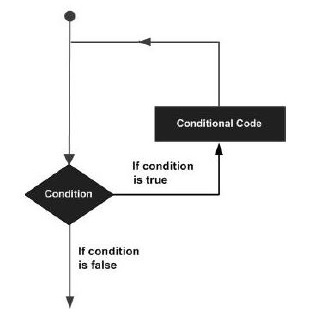
Rust 提供不同类型的循环来处理循环需求:
- while
- loop
- for
确定循环
迭代次数确定/固定的循环称为确定循环。for循环是确定循环的一种实现。
For循环
for循环执行代码块指定的次数。它可以用于迭代一组固定的值,例如数组。for循环的语法如下所示:
语法
for temp_variable in lower_bound..upper_bound { //statements }
for循环示例如下所示:
fn main(){ for x in 1..11{ // 11 is not inclusive if x==5 { continue; } println!("x is {}",x); } }
注意:变量x仅在for块内可访问。
输出
x is 1 x is 2 x is 3 x is 4 x is 6 x is 7 x is 8 x is 9 x is 10
不确定循环
当循环的迭代次数不确定或未知时,使用不确定循环。
不确定循环可以使用:
序号 | 名称及描述 |
---|---|
1 | While while循环在每次指定条件评估为true时执行指令。 |
2 | Loop loop是一个while(true)不确定循环。 |
while循环示例
fn main(){ let mut x = 0; while x < 10{ x+=1; println!("inside loop x value is {}",x); } println!("outside loop x value is {}",x); }
输出如下:
inside loop x value is 1 inside loop x value is 2 inside loop x value is 3 inside loop x value is 4 inside loop x value is 5 inside loop x value is 6 inside loop x value is 7 inside loop x value is 8 inside loop x value is 9 inside loop x value is 10 outside loop x value is 10
loop循环示例
fn main(){ //while true let mut x = 0; loop { x+=1; println!("x={}",x); if x==15 { break; } } }
break语句用于将控制权从一个结构中转移出去。在循环中使用break会导致程序退出循环。
输出
x=1 x=2 x=3 x=4 x=5 x=6 x=7 x=8 x=9 x=10 x=11 x=12 x=13 x=14 x=15
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
Continue语句
continue语句跳过当前迭代中的后续语句,并将控制权返回到循环的开头。与break语句不同,continue不会退出循环。它终止当前迭代并开始后续迭代。
continue语句示例如下。
fn main() { let mut count = 0; for num in 0..21 { if num % 2==0 { continue; } count+=1; } println! (" The count of odd values between 0 and 20 is: {} ",count); //outputs 10 }
上述示例显示0到20之间偶数值的数量。如果数字是偶数,则循环退出当前迭代。这是使用continue语句实现的。
0到20之间奇数值的数量是10。
广告