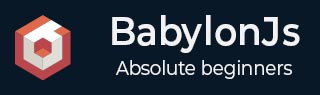
- BabylonJS 教程
- BabylonJS - 主页
- BabylonJS - 简介
- BabylonJS - 环境设置
- BabylonJS - 概览
- BabylonJS - 基本元素
- BabylonJS - 材质
- BabylonJS - 动画
- BabylonJS - 相机
- BabylonJS - 灯光
- BabylonJS - 参数化形状
- BabylonJS - 网格
- VectorPosition 和 Rotation
- BabylonJS - 贴花
- BabylonJS - Curve3
- BabylonJS - 动态纹理
- BabylonJS - 视差贴图
- BabylonJS - 镜头光晕
- BabylonJS - 创建屏幕截图
- BabylonJS - 反射探针
- 标准渲染管道
- BabylonJS - ShaderMaterial
- BabylonJS - 骨骼
- BabylonJS - 物理引擎
- BabylonJS - 播放声音和音乐
- BabylonJS 有用资源
- BabylonJS - 快速指南
- BabylonJS - 有用资源
- BabylonJS - 讨论
BabylonJS - 位置
演示
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3(0, 1, 0); var camera = new BABYLON.ArcRotateCamera("Camera", 1, 0.8, 10, new BABYLON.Vector3(0, 0, 0), scene); scene.activeCamera.attachControl(canvas); var light = new BABYLON.PointLight("Omni", new BABYLON.Vector3(0, 100, 100), scene); var boxa = BABYLON.Mesh.CreateBox("BoxA", 1.0, scene); boxa.position = new BABYLON.Vector3(0,0.5,0); var boxb = BABYLON.Mesh.CreateBox("BoxB", 1.0, scene); boxb.position = new BABYLON.Vector3(3,0.5,0); var boxc = BABYLON.Mesh.CreateBox("BoxC", 1.0, scene); boxc.position = new BABYLON.Vector3(-3,0.5,0); var boxd = BABYLON.Mesh.CreateBox("BoxD", 1.0, scene); boxd.position = new BABYLON.Vector3(0,0.5,3); var boxe = BABYLON.Mesh.CreateBox("BoxE", 1.0, scene); boxe.position = new BABYLON.Vector3(0,0.5,-3); var ground = BABYLON.Mesh.CreateGround("ground1", 10, 6, 2, scene); ground.position = new BABYLON.Vector3(0,0,0); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
输出
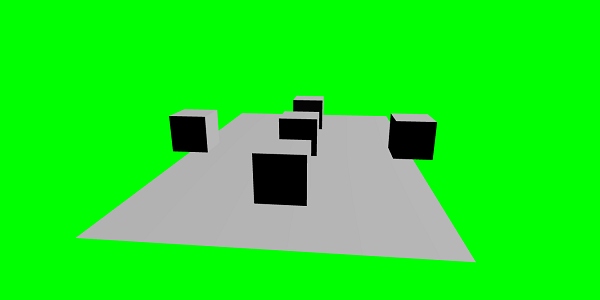
演示
在以上示例中,我们创建了 5 个尺寸为 1 的方块,即方块的边长为 1。我们创建了一个地面并将其放置在中心。
第一个方块,即方块 A 放置在地面中央上方。我们可以使用 new BABYLON.Vector3(x, y, z) 或 shape.position.x , shape.position.y 或 shape.position.z 来放置形状。在以上示例中,我们使用了 new BABYLON.Vector3(x, y, z)。
要将方块 A 放置在地面中心,我们使用了 x = 0,y = 方块高度的一半,即 0.5 和 z = 0。
boxa.position = new BABYLON.Vector3(0,0.5,0);
下一个方块 - 方块 b 放置在 x 轴方向;在 x 方向上的值为 3。
boxb.position = new BABYLON.Vector3(3,0.5,0);
boxc 放置在 x 方向的对面;x 的值为 -3。
boxc.position = new BABYLON.Vector3(-3,0.5,0);
boxd 沿 z 轴放置,如果放置在 z 轴的相反方向,则值为 3 和 -3。
boxd.position = new BABYLON.Vector3(0,0.5,3); boxe.position = new BABYLON.Vector3(0,0.5,-3);
使用球和地面进行演示
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Ball/Ground Demo</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3( .5, .5, .5); var camera = new BABYLON.ArcRotateCamera("camera1", 0, 0, 0, new BABYLON.Vector3(0, 0, 0), scene); camera.setPosition(new BABYLON.Vector3(-100, 0, -100)); camera.attachControl(canvas, true); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(1, 0.5, 0), scene); var pl = new BABYLON.PointLight("pl", new BABYLON.Vector3(0, 0, 0), scene); var gmat = new BABYLON.StandardMaterial("mat1", scene); gmat.alpha = 1.0; var texture = new BABYLON.Texture("images/mat.jpg", scene); gmat.diffuseTexture = texture; var ground = BABYLON.MeshBuilder.CreateGround("ground", {width: 150, height:15}, scene); ground.material = gmat; var mat = new BABYLON.StandardMaterial("mat1", scene); mat.alpha = 1.0; mat.diffuseColor = new BABYLON.Color3(1, 0, 0); var texture = new BABYLON.Texture("images/rugby.jpg", scene); mat.diffuseTexture = texture; var sphere = BABYLON.MeshBuilder.CreateSphere("sphere", {diameter: 5, diameterX:5}, scene); sphere.position= new BABYLON.Vector3(-75,2.5,0); sphere.material = mat; console.log(sphere.position.x); scene.registerBeforeRender(function () { if (sphere.position.x <=75) { console.log(sphere.position.x); if (sphere.position.x <= -75) sphere.position.x=75; sphere.position.x -= 0.25; } else if (sphere.position.x <= -15) { console.log('B'); sphere.position.x += 1; } }); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
输出
以上代码行将生成以下输出−
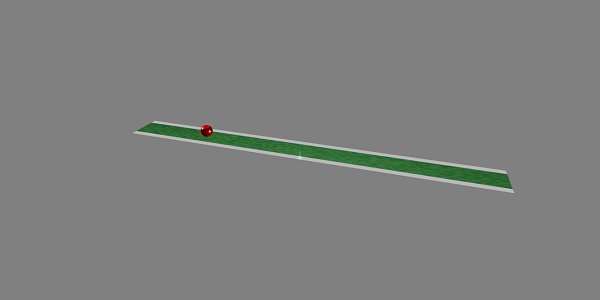
在此演示中,我们使用了两个图像 - mat.jpg 和 rugby.jpg。这些图像存储在本地 images/ 文件夹中,并贴在下面供参考。您可以在演示链接中下载您选择的任何图像并使用它。
用于地面的纹理 − images/mat.jpg
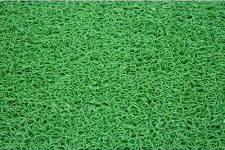
用于球体的纹理 − images/rugby.jpg
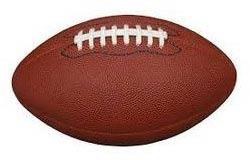
babylonjs_basic_elements.htm
广告