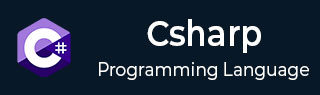
- C# 基础教程
- C# - 首页
- C# - 概述
- C# - 环境
- C# - 程序结构
- C# - 基本语法
- C# - 数据类型
- C# - 类型转换
- C# - 变量
- C# - 常量
- C# - 运算符
- C# - 决策制定
- C# - 循环
- C# - 封装
- C# - 方法
- C# - 可空类型
- C# - 数组
- C# - 字符串
- C# - 结构体
- C# - 枚举
- C# - 类
- C# - 继承
- C# - 多态
- C# - 运算符重载
- C# - 接口
- C# - 命名空间
- C# - 预处理器指令
- C# - 正则表达式
- C# - 异常处理
- C# - 文件 I/O
- C# 高级教程
- C# - 属性
- C# - 反射
- C# - 属性
- C# - 索引器
- C# - 委托
- C# - 事件
- C# - 集合
- C# - 泛型
- C# - 匿名方法
- C# - 不安全代码
- C# - 多线程
- C# 有用资源
- C# - 问题与解答
- C# - 快速指南
- C# - 有用资源
- C# - 讨论
C# - 按引用传递参数
引用参数是变量的内存位置的引用。当您按引用传递参数时,与值参数不同,不会为这些参数创建新的存储位置。引用参数表示与传递给方法的实际参数相同的内存位置。
您可以使用ref关键字声明引用参数。以下示例演示了这一点:
using System; namespace CalculatorApplication { class NumberManipulator { public void swap(ref int x, ref int y) { int temp; temp = x; /* save the value of x */ x = y; /* put y into x */ y = temp; /* put temp into y */ } static void Main(string[] args) { NumberManipulator n = new NumberManipulator(); /* local variable definition */ int a = 100; int b = 200; Console.WriteLine("Before swap, value of a : {0}", a); Console.WriteLine("Before swap, value of b : {0}", b); /* calling a function to swap the values */ n.swap(ref a, ref b); Console.WriteLine("After swap, value of a : {0}", a); Console.WriteLine("After swap, value of b : {0}", b); Console.ReadLine(); } } }
当以上代码编译并执行时,会产生以下结果:
Before swap, value of a : 100 Before swap, value of b : 200 After swap, value of a : 200 After swap, value of b : 100
它表明值已在swap函数内部更改,并且此更改反映在Main函数中。
csharp_methods.htm
广告