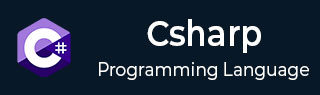
- C# 基础教程
- C# - 首页
- C# - 概述
- C# - 环境
- C# - 程序结构
- C# - 基本语法
- C# - 数据类型
- C# - 类型转换
- C# - 变量
- C# - 常量
- C# - 运算符
- C# - 决策制定
- C# - 循环
- C# - 封装
- C# - 方法
- C# - 可空类型
- C# - 数组
- C# - 字符串
- C# - 结构体
- C# - 枚举
- C# - 类
- C# - 继承
- C# - 多态
- C# - 运算符重载
- C# - 接口
- C# - 命名空间
- C# - 预处理器指令
- C# - 正则表达式
- C# - 异常处理
- C# - 文件 I/O
C# - 读写文本文件
StreamReader 和 StreamWriter 类用于读写文本文件中的数据。这些类继承自抽象基类 Stream,该基类支持将字节读写到文件流中。
StreamReader 类
StreamReader 类也继承自抽象基类 TextReader,该基类表示用于读取一系列字符的读取器。下表描述了 StreamReader 类的一些常用方法:
序号 | 方法和描述 |
---|---|
1 | public override void Close() 它关闭 StreamReader 对象和底层流,并释放与读取器关联的任何系统资源。 |
2 | public override int Peek() 返回下一个可用字符,但不读取它。 |
3 | public override int Read() 从输入流读取下一个字符,并将字符位置前进一位。 |
示例
以下示例演示如何读取名为 Jamaica.txt 的文本文件。文件内容为:
Down the way where the nights are gay And the sun shines daily on the mountain top I took a trip on a sailing ship And when I reached Jamaica I made a stop
using System; using System.IO; namespace FileApplication { class Program { static void Main(string[] args) { try { // Create an instance of StreamReader to read from a file. // The using statement also closes the StreamReader. using (StreamReader sr = new StreamReader("c:/jamaica.txt")) { string line; // Read and display lines from the file until // the end of the file is reached. while ((line = sr.ReadLine()) != null) { Console.WriteLine(line); } } } catch (Exception e) { // Let the user know what went wrong. Console.WriteLine("The file could not be read:"); Console.WriteLine(e.Message); } Console.ReadKey(); } } }
猜猜编译并运行程序后它会显示什么!
StreamWriter 类
StreamWriter 类继承自抽象类 TextWriter,该类表示一个写入器,可以写入一系列字符。
下表描述了此类最常用的方法:
序号 | 方法和描述 |
---|---|
1 | public override void Close() 关闭当前 StreamWriter 对象和底层流。 |
2 | public override void Flush() 清除当前写入器的所有缓冲区,并将任何缓冲数据写入底层流。 |
3 | public virtual void Write(bool value) 将布尔值的文本表示形式写入文本字符串或流。(继承自 TextWriter。) |
4 | public override void Write(char value) 将字符写入流。 |
5 | public virtual void Write(decimal value) 将十进制值的文本表示形式写入文本字符串或流。 |
6 | public virtual void Write(double value) 将 8 字节浮点值的文本表示形式写入文本字符串或流。 |
7 | public virtual void Write(int value) 将 4 字节有符号整数的文本表示形式写入文本字符串或流。 |
8 | public override void Write(string value) 将字符串写入流。 |
9 | public virtual void WriteLine() 将行终止符写入文本字符串或流。 |
有关方法的完整列表,请访问 Microsoft 的 C# 文档。
示例
以下示例演示如何使用 StreamWriter 类将文本数据写入文件:
using System; using System.IO; namespace FileApplication { class Program { static void Main(string[] args) { string[] names = new string[] {"Zara Ali", "Nuha Ali"}; using (StreamWriter sw = new StreamWriter("names.txt")) { foreach (string s in names) { sw.WriteLine(s); } } // Read and show each line from the file. string line = ""; using (StreamReader sr = new StreamReader("names.txt")) { while ((line = sr.ReadLine()) != null) { Console.WriteLine(line); } } Console.ReadKey(); } } }
编译并执行上述代码后,将产生以下结果:
Zara Ali Nuha Ali