在Snack中制作双屏幕应用程序
在React Native应用中,通常需要多个屏幕,用户需要在屏幕之间切换。本文使用两种不同的方法来制作双屏幕应用程序,用户可以在两个屏幕之间来回切换。在示例1中,使用'react-navigation'中的createStackNavigator模块实现导航,而在示例2中,使用createSwitchNavigator创建导航。
算法
算法1
步骤1 − 从'react-navigation'导入createStackNavigator
步骤2 − 创建App.js并在javascript文件中编写从一个屏幕导航到另一个屏幕的代码。
步骤3 − 在App.js文件中创建两个屏幕作为类My_Home和My_School。
步骤4 − 设置导航选项,例如标题和headerStyle。
步骤5 − 编写从My_Home导航到My_School,然后再返回My_Home的代码。
步骤6 − 在WebView上立即查看在线结果。
算法2
步骤1 − 创建一个名为screens的文件夹,并创建名为FirstScreen.js和SecondScreen.js的javascript文件。在这些文件中为FirstScrr和SecondScrr编写代码。
步骤2 − 从'react-navigation'库导入名为createAppContainer和createSwitchNavigator的模块。
步骤3 − 创建App.js并在其中编写从FirstScrr到SecondScrr,然后再返回FirstScrr的代码。
步骤4 − 使用按钮的onPress()函数在FirstScrr和SecondScrr之间切换。
步骤5 − 在WebView上立即查看在线结果。
多种方法
我们提供了多种不同的解决方案。
使用'react-navigation'中的createStackNavigator
使用'react-navigation'库中的createAppContainer和createSwitchNavigator
让我们逐一查看程序及其输出。
方法1:使用'react-navigation'中的createStackNavigator
项目中的重要文件
App.js
package.json
两个图像文件myhome.jpg和myschool.jpg
App.js:这是主要的javascript文件。
示例
import {Component} from 'react'; import { Button,Image, View } from 'react-native'; import {createStackNavigator} from 'react-navigation'; export default class TheTwoScreenApp extends Component { render() { return <TheTwoScreenNavigator />; } } class My_Home extends Component { navopt = { title: 'The Home', headerStyle: {backgroundColor:"#499AC8"} }; render() { return ( <View style={{ flex: 1, justifyContent: 'center' }}> <Image style={{ width: 300, height: 300, margin:15 }} source={require('./myhome.jpg')} /> <Button title="Go to Your School" onPress={() => this.props.navigation.navigate('My_School') } /> </View> ); } } class My_School extends Component { navopt = { title: 'The School', headerStyle: {backgroundColor:"#499AC8"} }; render() { return ( <View style={{ flex: 1, justifyContent: 'center', }}> <Image style={{ width: 300, height: 300, margin:15 }} source={require('./myschool.jpg')} /> <Button title="Go back to Your Home" onPress={() => this.props.navigation.navigate('My_Home') } /> </View> ); } } const TheTwoScreenNavigator = createStackNavigator( { My_Home: My_Home, My_School: My_School, }, );
package.json:这个文件包含所需的依赖项。
示例
{ "dependencies": { "react-navigation": "2.0.0-rc.2", "@expo/vector-icons": "^13.0.0" } }
查看结果
起始屏幕输出
结果可以在线查看。
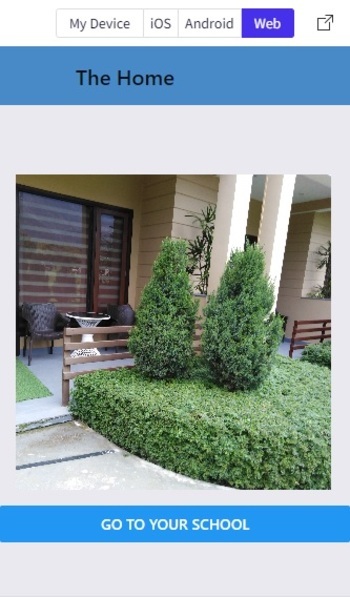
显示起始屏幕(主页)作为WebView
下一个屏幕输出
如果用户点击第一个屏幕上的按钮,导航将跳转到下一个屏幕。
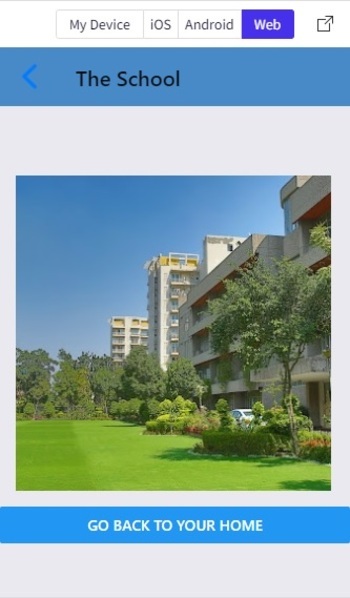
显示下一个屏幕(学校)作为WebView。
方法2:使用'react-navigation'库中的createAppContainer和createSwitchNavigator
项目中的重要文件
文件夹名称screens包含FirstScreen.js和SecondScreen.js
App.js
两个图像文件myhome.jpg和myschool.jpg
FirstScreen.js:这是包含FirstScrr代码的javascript文件
示例
import {Component} from 'react'; import {View,Button, Text, Image, StyleSheet} from 'react-native'; export default class FirstScrr extends Component { render() { return ( <View style={styles.mainSpace}> <Text style={{textAlign:"center", fontSize:30, color: "#357EC7"}}>The Home</Text> <Image style={{ width: 304, height: 304, margin:15, paddingTop: 40}} source={require('../myhome.jpg')} /> <Button title="Go to Your School" onPress={() => this.props.navigation.navigate('SecondScrr') } /> </View> ); } } const styles = StyleSheet.create({ mainSpace: { flex: 1, alignItems: 'center', justifyContent: 'center', marginTop: 36, backgroundColor: '#ecf0f1', }, });
SecondScreen.js:这是包含第二个屏幕代码的javascript文件
示例
import{Component}from'react'; import{View,Button,Text,Image,StyleSheet}from'react-native'; exportdefaultclassSecondScrrextendsComponent{ render(){ return( <Viewstyle={styles.mainSpace}> <Textstyle={{textAlign:"center",fontSize:30,color:"#357EC7"}}>TheSchool</Text> <Image style={{width:304,height:304,margin:15}} source={require('../myschool.jpg')}/> <Button title="GobacktoYourHome" onPress={()=> this.props.navigation.navigate('FirstScrr') } /> </View> ); } } conststyles=StyleSheet.create({ mainSpace:{ flex:1, alignItems:'center', justifyContent:'center', marginTop:36, backgroundColor:'#ecf0f1', }, });
App.js:这是该项目的主要javascript文件。
示例
import {Component} from 'react'; import {View} from 'react-native'; import { createAppContainer, createSwitchNavigator } from 'react-navigation'; import FirstScrr from './screens/FirstScreen'; import SecondScrr from './screens/SecondScreen'; export default class TheTwoScreenDemoExample extends Component { render() { return ( <View> <AppBox /> </View> ); } } var AppNav = createSwitchNavigator({ FirstScrr: FirstScrr, SecondScrr: SecondScrr, }); const AppBox = createAppContainer(AppNav);
查看结果
FirstScreen输出
结果可以在线查看。
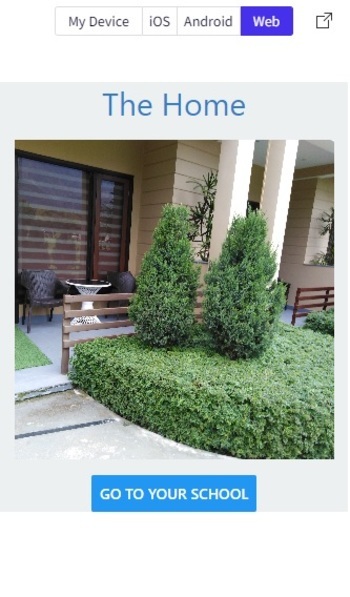
显示FirstScreen作为WebView
SecondScreen输出
如果用户点击FirstScreen上的按钮,导航将跳转到SecondScreen。
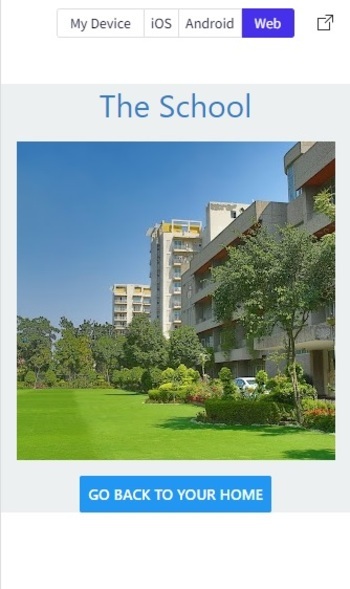
显示第二个屏幕作为WebView。
本文展示了在应用程序中使用多个屏幕的两种方法。使用两种不同的导航方法给出了如何在屏幕之间导航的过程。在第一种方法中,使用StackNavigator,而在第二种方法中,使用SwitchNavigator。