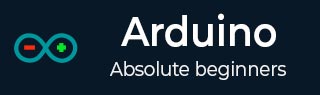
- Arduino 教程
- Arduino - 首页
- Arduino - 概述
- Arduino - 开发板介绍
- Arduino - 安装
- Arduino - 程序结构
- Arduino - 数据类型
- Arduino - 变量与常量
- Arduino - 运算符
- Arduino - 控制语句
- Arduino - 循环
- Arduino - 函数
- Arduino - 字符串
- Arduino - 字符串对象
- Arduino - 时间
- Arduino - 数组
- Arduino 函数库
- Arduino - I/O 函数
- Arduino - 高级 I/O 函数
- Arduino - 字符函数
- Arduino - 数学库
- Arduino - 三角函数
- Arduino 高级应用
- Arduino - Due & Zero
- Arduino - 脉宽调制 (PWM)
- Arduino - 随机数
- Arduino - 中断
- Arduino - 通信
- Arduino - I2C 通信
- Arduino - SPI 通信
- Arduino 项目
- Arduino - LED 闪烁
- Arduino - LED 渐变
- Arduino - 读取模拟电压
- Arduino - LED 条形图
- Arduino - 键盘注销
- Arduino - 键盘消息
- Arduino - 鼠标按键控制
- Arduino - 键盘串口
- Arduino 传感器
- Arduino - 湿度传感器
- Arduino - 温度传感器
- Arduino - 水位检测/传感器
- Arduino - PIR 传感器
- Arduino - 超声波传感器
- Arduino - 连接开关
- 电机控制
- Arduino - 直流电机
- Arduino - 伺服电机
- Arduino - 步进电机
- Arduino 与声音
- Arduino - 音调库
- Arduino - 无线通信
- Arduino - 网络通信
- Arduino 有用资源
- Arduino - 快速指南
- Arduino - 有用资源
- Arduino - 讨论
Arduino - LED 渐变
此示例演示了如何使用 `analogWrite()` 函数实现 LED 渐变熄灭效果。`analogWrite()` 使用脉宽调制 (PWM),通过以不同的比例快速开关数字引脚,从而创建渐变效果。
所需元件
您将需要以下元件:
- 1 个面包板
- 1 个 Arduino Uno R3
- 1 个 LED
- 1 个 330Ω 电阻
- 2 根跳线
步骤
按照电路图,将元件连接到面包板,如下图所示。
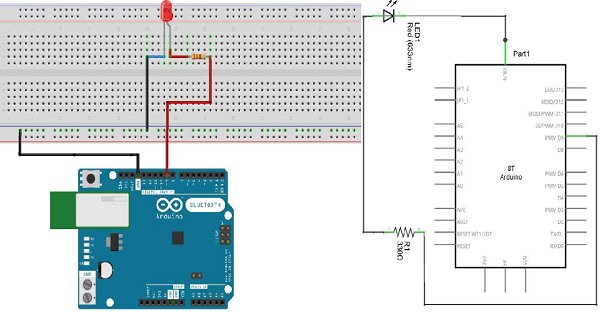
注意 - 要确定 LED 的极性,仔细观察它。较短的一端(靠近灯泡平面的那端)表示负极。
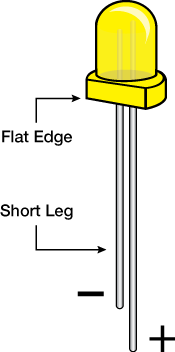
电阻等元件需要将其引脚弯成 90° 角才能正确插入面包板的插孔。您也可以将引脚剪短。
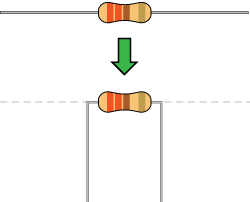
代码
在您的电脑上打开 Arduino IDE 软件。使用 Arduino 语言编写代码来控制您的电路。点击“新建”打开新的代码文件。
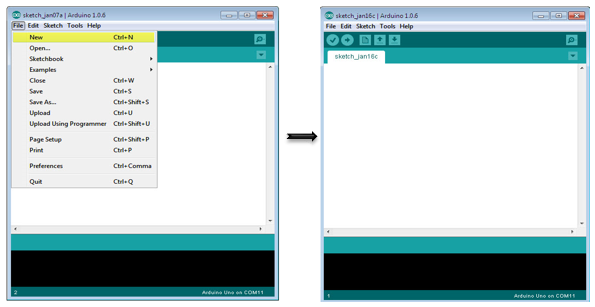
Arduino 代码
/* Fade This example shows how to fade an LED on pin 9 using the analogWrite() function. The analogWrite() function uses PWM, so if you want to change the pin you're using, be sure to use another PWM capable pin. On most Arduino, the PWM pins are identified with a "~" sign, like ~3, ~5, ~6, ~9, ~10 and ~11. */ int led = 9; // the PWM pin the LED is attached to int brightness = 0; // how bright the LED is int fadeAmount = 5; // how many points to fade the LED by // the setup routine runs once when you press reset: void setup() { // declare pin 9 to be an output: pinMode(led, OUTPUT); } // the loop routine runs over and over again forever: void loop() { // set the brightness of pin 9: analogWrite(led, brightness); // change the brightness for next time through the loop: brightness = brightness + fadeAmount; // reverse the direction of the fading at the ends of the fade: if (brightness == 0 || brightness == 255) { fadeAmount = -fadeAmount ; } // wait for 30 milliseconds to see the dimming effect delay(300); }
代码注释
在将 9 号引脚声明为 LED 引脚后,在代码的 `setup()` 函数中无需执行任何操作。您将在代码主循环中使用的 `analogWrite()` 函数需要两个参数:一个参数指示要写入的引脚,另一个参数指示要写入的 PWM 值。
为了使 LED 逐渐熄灭和点亮,我们将 PWM 值从 0(完全熄灭)逐渐增加到 255(完全点亮),然后再返回 0,以完成一个循环。在上图所示的代码中,PWM 值使用名为 `brightness` 的变量设置。每次循环,它都会增加 `fadeAmount` 变量的值。
如果 `brightness` 的值达到任一极值(0 或 255),则 `fadeAmount` 将变为其相反数。换句话说,如果 `fadeAmount` 为 5,则将其设置为 -5。如果为 -5,则将其设置为 5。在下一次循环中,此更改也会导致 `brightness` 改变方向。
`analogWrite()` 可以非常快速地更改 PWM 值,因此代码末尾的 `delay()` 函数控制渐变的速度。尝试更改 `delay()` 的值,看看它如何改变渐变效果。
结果
您应该看到 LED 亮度逐渐变化。