在Snack中使用列表
有时,任务是将多个项目存储和显示为列表。对于这个React Native组件,可以使用FlatList。FlatList也可以设置为可选择或可点击的。在这篇文章中,将展示React Native和Javascript代码,其中包含两个不同的示例,第一个示例将列表的项目存储为具有唯一ID的可识别的键值对数组,然后获取和渲染。
算法
算法-1
步骤1 − 从'react-native'导入FlatList、Text和View。
步骤2 − 创建App.js并编写存储列表的代码。列表以数组的形式存在,但存储为键值对。每个项目都有一个唯一的ID。
步骤3 − 创建一个箭头函数“showlist”来设置状态,使其包含所有列表项。
步骤4 − 获取状态变量列表并逐一渲染所有项目。
步骤5 − 使用所需的样式,View和Text用于在屏幕上显示项目。
示例1:在FlatList中显示所有项目。
项目中使用的重要文件是
App.js
App.js:这是此项目的Javascript主文件。
示例
import React from 'react'; import {FlatList,Text, View} from 'react-native'; export default class FetchExample extends React.Component { constructor(props){ super(props); this.state ={ list:[ { "id": 1, "name": "Sector 15 A", "city": "Faridabad", "lat": 28.395403, "lng": 77.315292, }, { "id": 2, "name": "Borivali", "city": "Mumbai", "lat": 19.228825, "lng": 72.854118, }, { "id": 3, "name": "Kolutolla", "city": "Kolkata", "lat": 22.578005, "lng": 88.358536, }, { "id": 4, "name": "Benz Circle", "city": "Vijayawada", "lat": 16.499725, "lng": 80.656067, }, ], keyid:0, } } showitem= ()=>{ for(var i=0;i<this.state.list.length;i++){ this.setState({keyid:this.state.keyid+1}) } } render(){ return( <View style={{flex: 1, paddingTop:5, backgroundColor: 'forestgreen'}}> <Text style={{padding:60,fontSize: 18, fontWeight: 'bold'}}>Latitude and Longitude</Text> <View style={{flexDirection:'row', justifyContent: 'space-between', alignItems:'center', padding:10, backgroundColor: 'brown', margin: 10}}> <Text style={{fontSize: 18, fontWeight: 'bold'}}>Place</Text> <Text style={{fontSize: 18, fontWeight: 'bold'}}>City</Text> <Text style={{fontSize: 18, fontWeight: 'bold'}}>Latitude</Text> <Text style={{fontSize: 18, fontWeight: 'bold'}}>Longitude</Text> </View> <FlatList data={this.state.list} renderItem={({item}) => ( <View style={{flexDirection:'row', justifyContent: 'space-between', alignItems:'center', padding:10, backgroundColor: 'lawngreen', margin: 10}}> <Text>{item.name}</Text> <Text>{item.city}</Text> <Text>{item.lat}</Text> <Text>{item.lng}</Text> </View> )} keyExtractor={(item, index) => index} /> </View> ); } }
查看结果
结果可以在线查看。当用户输入代码时,Web视图将默认选中,结果会立即显示。
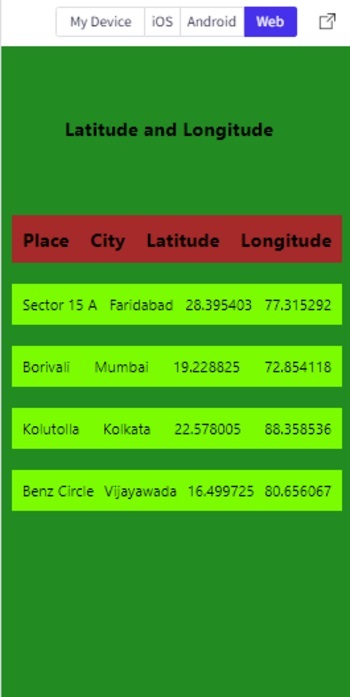
在Snack的Web视图中显示FlatList项目
算法-2
步骤1 − 从'react-native'导入FlatList、TouchableOpacity、Alert、Text和View。
步骤2 − 创建App.js并编写存储列表的代码。列表以数组的形式存在,但存储为键值对。每个项目都有一个唯一的ID。
步骤3 − 创建一个箭头函数“showlist”来设置状态,使其包含所有列表项。
步骤4 − 获取状态变量列表并逐一渲染所有项目。
步骤5 − 要使FlatList项目可点击,请使用具有所需样式的TouchableOpacity。
步骤6 − 函数onPress()指定单击列表项时要执行的操作。例如,此处将JSON对象转换为文本后,在警报中显示纬度和经度值作为结果。
示例2:使FlatList中的项目可点击以显示与所选项目相关的结果。
项目中使用的重要文件是
App.js
App.js:这是此项目的Javascript主文件。
示例
import React from 'react'; import {Alert, FlatList,Text, View,TouchableOpacity} from 'react-native'; export default class FetchExample extends React.Component { constructor(props){ super(props); this.state ={ list:[ { "id": 1, "name": "Sector 15 A", "city": "Faridabad", "lat": 28.395403, "lng": 77.315292, }, { "id": 2, "name": "Borivali", "city": "Mumbai", "lat": 19.228825, "lng": 72.854118, }, { "id": 3, "name": "Kolutolla", "city": "Kolkata", "lat": 22.578005, "lng": 88.358536, }, { "id": 4, "name": "Benz Circle", "city": "Vijayawada", "lat": 16.499725, "lng": 80.656067, }, ], keyid:0, } } showitem= ()=>{ for(var i=0;i<this.state.list.length;i++){ this.setState({keyid:this.state.keyid+1}) } } render(){ return ( <View style={{flex: 1, paddingTop:5, backgroundColor: 'forestgreen'}}> <Text style={{padding:60,fontSize: 18, fontWeight: 'bold'}}>Latitude and Longitude</Text> <View style={{flexDirection:'row', justifyContent: 'space-between', alignItems:'center', padding:10, backgroundColor: 'brown', margin: 10}}> <Text style={{fontSize: 18, fontWeight: 'bold'}}>Place</Text> <Text style={{fontSize: 18, fontWeight: 'bold'}}>City</Text> <Text style={{fontSize: 18, fontWeight: 'bold'}}>Latitude</Text> <Text style={{fontSize: 18, fontWeight: 'bold'}}>Longitude</Text> </View> <FlatList data={this.state.list} renderItem={ ({item ,index}) => ( <View style={{flex: 1, flexDirection:'row', justifyContent: 'space-between', alignItems:'center', padding:10, backgroundColor: 'lawngreen', margin: 10}}> <TouchableOpacity key={index.toString()} onPress={() =>{ let str= JSON.stringify(item.name); let str1 = JSON.stringify(item.lat); let str2 = JSON.stringify(item.lng); Alert.alert(str +" --" + "latitude: " +str1+ "" + "
longitude: " +str2); //alert(str +" --" + "latitude: " +str1+ "" + "
longitude: " +str2); } } > <Text>{item.name}</Text> <Text>{item.city}</Text> </TouchableOpacity> </View> ) } keyExtractor={(item, index) => index} /> </View> ); } }
查看结果
结果可以在线查看。当用户输入代码时,Web视图将默认选中,结果会立即显示。Alert.alert()函数可能不会在Web视图中显示结果。因此,要查看结果,可以使用简单的alert()。代码示例中显示了这两个语句。其中一个可以保留为注释。
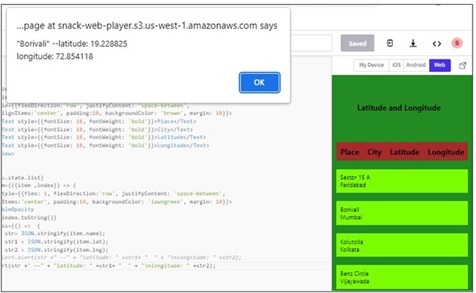
在个人手机上选择项目时,显示简单警报的可点击FlatList项目
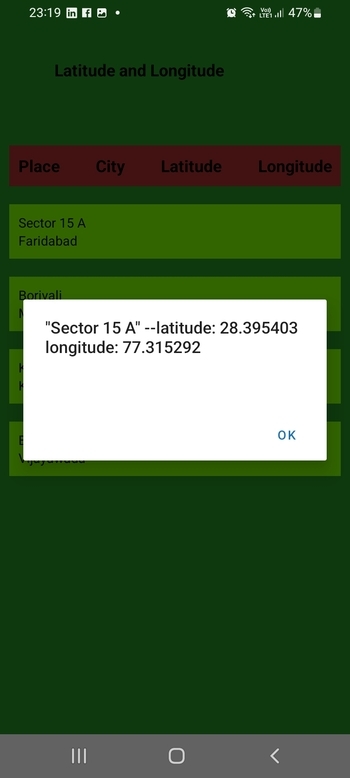
在个人手机上显示警报的可点击FlatList项目
本文通过两个不同的示例,介绍了在Expo Snack上显示FlatList的方法。首先介绍了存储列表项的方法,然后介绍了获取列表项并将其显示为FlatList的过程。示例2中还指定了单击FlatList项目并执行操作的方法。此外,还显示了在线Web视图和个人移动设备上的输出。