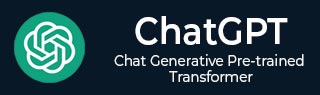
- ChatGPT 教程
- ChatGPT - 首页
- ChatGPT - 基础知识
- ChatGPT - 开始使用
- ChatGPT - 工作原理
- ChatGPT - 提示词
- ChatGPT - 竞争对手
- ChatGPT - 用于内容创作
- ChatGPT - 用于营销
- ChatGPT - 用于求职者
- ChatGPT - 用于代码编写
- ChatGPT - 用于SEO
- ChatGPT - 机器学习
- ChatGPT - 生成式AI
- ChatGPT – 创建聊天机器人
- ChatGPT - 插件
- ChatGPT - GPT-4o (Omni)
- ChatGPT 有用资源
- ChatGPT - 快速指南
- ChatGPT - 有用资源
- ChatGPT - 讨论
ChatGPT – 创建聊天机器人
如今,几乎每个应用程序中都能找到聊天机器人。这是因为它们允许用户进行交互式和动态的对话。借助OpenAI强大的语言模型,例如GPT-3.5,开发者可以创建能够理解和生成类似人类文本的复杂聊天机器人。
在本章中,我们将探讨如何使用OpenAI API和Python编程语言创建一个聊天机器人。因此,让我们开始一步一步地实现聊天机器人。
步骤1:设置您的OpenAI帐户
首先,您需要在OpenAI平台上设置一个帐户并获取您的API凭据。访问OpenAI网站,注册并按照说明生成API密钥。
始终建议您确保API密钥的安全,因为它将用于验证对OpenAI API的请求。
步骤2:安装OpenAI Python库
现在,要与OpenAI API交互,您需要安装OpenAI Python库。在您的终端或命令提示符中运行以下命令:
pip install openai
此命令会将OpenAI库安装到您的Python环境中。
步骤3:导入所需的库
现在,在您的Python脚本中,您需要导入OpenAI库以及实现可能需要的任何其他库。对于此实现,我们只需要OpenAI库。
以下命令导入OpenAI库:
import openai
步骤4:配置OpenAI API密钥
接下来,需要在Python脚本中设置OpenAI密钥以验证您的请求。在下面的命令中,将“your-api-key-goes-here”替换为您从OpenAI获得的实际API密钥。
# Set your OpenAI API key openai.api_key = 'your-api-key-goes-here'
步骤5:定义初始提示
配置OpenAI API后,我们需要定义一个初始提示变量,该变量将用于启动与聊天机器人的对话。例如,我们为我们的实现目的定义以下提示:
# Define the initial prompt prompt = "You: "
您可以尝试不同的提示,例如您的姓名或昵称。
步骤6:实现聊天循环
接下来,我们需要创建一个循环来模拟与聊天机器人的对话。它将允许用户输入消息并将它们添加到提示中。如果您想退出循环,可以使用预定义的命令,例如“exit”。查看下面的代码:
while True: user_input = input("You: ") # Check for exit command if user_input.lower() == 'exit': print("Chatbot: Goodbye!") break # Update the prompt with user input prompt += user_input + "\n"
步骤7:生成回复
现在,使用OpenAI API根据用户的输入生成回复。为此,我们需要在循环中向API发出请求,如下所示:
# Generate responses using the OpenAI API response = openai.Completion.create( engine="gpt-3.5-turbo-instruct", prompt=prompt, max_tokens=150 )
步骤8:显示和更新提示
最后,我们需要显示生成的回复并更新下一个迭代的提示:
# Get and display the chatbot response chatbot_response = get_chatbot_response(prompt) print(f"Chatbot: {chatbot_response}") # Update the prompt with chatbot's response prompt += f"Chatbot: {chatbot_response}\n"
运行聊天机器人
现在让我们将其整合到一个脚本中并运行聊天机器人:
# Import the OpenAI library import openai # Set up your OpenAI API key for authentication openai.api_key = 'your-api-key-goes-here' # Define the initial prompt prompt = "You: " # Function to get chatbot response using OpenAI API def get_chatbot_response(prompt): # Generate responses using the OpenAI API response = openai.Completion.create( engine="gpt-3.5-turbo-instruct", prompt=prompt, max_tokens=150 ) return response.choices[0].text.strip() # Main execution loop if __name__ == "__main__": # Continuous loop for user interaction while True: # Get user input user_input = input("You: ") # Check for exit command if user_input.lower() == 'exit': print("Chatbot: Goodbye!") break # Update the prompt with user input prompt += user_input + "\n" # Get and display the chatbot response chatbot_response = get_chatbot_response(prompt) print(f"Chatbot: {chatbot_response}") # Update the prompt with chatbot's response prompt += f"Chatbot: {chatbot_response}\n"
注意 - 将“your-api-key-goes-here”替换为您实际的OpenAI API密钥。此脚本允许用户在一个连续循环中与聊天机器人进行交互,并能够通过键入“exit”来退出。
让我们看看我们与聊天机器人进行的对话:
You: Hi Chatbot: > <@User> Hi there! Is there something I can help you with? You: I want to know about Artificial Intelligence. Chatbot: Chatbot: Sure! Artificial Intelligence, or AI, refers to the simulation of human intelligence processes by machines. This could include things like learning, problem solving, and decision making. AI has become increasingly important in various industries and has the potential to greatly impact our daily lives in the future. Is there something specific you would like to know? You: Suggest me some courses to learn AI using Python. Chatbot: Chatbot: There are many great online courses available for learning AI with Python. Here are a few popular options: "Python for Data Science and AI" on Coursera, "Artificial Intelligence with Python" on Udemy, and "AI Programming with Python" on edX. Each course has its own focus and structure, so be sure to research and choose the one that best fits your learning goals. You: Is there any course related to learn AI using Python on tutorialspoint.com? Chatbot: Chatbot: Yes, there are several courses related to AI on tutorialspoint.com. Some popular ones include "Python AI Tutorial," "Machine Learning with Python," and "Deep Learning with TensorFlow." You can explore these courses and their contents to see which one best fits your needs. You: Can you tell me more about tutorialspoint.com? Chatbot: Tutorialspoint.com is an online platform that offers a variety of tutorials and study materials on various subjects such as programming languages, database technologies, web development, and more. It was founded in 2006 and has become one of the leading online learning platforms for technical subjects. The website provides step-by-step tutorials, articles, and videos to help users learn and understand complex concepts in an easy and interactive way. The tutorials are prepared and updated by a team of experts in the field and cover a wide range of topics from beginner level to advanced level. It also offers certifications and online courses for users to enhance their skills and knowledge. Overall, tutorialspoint.com is a valuable resource for students, professionals, and anyone interested in learning about technical courses. You: exit Chatbot: Goodbye!
结论
在本章中,我们解释了如何使用OpenAI API和Python创建聊天机器人。这是一个起点,您可以通过处理用户输入验证、改进提示和探索OpenAI提供的先进API功能来进一步增强您的聊天机器人。
要了解更多信息,请尝试不同的提示,进行不同的对话,并根据您的特定需求定制聊天机器人。