在Snack中使用标签页
标签页用于在应用程序中实现多页视图。标签页通常位于屏幕顶部或底部。一些库允许在移动应用程序中创建标签页。也可以使用简单的组件(如按钮)来创建标签页。本文展示了 React Native 和 Javascript 代码,其中包含两个不同的示例:第一个示例使用按钮创建标签页;第二个示例使用 `'@react-navigation/material-top-tabs'` 中的 `createMaterialTopTabNavigator` 创建标签页,然后将其渲染到设备屏幕上。
算法 1
步骤 1 − 从 'react-native' 中导入 Text、View、StyleSheet 和 Button。
步骤 2 − 编写单独的函数以显示不同页面内容。
步骤 3 − 创建 App.js 并编写代码。创建四个按钮并将它们放置为顶部标签。
步骤 4 − 为指定的标签创建页面内容,并通过按钮点击显示它。
步骤 5 − 创建一个新的样式并将其命名为 selectedOne。选择给定标签时设置此样式。这将使指定的标签在该行中看起来与其他未选择的标签不同。
步骤 6 − 检查结果。
示例 1:在 Snack 中使用按钮创建标签页。
项目中使用的重要文件是
App.js
App.js:这是该项目的 Javascript 主文件。
示例
import{Component}from'react'; import{Text,View,StyleSheet,Button}from'react-native'; exportdefaultclassAppextendsComponent{ constructor(props){ super(props); this.state={ LessonSelected:'One', btnClr:"#6690ad", }; } render(){ return( <Viewstyle={styles.mainSpace}> <Viewstyle={styles.Lessonbuttons}> <Viewstyle={this.state.LessonSelected==='One'?styles.selectedOne:null}> <Button onPress={()=>{ this.setState({LessonSelected:'One'}); }} title="Lesson1" color={this.state.btnClr} borderColor='#000' /> </View> <Viewstyle={this.state.LessonSelected==='Two'?styles.selectedOne:null}> <Button onPress={()=>{ this.setState({LessonSelected:'Two'}); }} title="Lesson2" color={this.state.btnClr} /> </View> <Viewstyle={this.state.LessonSelected==='Three'?styles.selectedOne:null}> <Button onPress={()=>{ this.setState({LessonSelected:'Three'}); }} title="Lesson3" color={this.state.btnClr} /> </View> <Viewstyle={this.state.LessonSelected==='Four'?styles.selectedOne:null}> <Button onPress={()=>{ this.setState({LessonSelected:'Four'}); }} title="Lesson4" color={this.state.btnClr} /> </View> </View> {this.state.LessonSelected==='One'?this.showLesson1() :this.state.LessonSelected==='Two'?this.showLesson2() :this.state.LessonSelected==='Three'?this.showLesson3() :this.state.LessonSelected==='Four'?this.showLesson4() :this.showLesson1() } </View> ); } showLesson1=()=>( <Viewstyle={[styles.Lessoncontents,styles.Lessons]}> <Textstyle={styles.LessonHeader}>Lesson1</Text> <Textstyle={styles.LessonContent}>Contents</Text> </View> ); showLesson2=()=>( <Viewstyle={[styles.Lessoncontents,styles.Lessons]}> <Textstyle={styles.LessonHeader}>Lesson2</Text> <Textstyle={styles.LessonContent}>Contents</Text> </View> ); showLesson3=()=>( <Viewstyle={[styles.Lessoncontents,styles.Lessons]}> <Textstyle={styles.LessonHeader}>Lesson3</Text> <Textstyle={styles.LessonContent}>Contents</Text> </View> ); showLesson4=()=>( <Viewstyle={[styles.Lessoncontents,styles.Lessons]}> <Textstyle={styles.LessonHeader}>Lesson4</Text> <Textstyle={styles.LessonContent}>Contents</Text> </View> ); } conststyles=StyleSheet.create({ mainSpace:{ flex:1, marginTop:50, }, Lessonbuttons:{ width:'100%', flexDirection:'row', justifyContent:'space-between', }, Lessoncontents:{ flex:1, width:'100%', padding:10, marginTop:10, borderWidth:1, borderColor:'black', }, LessonHeader:{ color:'black', fontSize:28, textAlign:'center', }, selectedOne:{ borderBottomWidth:10, borderBottomColor:'#ffc181', }, });
输出
虽然结果可以在线查看,但此处显示的是在个人移动设备上的结果。
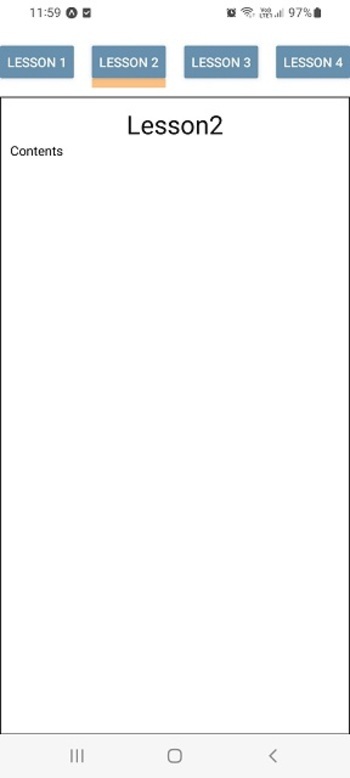
算法 2
步骤 1 − 从 'react-native' 中导入 Text、View、StyleSheet。
步骤 2 − 从 '@react-navigation/native' 导入 NavigationContainer,然后从 '@react-navigation/material-top-tabs' 导入 createMaterialTopTabNavigator。
步骤 3 − 编写单独的函数以显示不同页面内容。
步骤 4 − 创建 App.js 并编写代码。使用 createMaterialTopTabNavigator() 创建 LessonTab。
步骤 5 − 创建 NavigationContainer 标签,并在其中创建 LessonTab.Navigator。从不同的 LessonTab.Screen 调用页面内容函数。
步骤 6 − 检查结果。
示例 2:使用 `'@react-navigation/material-top-tabs'` 中的 `createMaterialTopTabNavigator` 创建标签页。
项目中使用的重要文件是
App.js
App.js:这是该项目的 Javascript 主文件。
示例
import {Component} from 'react'; import { Text, View, StyleSheet } from 'react-native'; import { NavigationContainer } from '@react-navigation/native'; import { createMaterialTopTabNavigator } from '@react-navigation/material-top-tabs'; const LessonTab = createMaterialTopTabNavigator(); export default class TopTabs extends Component { constructor(props) { super(props); this.state = { LessonSelected: 'One', btnClr: "#6690ad", }; } render(){ return ( <View style={styles.mainSpace}> <NavigationContainer> <LessonTab.Navigator tabBarOptions={{ activeTintColor: '#e3486a', inactiveTintColor: '#7448e3', }} > <LessonTab.Screen name="Lesson 1" component={this.showLesson1} /> <LessonTab.Screen name="Lesson 2" component={this.showLesson2} /> <LessonTab.Screen name="Lesson 3" component={this.showLesson3} /> <LessonTab.Screen name="Lesson 4" component={this.showLesson4} /> </LessonTab.Navigator> </NavigationContainer> </View> ); } showLesson1 = () => ( <View style={[styles.Lessoncontents, styles.Lessons]}> <Text style={styles.LessonHeader}>Lesson1</Text> <Text style={styles.LessonContent}>Contents</Text> </View> ); showLesson2 = () => ( <View style={[styles.Lessoncontents, styles.Lessons]}> <Text style={styles.LessonHeader}>Lesson2</Text> <Text style={styles.LessonContent}>Contents</Text> </View> ); showLesson3 = () => ( <View style={[styles.Lessoncontents, styles.Lessons]}> <Text style={styles.LessonHeader}>Lesson3</Text> <Text style={styles.LessonContent}>Contents</Text> </View> ); showLesson4 = () => ( <View style={[styles.Lessoncontents, styles.Lessons]}> <Text style={styles.LessonHeader}>Lesson 4 </Text> <Text style={styles.LessonContent}>Contents</Text> </View> ); } const styles = StyleSheet.create({ mainSpace: { flex: 1, marginTop: 50, }, Lessoncontents: { flex: 1, width: '100%', padding: 10, marginTop: 10, borderWidth: 1, borderColor: 'black', }, LessonHeader: { color: 'black', fontSize: 28, textAlign: 'center', }, });
输出
结果可以在线查看。当用户输入代码时,Web 视图将默认选中,结果会立即显示。
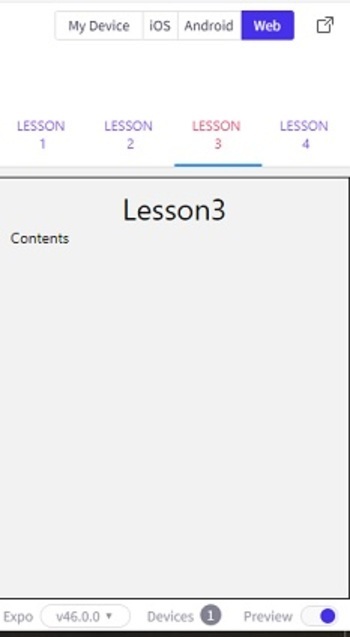
本文使用两个不同的示例介绍了在 Expo Snack 上创建顶部标签页的方法。首先介绍了使用简单按钮创建标签页的方法,然后以使用 `'@react-navigation/material-top-tabs'` 中的 `createMaterialTopTabNavigator` 创建顶部标签页为例,并展示了这些方法在线 Web 视图和个人移动设备上的输出。