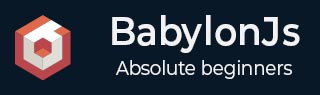
- BabylonJS 教程
- BabylonJS - 首页
- BabylonJS - 简介
- BabylonJS - 环境设置
- BabylonJS - 概述
- BabylonJS - 基本元素
- BabylonJS - 材质
- BabylonJS - 动画
- BabylonJS - 相机
- BabylonJS - 光照
- BabylonJS - 参数化形状
- BabylonJS - 网格
- 矢量位置和旋转
- BabylonJS - 贴花
- BabylonJS - Curve3
- BabylonJS - 动态纹理
- BabylonJS - 视差贴图
- BabylonJS - 镜头光晕
- BabylonJS - 创建屏幕截图
- BabylonJS - 反射探针
- 标准渲染管线
- BabylonJS - ShaderMaterial
- BabylonJS - 骨骼和骨架
- BabylonJS - 物理引擎
- BabylonJS - 播放声音和音乐
- BabylonJS 有用资源
- BabylonJS - 快速指南
- BabylonJS - 有用资源
- BabylonJS - 讨论
BabylonJS - 拉伸
拉伸有助于将二维形状转换为三维体积形状。假设你想创建一个二维星形,它将具有x、y坐标,而z坐标为0。利用二维坐标进行拉伸将将其转换为三维形状。因此,二维星形通过拉伸将变成三维形状。你可以尝试不同的二维形状并将它们转换为三维形状。
语法
BABYLON.Mesh.ExtrudeShape(name, shape, path, scale, rotation, cap, scene, updatable?, sideOrientation)
参数
考虑以下拉伸参数:
名称 - 网格名称。
形状 - 要拉伸的形状;它是一个向量数组。
路径 - 拉伸形状的路径。用于绘制形状的向量数组。
缩放 - 默认值为1。缩放是缩放初始形状的值。
旋转 - 在每个路径点旋转形状。
封盖 - BABYLON.Mesh.NO_CAP, BABYLON.Mesh.CAP_START, BABYLON.Mesh.CAP_END, BABYLON.Mesh.CAP_ALL。
场景 - 将绘制网格的当前场景。
可更新 - 默认值为false。如果设置为true,则网格将可更新。
侧面方向 - 侧面方向 - 正面、背面或双面。
演示 - 使用创建线条
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3( .5, .5, .5); // camera var camera = new BABYLON.ArcRotateCamera("camera1", 0, 0, 0, new BABYLON.Vector3(0, 0, -0), scene); camera.setPosition(new BABYLON.Vector3(0, 0, -10)); camera.attachControl(canvas, true); // lights var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(1, 0.5, 0), scene); light.intensity = 0.7; var spot = new BABYLON.SpotLight("spot", new BABYLON.Vector3(25, 15, -10), new BABYLON.Vector3(-1, -0.8, 1), 15, 1, scene); spot.diffuse = new BABYLON.Color3(1, 1, 1); spot.specular = new BABYLON.Color3(0, 0, 0); spot.intensity = 0.8; // shape var shape = [ new BABYLON.Vector3(2, 0, 0), new BABYLON.Vector3(2, 2, 0), new BABYLON.Vector3(1, 2, 0), new BABYLON.Vector3(0, 3, 0), new BABYLON.Vector3(-1, 2, 0), new BABYLON.Vector3(-2, 2, 0), new BABYLON.Vector3(-2, 0, 0), new BABYLON.Vector3(-2, -2, 0), new BABYLON.Vector3(-1, -2, 0), new BABYLON.Vector3(0, -3, 0), new BABYLON.Vector3(1, -2, 0), new BABYLON.Vector3(2, -2, 0), ]; shape.push(shape[0]); var shapeline = BABYLON.Mesh.CreateLines("sl", shape, scene); shapeline.color = BABYLON.Color3.Green(); return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
输出
以上代码行生成以下输出:
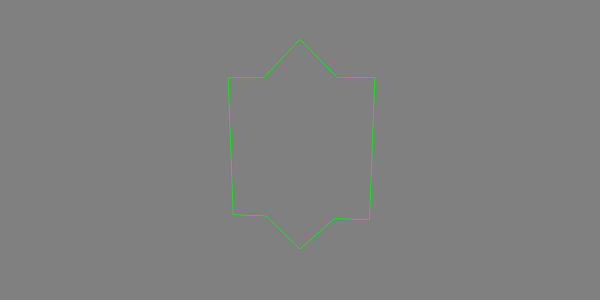
在上面的例子中,线条绘制在x,y坐标系中。现在让我们借助拉伸应用三维效果。为此,babylonjs有一个拉伸类,如下所述。
应用拉伸的演示
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3( .5, .5, .5); // camera var camera = new BABYLON.ArcRotateCamera("camera1", 0, 0, 0, new BABYLON.Vector3(0, 0, -0), scene); camera.setPosition(new BABYLON.Vector3(0, 0, -10)); camera.attachControl(canvas, true); // lights var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(1, 0.5, 0), scene); light.intensity = 0.7; var spot = new BABYLON.SpotLight("spot", new BABYLON.Vector3(25, 15, -10), new BABYLON.Vector3(-1, -0.8, 1), 15, 1, scene); spot.diffuse = new BABYLON.Color3(1, 1, 1); spot.specular = new BABYLON.Color3(0, 0, 0); spot.intensity = 0.8; var mat = new BABYLON.StandardMaterial("mat1", scene); mat.alpha = 1.0; mat.diffuseColor = new BABYLON.Color3(0.5, 0.5, 1.0); mat.backFaceCulling = false; // shape var shape = [ new BABYLON.Vector3(2, 0, 0), new BABYLON.Vector3(2, 2, 0), new BABYLON.Vector3(1, 2, 0), new BABYLON.Vector3(0, 3, 0), new BABYLON.Vector3(-1, 2, 0), new BABYLON.Vector3(-2, 2, 0), new BABYLON.Vector3(-2, 0, 0), new BABYLON.Vector3(-2, -2, 0), new BABYLON.Vector3(-1, -2, 0), new BABYLON.Vector3(0, -3, 0), new BABYLON.Vector3(1, -2, 0), new BABYLON.Vector3(2, -2, 0), ]; shape.push(shape[0]); var path = [ BABYLON.Vector3.Zero(), new BABYLON.Vector3(0, 0, -1) ]; var shapeline = BABYLON.Mesh.CreateLines("sl", shape, scene); shapeline.color = BABYLON.Color3.Green(); var extruded = BABYLON.Mesh.ExtrudeShape("extruded", shape, path, 1, 0, 0, scene); extruded.material = mat; return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
输出
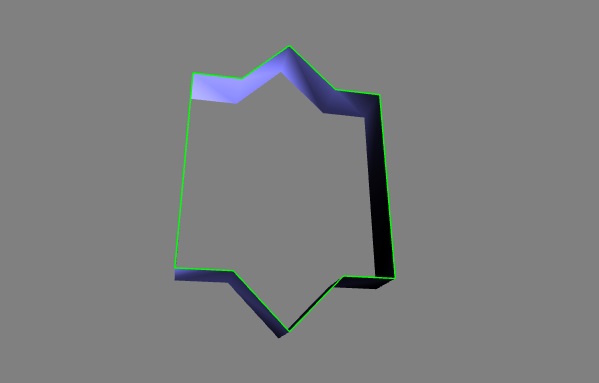
PolygonMeshBuilder演示
PolygonMeshBuilder使用earcut结构,为了使其正常工作,我们需要一个额外的文件,可以从cdn (https://unpkg.com/[email protected]/dist/earcut.min.js) 或npm包(https://github.com/mapbox/earcut#install)获取。
<!doctype html> <html> <head> <meta charset = "utf-8"> <title>BabylonJs - Basic Element-Creating Scene</title> <script src="https://unpkg.com/[email protected]/dist/earcut.min.js"></script> <script src = "babylon.js"></script> <style> canvas {width: 100%; height: 100%;} </style> </head> <body> <canvas id = "renderCanvas"></canvas> <script type = "text/javascript"> var canvas = document.getElementById("renderCanvas"); var engine = new BABYLON.Engine(canvas, true); var createScene = function() { var scene = new BABYLON.Scene(engine); scene.clearColor = new BABYLON.Color3(0, 0, 1); var camera = new BABYLON.ArcRotateCamera("Camera", -Math.PI/2, Math.PI/4, 25, BABYLON.Vector3.Zero(), scene); camera.attachControl(canvas, true); var light = new BABYLON.HemisphericLight("light1", new BABYLON.Vector3(0, 10, 0), scene); light.intensity = 0.5; var corners = [ new BABYLON.Vector2(4, 0), new BABYLON.Vector2(3, 1), new BABYLON.Vector2(2, 3), new BABYLON.Vector2(2, 4), new BABYLON.Vector2(1, 3), new BABYLON.Vector2(0, 3), new BABYLON.Vector2(-1, 3), new BABYLON.Vector2(-3, 4), new BABYLON.Vector2(-2, 2), new BABYLON.Vector2(-3, 0), new BABYLON.Vector2(-3, -2), new BABYLON.Vector2(-3, -3), new BABYLON.Vector2(-2, -2), new BABYLON.Vector2(0, -2), new BABYLON.Vector2(3, -2), new BABYLON.Vector2(3, -1), ]; var hole = [ new BABYLON.Vector2(1, -1), new BABYLON.Vector2(1.5, 0), new BABYLON.Vector2(1.4, 1), new BABYLON.Vector2(0.5, 1.5) ] var poly_tri = new BABYLON.PolygonMeshBuilder("polytri", corners, scene); poly_tri.addHole(hole); var polygon = poly_tri.build(null, 0.5); polygon.position.y = + 4; var poly_path = new BABYLON.Path2(2, 0); poly_path.addLineTo(5, 2); poly_path.addLineTo(1, 2); poly_path.addLineTo(-5, 5); poly_path.addLineTo(-3, 1); poly_path.addLineTo(-4, -4); poly_path.addArcTo(0, -2, 4, -4, 100); var poly_tri2 = new BABYLON.PolygonMeshBuilder("polytri2", poly_path, scene); poly_tri2.addHole(hole); var polygon2 = poly_tri2.build(false, 0.5); //updatable, extrusion depth - both optional polygon2.position.y = -4; return scene; }; var scene = createScene(); engine.runRenderLoop(function() { scene.render(); }); </script> </body> </html>
输出
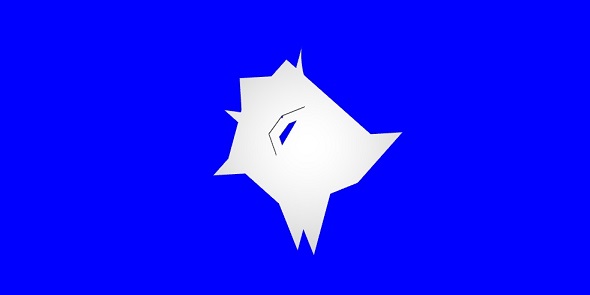
语法
以下是PolygonMeshBuilder的语法:
var poly_tri2 = new BABYLON.PolygonMeshBuilder("polytri2", poly_path, scene);
babylonjs_parametric_shapes.htm
广告