Android中的StackView
简介
在开发Android应用程序时,我们经常需要以堆栈的形式显示一组数据。例如,如果我们在应用程序中显示一堆卡片,我们可以将这些卡片以堆栈的形式显示在我们的Android应用程序中。在这篇文章中,我们将了解如何在Android应用程序中使用Stack View。
实现
我们将创建一个简单的应用程序,在这个应用程序中,我们将显示从1到5的数字堆栈,向下滑动每个数字将显示另一个数字。现在让我们转向Android Studio来创建一个新项目。
步骤1:在Android Studio中创建一个新项目
导航到Android Studio,如下面的屏幕所示。在下面的屏幕中,单击“新建项目”以创建一个新的Android Studio项目。
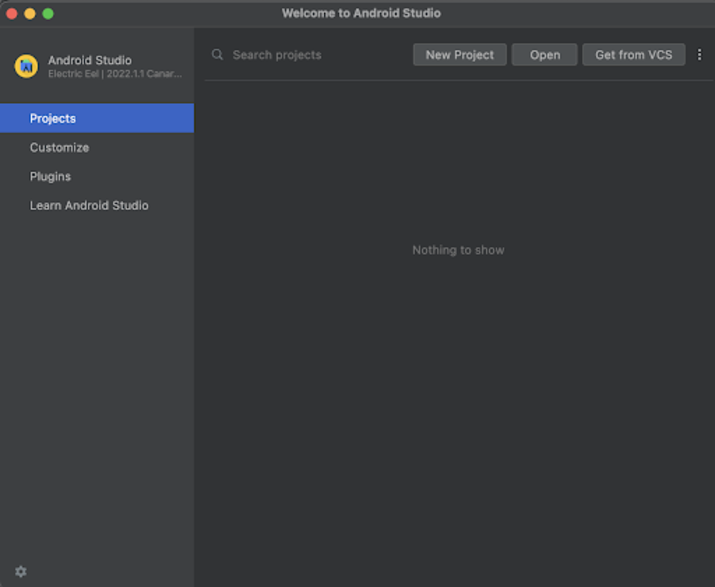
单击“新建项目”后,您将看到下面的屏幕。
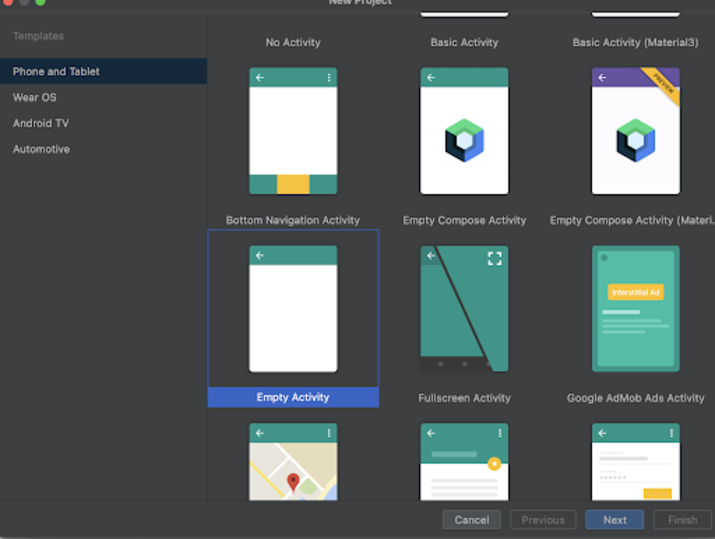
在这个屏幕中,我们只需选择“空活动”,然后单击“下一步”。单击“下一步”后,您将看到下面的屏幕。
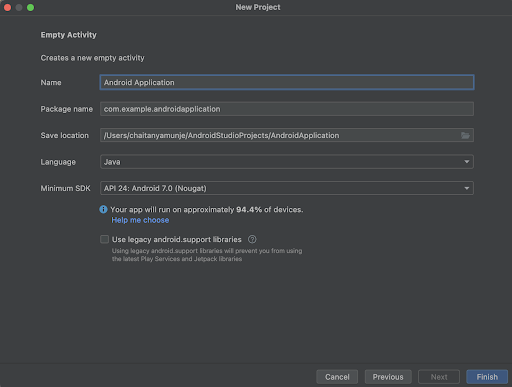
在这个屏幕中,我们只需指定项目名称。然后包名将自动生成。
注意 - 确保选择Java作为语言。
指定所有详细信息后,单击“完成”以创建一个新的Android Studio项目。
项目创建完成后,我们将看到打开的两个文件:activity_main.xml和MainActivity.java文件。
步骤2:使用activity_main.xml
导航到activity_main.xml。如果此文件不可见,则要打开此文件。在左侧窗格中导航到app>res>layout>activity_main.xml以打开此文件。打开此文件后,向其中添加以下代码。代码中添加了注释以详细了解。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <!-- on below line creating a text view for displaying the heading of our application --> <TextView android:id="@+id/idTVHeading" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_above="@id/idStackView" android:layout_margin="5dp" android:gravity="center" android:padding="4dp" android:text="StackView in Android" android:textAlignment="center" android:textColor="@color/black" android:textSize="20sp" android:textStyle="bold" /> <!-- on below line creating a text view for adding a strike through for our text --> <StackView android:id="@+id/idStackView" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_centerInParent="true" /> </RelativeLayout>
说明 - 在上面的代码中,我们创建一个根布局作为相对布局。在这个布局中,我们创建一个TextView,用于显示应用程序的标题。之后,我们创建了一个Stack View,我们将在其中显示数字堆栈。
步骤3:为要在Stack View中显示的项目创建布局文件
导航到app>res>layout>右键单击它>新建布局资源文件,并将其命名为stack_item,然后向其中添加以下代码。代码中添加了注释以详细了解。
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center"> <!-- on below line creating an image view for displaying an image --> <ImageView android:id="@+id/image" android:layout_width="200dp" android:layout_height="200dp" android:layout_centerInParent="true" android:background="@color/white" /> </RelativeLayout>
说明 - 在上面的代码中,我们创建一个根布局作为相对布局。在这个布局中,我们创建了一个ImageView,我们将在应用程序的堆栈中使用它来显示图像。
步骤4:为Stack View创建适配器类
导航到app>java>您的应用程序包名>右键单击它>新建Java类,并将其命名为StackViewAdapter,然后向其中添加以下代码。代码中添加了注释以详细了解。
package com.example.java_test_application; import android.content.Context; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.ArrayAdapter; import android.widget.ImageView; import android.widget.TextView; import androidx.annotation.NonNull; import androidx.annotation.Nullable; import java.util.List; public class StackViewAdapter extends ArrayAdapter { // on below line creating a variable for our array list for images. List<Integer> numbersList; // on below line creating a variable for context. Context context; // on below line creating a constructor and adding list and context to it. public StackViewAdapter(@NonNull Context ctx, int resource, List<Integer> lst) { super(ctx, resource); numbersList = lst; context = ctx; } // on below line creating a get count method. @Override public int getCount() { // on below line returning the size of number list. return numbersList.size(); } // on below line creating a get view method to inflate the layout file. @NonNull @Override public View getView(int position, @Nullable View convertView, @NonNull ViewGroup parent) { // on below line checking if the view is empty or not. if (convertView == null) { // if the view is empty we are inflating the layout file on below line. // on below line specifying the layout file name which we have already created. convertView = LayoutInflater.from(parent.getContext()).inflate(R.layout.stack_item, parent, false); } // on below line creating and initializing the variable for our image view. ImageView imageView = convertView.findViewById(R.id.image); // on below line setting image view from our array list. imageView.setImageResource(numbersList.get(position)); // on below line returning the view. return convertView; } }
说明 - 在上面的代码中,我们为列表和上下文创建了一个变量。之后,我们创建了一个构造函数来初始化这些变量。创建此构造函数后,我们创建了一个getCount方法,它将返回我们传递的ArrayList的大小。最后,我们创建了一个getView方法,它首先将检查视图是否为空。如果视图为空,我们将加载一个布局文件并初始化该布局中存在的视图,例如,在本例中,我们显示一个ImageView。因此,我们为ImageView创建变量并使用我们在stack_item.xml文件中给出的ID对其进行初始化。之后,我们从ArrayList设置ImageView的图像,最后返回我们的视图。
步骤6:在drawable文件夹中添加图像
复制要添加到Stack View中的图像,然后在Android Studio项目中。导航到app>res>drawable,右键单击它并粘贴已复制的图像。
步骤6:使用MainActivity.java文件
导航到MainActivity.java。如果此文件不可见,则要打开此文件。在左侧窗格中导航到app>res>layout>MainActivity.java以打开此文件。打开此文件后,向其中添加以下代码。代码中添加了注释以详细了解。
package com.example.java_test_application; import android.os.Bundle; import android.widget.StackView; import androidx.appcompat.app.AppCompatActivity; import java.util.ArrayList; import java.util.List; public class MainActivity extends AppCompatActivity { // creating variables for stack view on below line. private StackView stackView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // initializing variables for stack view on below line. stackView = findViewById(R.id.idStackView); // creating a variable for list and adding data to it. List<Integer> numberList = new ArrayList<>(); numberList.add(R.drawable.numberone); numberList.add(R.drawable.numbertwo); numberList.add(R.drawable.numberthree); numberList.add(R.drawable.numberfour); numberList.add(R.drawable.numberfive); // on below line creating and initializing the variable for adapter for stack view. StackViewAdapter stackViewAdapter = new StackViewAdapter(this, R.layout.stack_item, numberList); stackView.setAdapter(stackViewAdapter); } }
说明 - 在上面的代码中,我们首先为Stack View创建变量。现在我们将看到onCreate方法。这是每个Android应用程序的默认方法。创建应用程序视图时将调用此方法。在此方法中,我们设置内容视图,即名为activity_main.xml的布局文件,以从该文件中设置UI。在onCreate方法中,我们使用我们在activity_main.xml文件中给出的ID初始化Stack View变量。之后,我们创建整数ArrayList并对其进行初始化。初始化后,我们将数据添加到其中。我们将drawable文件夹中添加的图像添加到ArrayList中。之后,我们创建一个适配器类并将ArrayList传递给它。最后,我们将此适配器设置为Stack View以显示堆栈。
添加上述代码后,我们只需单击顶部栏中的绿色图标即可在移动设备上运行我们的应用程序。
注意 - 确保已连接到您的真实设备或模拟器。
输出
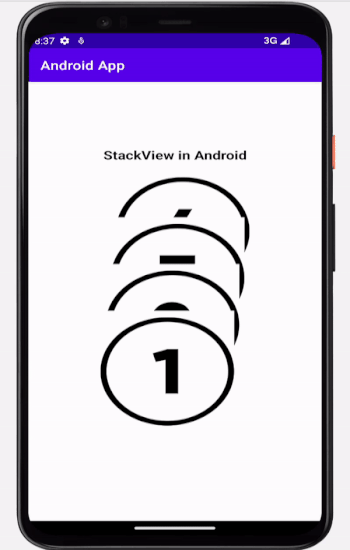
结论
在本文中,我们了解了如何在Android中创建Stack View。