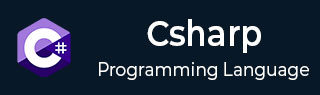
- C# 基础教程
- C# - 首页
- C# - 概述
- C# - 环境
- C# - 程序结构
- C# - 基本语法
- C# - 数据类型
- C# - 类型转换
- C# - 变量
- C# - 常量
- C# - 运算符
- C# - 决策制定
- C# - 循环
- C# - 封装
- C# - 方法
- C# - 可空类型
- C# - 数组
- C# - 字符串
- C# - 结构体
- C# - 枚举
- C# - 类
- C# - 继承
- C# - 多态
- C# - 运算符重载
- C# - 接口
- C# - 命名空间
- C# - 预处理器指令
- C# - 正则表达式
- C# - 异常处理
- C# - 文件 I/O
- C# 高级教程
- C# - 属性
- C# - 反射
- C# - 属性
- C# - 索引器
- C# - 委托
- C# - 事件
- C# - 集合
- C# - 泛型
- C# - 匿名方法
- C# - 不安全代码
- C# - 多线程
- C# 有用资源
- C# - 问题和解答
- C# - 快速指南
- C# - 有用资源
- C# - 讨论
C# - SortedList 类
SortedList 类表示一个键值对的集合,这些键值对按键排序,并且可以通过键和索引访问。
排序列表是数组和哈希表的一个组合。它包含一个项目列表,可以使用键或索引访问。如果使用索引访问项目,则为 ArrayList,如果使用键访问项目,则为 Hashtable。项目的集合始终按键值排序。
SortedList 类的属性和方法
下表列出了 SortedList 类的一些常用属性:
序号 | 属性及描述 |
---|---|
1 | Capacity 获取或设置 SortedList 的容量。 |
2 | Count 获取 SortedList 中包含的元素数。 |
3 | IsFixedSize 获取一个值,该值指示 SortedList 是否具有固定大小。 |
4 | IsReadOnly 获取一个值,该值指示 SortedList 是否为只读。 |
5 | Item 获取和设置与 SortedList 中特定键关联的值。 |
6 | Keys 获取 SortedList 中的键。 |
7 | Values 获取 SortedList 中的值。 |
下表列出了 SortedList 类的一些常用方法:
序号 | 方法及描述 |
---|---|
1 | public virtual void Add(object key, object value); 将具有指定键和值的元素添加到 SortedList 中。 |
2 | public virtual void Clear(); 移除 SortedList 中的所有元素。 |
3 | public virtual bool ContainsKey(object key); 确定 SortedList 是否包含特定键。 |
4 | public virtual bool ContainsValue(object value); 确定 SortedList 是否包含特定值。 |
5 | public virtual object GetByIndex(int index); 获取 SortedList 中指定索引处的值。 |
6 | public virtual object GetKey(int index); 获取 SortedList 中指定索引处的键。 |
7 | public virtual IList GetKeyList(); 获取 SortedList 中的键。 |
8 | public virtual IList GetValueList(); 获取 SortedList 中的值。 |
9 | public virtual int IndexOfKey(object key); 返回 SortedList 中指定键的基于零的索引。 |
10 | public virtual int IndexOfValue(object value); 返回 SortedList 中指定值的第一次出现的基于零的索引。 |
11 | public virtual void Remove(object key); 从 SortedList 中移除具有指定键的元素。 |
12 | public virtual void RemoveAt(int index); 移除 SortedList 中指定索引处的元素。 |
13 | public virtual void TrimToSize(); 将容量设置为 SortedList 中元素的实际数量。 |
示例
以下示例演示了该概念:
using System; using System.Collections; namespace CollectionsApplication { class Program { static void Main(string[] args) { SortedList sl = new SortedList(); sl.Add("001", "Zara Ali"); sl.Add("002", "Abida Rehman"); sl.Add("003", "Joe Holzner"); sl.Add("004", "Mausam Benazir Nur"); sl.Add("005", "M. Amlan"); sl.Add("006", "M. Arif"); sl.Add("007", "Ritesh Saikia"); if (sl.ContainsValue("Nuha Ali")) { Console.WriteLine("This student name is already in the list"); } else { sl.Add("008", "Nuha Ali"); } // get a collection of the keys. ICollection key = sl.Keys; foreach (string k in key) { Console.WriteLine(k + ": " + sl[k]); } } } }
编译并执行上述代码后,将产生以下结果:
001: Zara Ali 002: Abida Rehman 003: Joe Holzner 004: Mausam Banazir Nur 005: M. Amlan 006: M. Arif 007: Ritesh Saikia 008: Nuha Ali