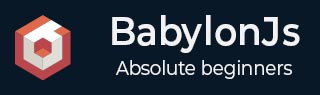
- BabylonJS 教程
- BabylonJS - 首页
- BabylonJS - 简介
- BabylonJS - 环境设置
- BabylonJS - 概述
- BabylonJS - 基本元素
- BabylonJS - 材质
- BabylonJS - 动画
- BabylonJS - 相机
- BabylonJS - 光照
- BabylonJS - 参数化形状
- BabylonJS - 网格(Mesh)
- 矢量位置和旋转
- BabylonJS - 贴花(Decals)
- BabylonJS - 三维曲线(Curve3)
- BabylonJS - 动态纹理
- BabylonJS - 视差贴图
- BabylonJS - 镜头光晕
- BabylonJS - 创建屏幕截图
- BabylonJS - 反射探针
- 标准渲染管线
- BabylonJS - 着色器材质(ShaderMaterial)
- BabylonJS - 骨骼和骨架
- BabylonJS - 物理引擎
- BabylonJS - 播放声音和音乐
- BabylonJS 有用资源
- BabylonJS - 快速指南
- BabylonJS - 有用资源
- BabylonJS - 讨论
BabylonJS - 网格实体粒子(Mesh SolidParticles)
实体粒子系统更新在网格上。我们在网格上看到的全部属性都可以在实体粒子中使用。
在下面给出的演示中,我们创建了标准材质并将其分配给盒子和球体。
要创建实体粒子系统,请执行以下命令:
var SPS = new BABYLON.SolidParticleSystem('SPS', scene); SPS.addShape(sphere, 500); SPS.addShape(box, 500); var mesh = SPS.buildMesh();
要向系统添加粒子,请使用 addShape 方法。它接受诸如形状(即要添加的网格)和数量之类的参数。
在演示链接中,我们将添加球体和盒子。计数为 500,这意味着 500 个球体和盒子。
sphere.dispose(); // free memory box.dispose();
dispose() 方法有助于释放内存,如上所示。
粒子属性
现在让我们看看粒子属性是如何工作的:
var speed = 1.5; var gravity = -0.01;
我们在演示中对粒子系统使用了以下方法:
initParticles - 此方法有助于初始化粒子。SPS.nbParticles 提供所有可用的粒子。
recycleParticle - 你可以使用此方法回收粒子。它包含单个粒子的详细信息。
updateParticle - 允许更新粒子属性。
试用提供的演示,你可以更改属性并查看输出。
演示
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3( .1, .2, .4); var camera = new BABYLON.ArcRotateCamera("camera1", 0, 0, 0, new BABYLON.Vector3(0, 0, -0), scene); camera.setPosition(new BABYLON.Vector3(0, 50, -300)); camera.attachControl(canvas, true); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(1, 1, 0), scene); light.intensity = 0.9; var pl = new BABYLON.PointLight("pl", new BABYLON.Vector3(0, 0, 0), scene); pl.diffuse = new BABYLON.Color3(1, 1, 1); pl.specular = new BABYLON.Color3(0.2, 0.2, 0.8); pl.intensity = 0.75; // texture and material var url = "images/gem1.jpg"; var mat = new BABYLON.StandardMaterial("mat1", scene); var texture = new BABYLON.Texture(url, scene); mat.diffuseTexture = texture; // SPS creation var sphere = BABYLON.Mesh.CreateSphere("sphere", 32, 2, scene); var box = BABYLON.MeshBuilder.CreateBox("box", { size: 2 }, scene); var SPS = new BABYLON.SolidParticleSystem('SPS', scene); SPS.addShape(sphere, 500); SPS.addShape(box, 500); var mesh = SPS.buildMesh(); mesh.material = mat; mesh.position.y = -50; sphere.dispose(); // free memory box.dispose(); // SPS behavior definition var speed = 1.5; var gravity = -0.01; // init SPS.initParticles = function() { // just recycle everything for (var p = 0; p < this.nbParticles; p++) { this.recycleParticle(this.particles[p]); } }; // recycle SPS.recycleParticle = function(particle) { particle.position.x = 0; particle.position.y = 0; particle.position.z = 0; particle.velocity.x = (Math.random() - 0.5) * speed; particle.velocity.y = Math.random() * speed; particle.velocity.z = (Math.random() - 0.5) * speed; var scale = Math.random() +0.5; particle.scale.x = scale; particle.scale.y = scale; particle.scale.z = scale; particle.rotation.x = Math.random() * 3.5; particle.rotation.y = Math.random() * 3.5; particle.rotation.z = Math.random() * 3.5; particle.color.r = Math.random() * 0.6 + 0.5; particle.color.g = Math.random() * 0.6 + 0.5; particle.color.b = Math.random() * 0.6 + 0.5; particle.color.a = Math.random() * 0.6 + 0.5; }; // update : will be called by setParticles() SPS.updateParticle = function(particle) { // some physics here if (particle.position.y < 0) { this.recycleParticle(particle); } particle.velocity.y += gravity; // apply gravity to y (particle.position).addInPlace(particle.velocity); // update particle new position particle.position.y += speed / 2; var sign = (particle.idx % 2 == 0) ? 1 : -1; // rotation sign and new value particle.rotation.z += 0.1 * sign; particle.rotation.x += 0.05 * sign; particle.rotation.y += 0.008 * sign; }; // init all particle values and set them once to apply textures, colors, etc SPS.initParticles(); SPS.setParticles(); // Tuning : SPS.computeParticleColor = false; SPS.computeParticleTexture = false; //scene.debugLayer.show(); // animation scene.registerBeforeRender(function() { SPS.setParticles(); pl.position = camera.position; SPS.mesh.rotation.y += 0.01; }); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
输出
以上代码行生成以下输出:
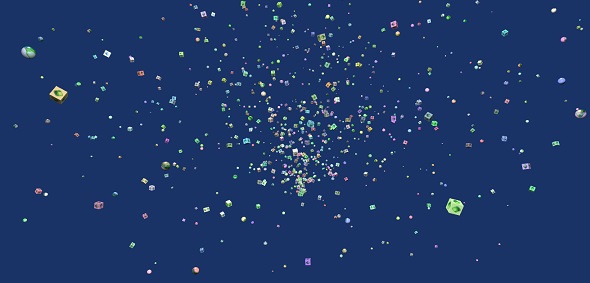
在此演示中,我们使用了图像gem1.jpg。这些图像是存储在本地 images/ 文件夹中,并且也粘贴在下面以供参考。你可以下载任何你选择的图像并在演示链接中使用。
images/gem1.jpg
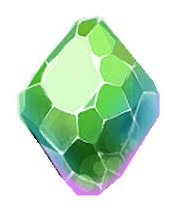
babylonjs_mesh.htm
广告