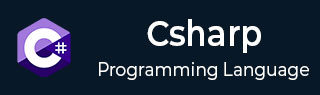
- C# 基础教程
- C# - 首页
- C# - 概述
- C# - 环境
- C# - 程序结构
- C# - 基本语法
- C# - 数据类型
- C# - 类型转换
- C# - 变量
- C# - 常量
- C# - 运算符
- C# - 决策
- C# - 循环
- C# - 封装
- C# - 方法
- C# - 可空类型
- C# - 数组
- C# - 字符串
- C# - 结构体
- C# - 枚举
- C# - 类
- C# - 继承
- C# - 多态
- C# - 运算符重载
- C# - 接口
- C# - 命名空间
- C# - 预处理器指令
- C# - 正则表达式
- C# - 异常处理
- C# - 文件I/O
C# - 输出参数
return语句只能返回函数的一个值。但是,使用**输出参数**,可以从函数返回两个值。输出参数类似于引用参数,不同之处在于它们将数据从方法传递出去,而不是传入方法。
下面的例子说明了这一点:
using System; namespace CalculatorApplication { class NumberManipulator { public void getValue(out int x ) { int temp = 5; x = temp; } static void Main(string[] args) { NumberManipulator n = new NumberManipulator(); /* local variable definition */ int a = 100; Console.WriteLine("Before method call, value of a : {0}", a); /* calling a function to get the value */ n.getValue(out a); Console.WriteLine("After method call, value of a : {0}", a); Console.ReadLine(); } } }
编译并运行上述代码后,将产生以下结果:
Before method call, value of a : 100 After method call, value of a : 5
为输出参数提供的变量不需要赋值。当需要通过参数从方法返回值,而无需为参数赋值初始值时,输出参数特别有用。请看下面的例子,了解这一点:
using System; namespace CalculatorApplication { class NumberManipulator { public void getValues(out int x, out int y ) { Console.WriteLine("Enter the first value: "); x = Convert.ToInt32(Console.ReadLine()); Console.WriteLine("Enter the second value: "); y = Convert.ToInt32(Console.ReadLine()); } static void Main(string[] args) { NumberManipulator n = new NumberManipulator(); /* local variable definition */ int a , b; /* calling a function to get the values */ n.getValues(out a, out b); Console.WriteLine("After method call, value of a : {0}", a); Console.WriteLine("After method call, value of b : {0}", b); Console.ReadLine(); } } }
编译并运行上述代码后,将产生以下结果:
Enter the first value: 7 Enter the second value: 8 After method call, value of a : 7 After method call, value of b : 8
csharp_methods.htm
广告