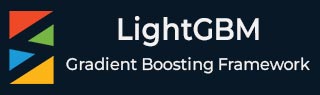
- LightGBM 教程
- LightGBM - 首页
- LightGBM - 概述
- LightGBM - 架构
- LightGBM - 安装
- LightGBM - 核心参数
- LightGBM - Boosting算法
- LightGBM - 树生长策略
- LightGBM - 数据集结构
- LightGBM - 二元分类
- LightGBM - 回归
- LightGBM - 排序
- LightGBM - Python实现
- LightGBM - 参数调优
- LightGBM - 绘图功能
- LightGBM - 早停训练
- LightGBM - 特征交互约束
- LightGBM 与其他Boosting算法的比较
- LightGBM 有用资源
- LightGBM - 有用资源
- LightGBM - 讨论
LightGBM - Python实现
本章将介绍使用Python开发LightGBM模型的步骤。我们将使用Scikit-learn的load_breast_cancer数据集构建一个二元分类模型。步骤如下:加载数据,准备LightGBM所需的数据,设置参数,训练模型,进行预测,并评估结果。
LightGBM的实现
让我们使用Python创建一个基本模型:
1. 加载数据集
首先,我们使用Scikit-learn的load_breast_cancer方法加载数据集。此数据集包含乳腺癌分类的特征和标签。
from sklearn.datasets import load_breast_cancer # Load dataset data = load_breast_cancer() X = data.data y = data.target
2. 分割数据
使用Scikit-learn的train_test_split方法将数据集分割成训练集和测试集。这允许我们在一个数据集上训练模型,然后在另一个数据集上评估其性能。
from sklearn.model_selection import train_test_split # Split the data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
3. 准备LightGBM所需的数据
将训练数据和测试数据转换为LightGBM数据集格式。此步骤优化了LightGBM训练算法的数据格式。
import lightgbm as lgb # Convert the data to LightGBM dataset format train_data = lgb.Dataset(X_train, label=y_train) test_data = lgb.Dataset(X_test, label=y_test, reference=train_data)
4. 定义参数
设置LightGBM模型的参数。这包括目标函数、评估指标、学习率、叶子节点数量和最大树深度。
params = { 'objective': 'binary', 'metric': 'binary_logloss', 'boosting_type': 'gbdt', 'learning_rate': 0.1, # Increased from 31 'num_leaves': 63, # Set to a positive value to limit depth 'max_depth': 10 }
5. 训练模型
使用训练数据训练LightGBM模型。为了防止过拟合,我们使用早停机制,这意味着当验证集上没有进展时训练结束。
# Train the model with early stopping lgb_model = lgb.train( params, train_data, num_boost_round=100, valid_sets=[test_data], # Use callback for early stopping callbacks=[lgb.early_stopping(stopping_rounds=10)] )
输出
以下是上述步骤的结果:
[LightGBM] [Info] Number of positive: 286, number of negative: 169 [LightGBM] [Info] Auto-choosing col-wise multi-threading, the overhead of testing was 0.000734 seconds. You can set `force_col_wise=true` to remove the overhead. [LightGBM] [Info] Total Bins 4548 [LightGBM] [Info] Number of data points in the train set: 455, number of used features: 30 [LightGBM] [Info] [binary:BoostFromScore]: pavg=0.628571 -> initscore=0.526093 [LightGBM] [Info] Start training from score 0.526093 [LightGBM] [Warning] No further splits with positive gain, best gain: -inf [LightGBM] [Warning] No further splits with positive gain, best gain: -inf
6. 进行预测
使用训练好的模型预测测试数据。我们将概率转换为二元结果。
# Predict on the test set y_pred = lgb_model.predict(X_test) y_pred_binary = [1 if x > 0.5 else 0 for x in y_pred]
7. 评估模型
计算测试集的准确率分数以评估模型的性能。这使我们能够了解模型对以前未见过的数据的性能。
from sklearn.metrics import accuracy_score # Evaluate the model accuracy = accuracy_score(y_test, y_pred_binary) print(f"Accuracy: {accuracy:.2f}")
输出
以下是上述模型的准确率:
Accuracy: 0.96
LightGBM模型的探索性数据分析 (EDA)
在训练和测试LightGBM模型之前,必须进行探索性数据分析 (EDA),以了解数据集,识别模式并准备建模。EDA包括检查数据集的结构、分布、相关性和潜在问题。
以下是使用EDA处理load_breast_cancer数据集的步骤:
1. 加载和检查数据集
首先,我们必须加载数据集并检查其基本结构,例如样本数量、特征和目标变量。
import pandas as pd from sklearn.datasets import load_breast_cancer # Load dataset data = load_breast_cancer() df = pd.DataFrame(data.data, columns=data.feature_names) df['target'] = data.target # Inspect the dataset print(df.head()) print(df.info()) print(df.describe())
输出
这将产生以下结果:
mean radius mean texture mean perimeter mean area mean smoothness \ 0 17.99 10.38 122.80 1001.0 0.11840 1 20.57 17.77 132.90 1326.0 0.08474 2 19.69 21.25 130.00 1203.0 0.10960 3 11.42 20.38 77.58 386.1 0.14250 4 20.29 14.34 135.10 1297.0 0.10030 mean compactness mean concavity mean concave points mean symmetry \ 0 0.27760 0.3001 0.14710 0.2419 1 0.07864 0.0869 0.07017 0.1812 2 0.15990 0.1974 0.12790 0.2069 3 0.28390 0.2414 0.10520 0.2597 4 0.13280 0.1980 0.10430 0.1809 mean fractal dimension ... worst texture worst perimeter worst area \ 0 0.07871 ... 17.33 184.60 2019.0 1 0.05667 ... 23.41 158.80 1956.0 2 0.05999 ... 25.53 152.50 1709.0 3 0.09744 ... 26.50 98.87 567.7 4 0.05883 ... 16.67 152.20 1575.0 worst smoothness worst compactness worst concavity worst concave points \ 0 0.1622 0.6656 0.7119 0.2654 1 0.1238 0.1866 0.2416 0.1860 2 0.1444 0.4245 0.4504 0.2430 3 0.2098 0.8663 0.6869 0.2575 4 0.1374 0.2050 0.4000 0.1625 worst symmetry worst fractal dimension target 0 0.4601 0.11890 0 1 0.2750 0.08902 0 2 0.3613 0.08758 0 3 0.6638 0.17300 0 4 0.2364 0.07678 0
2. 检查缺失值
现在让我们看看数据集中是否存在任何缺失值。
# Check for missing values print(df.isnull().sum())
输出
这将生成以下结果:
mean radius 0 mean texture 0 mean perimeter 0 mean area 0 mean smoothness 0 mean compactness 0 mean concavity 0 mean concave points 0 mean symmetry 0 mean fractal dimension 0 radius error 0 texture error 0 perimeter error 0 area error 0 smoothness error 0 compactness error 0 concavity error 0 concave points error 0 symmetry error 0 fractal dimension error 0 worst radius 0 worst texture 0 worst perimeter 0 worst area 0 worst smoothness 0 worst compactness 0 worst concavity 0 worst concave points 0 worst symmetry 0 worst fractal dimension 0 target 0 dtype: int64
3. 特征分布
确定每个特征的分布。为了更多地了解特征值的范围和分布,可以使用直方图、箱线图或其他可视化方法。
import matplotlib.pyplot as plt import seaborn as sns # Plot histograms for each feature df.iloc[:, :-1].hist(bins=30, figsize=(20, 15)) plt.show() # Box plot for a selected feature sns.boxplot(x='target', y='mean radius', data=df) plt.show()
输出
这将创建以下结果:
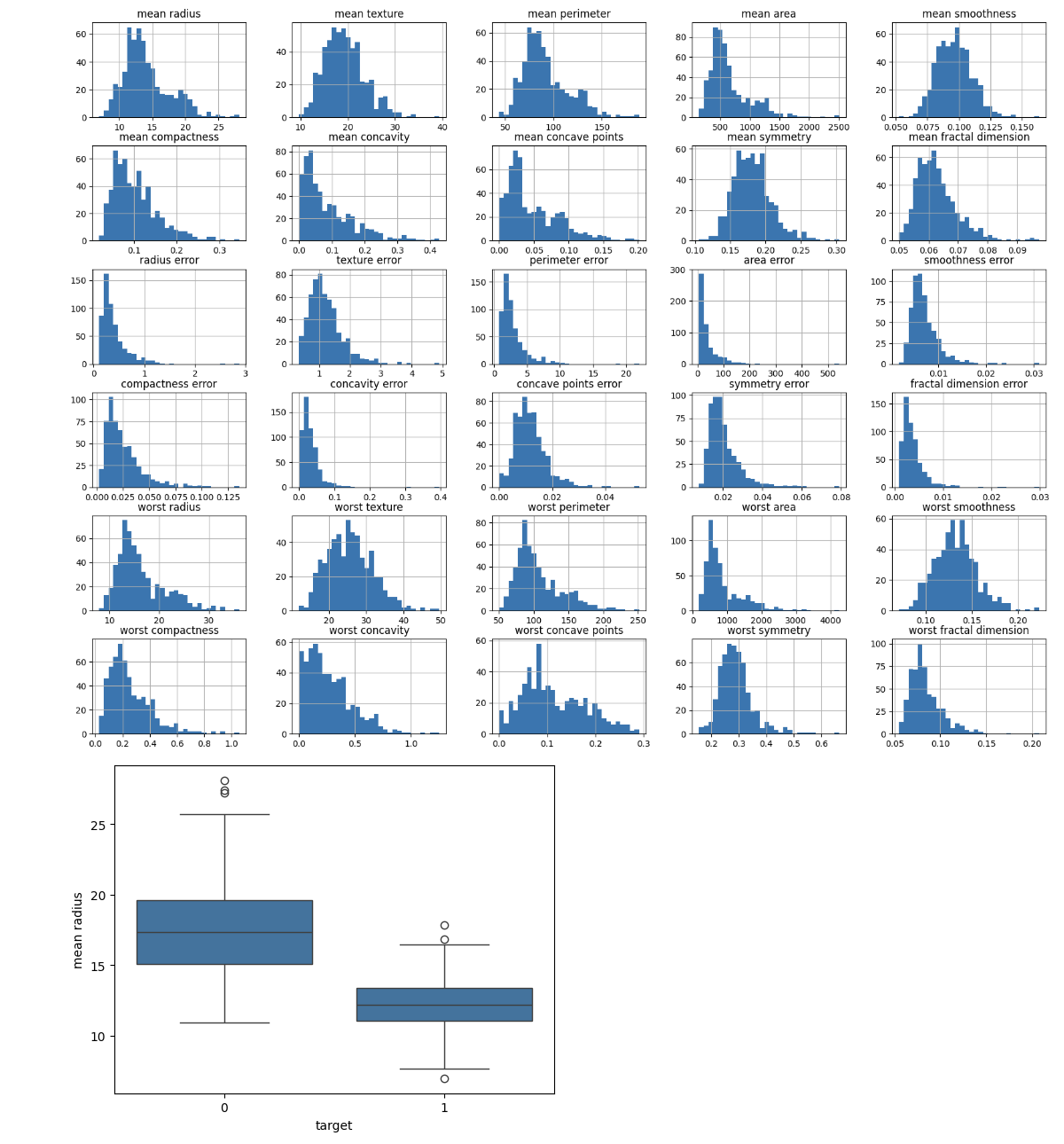
4. 分析类别分布
检查目标变量的分布以查找类别平衡。这将使您能够识别数据集是否不平衡。
# Class distribution print(df['target'].value_counts()) sns.countplot(x='target', data=df) plt.show()
输出
这将显示以下输出:
target 1 357 0 212 Name: count, dtype: int64
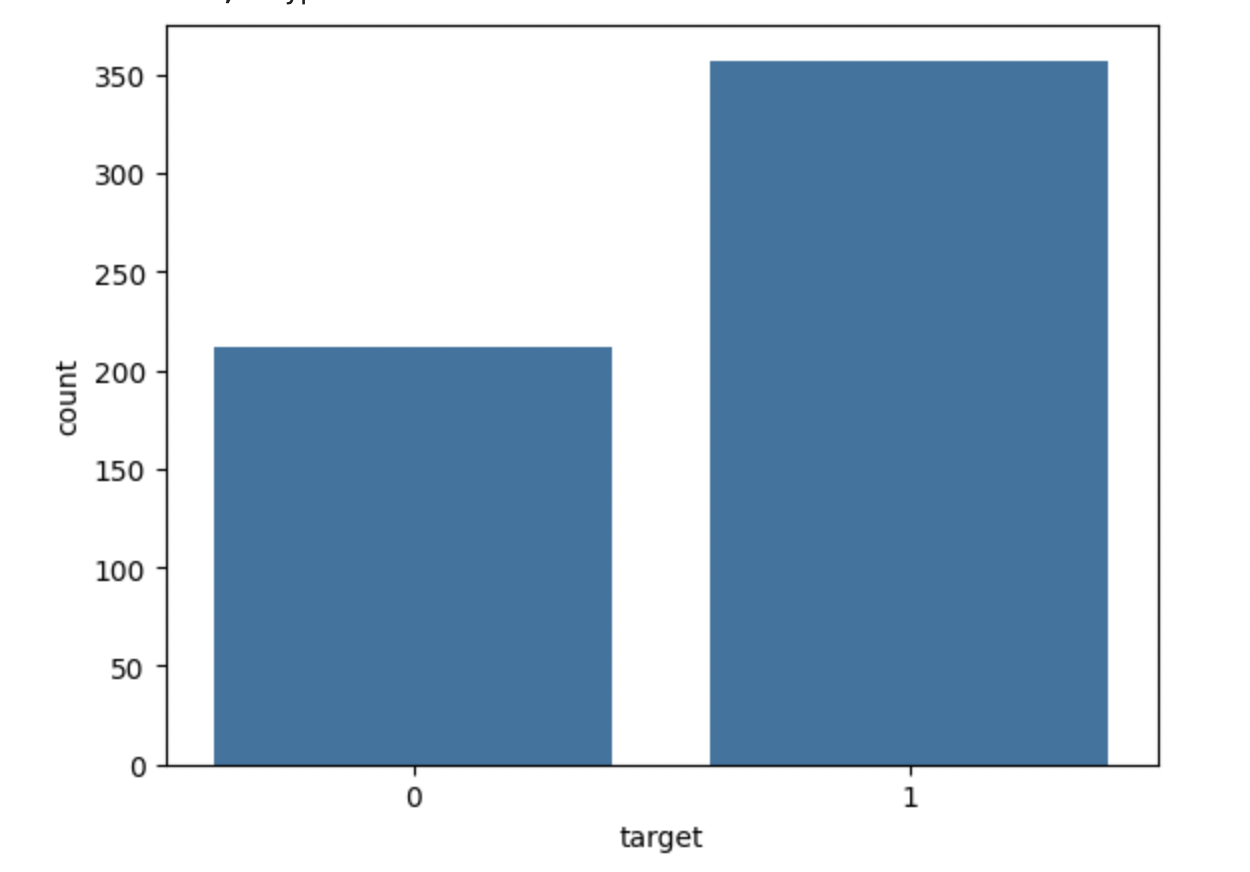
总结
按照这些步骤,您可以创建和测试用于Python中分类问题的LightGBM模型。可以通过根据需要调整参数和准备步骤,将此技术修改为适用于不同的数据集和问题。