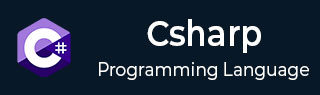
- C# 基础教程
- C# - 首页
- C# - 概述
- C# - 环境
- C# - 程序结构
- C# - 基本语法
- C# - 数据类型
- C# - 类型转换
- C# - 变量
- C# - 常量
- C# - 运算符
- C# - 决策
- C# - 循环
- C# - 封装
- C# - 方法
- C# - 可空类型
- C# - 数组
- C# - 字符串
- C# - 结构体
- C# - 枚举
- C# - 类
- C# - 继承
- C# - 多态
- C# - 运算符重载
- C# - 接口
- C# - 命名空间
- C# - 预处理器指令
- C# - 正则表达式
- C# - 异常处理
- C# - 文件 I/O
C# - if...else 语句
一个if语句可以后跟一个可选的else语句,当布尔表达式为假时执行。
语法
C#中if...else语句的语法为:
if(boolean_expression) { /* statement(s) will execute if the boolean expression is true */ } else { /* statement(s) will execute if the boolean expression is false */ }
如果布尔表达式计算结果为true,则执行if块代码,否则执行else块代码。
流程图
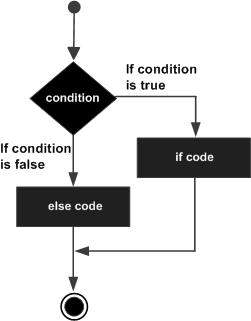
示例
using System; namespace DecisionMaking { class Program { static void Main(string[] args) { /* local variable definition */ int a = 100; /* check the boolean condition */ if (a < 20) { /* if condition is true then print the following */ Console.WriteLine("a is less than 20"); } else { /* if condition is false then print the following */ Console.WriteLine("a is not less than 20"); } Console.WriteLine("value of a is : {0}", a); Console.ReadLine(); } } }
编译并执行上述代码后,将产生以下结果:
a is not less than 20; value of a is : 100
if...else if...else 语句
一个if语句可以后跟一个可选的else if...else语句,这对于使用单个if...else if语句测试各种条件非常有用。
使用if、else if、else语句时,需要注意以下几点。
一个if语句可以有零个或一个else语句,并且它必须位于任何else if语句之后。
一个if语句可以有零个或多个else if语句,并且它们必须位于else语句之前。
一旦else if语句成功,就不会测试任何剩余的else if语句或else语句。
语法
C#中if...else if...else语句的语法为:
if(boolean_expression 1) { /* Executes when the boolean expression 1 is true */ } else if( boolean_expression 2) { /* Executes when the boolean expression 2 is true */ } else if( boolean_expression 3) { /* Executes when the boolean expression 3 is true */ } else { /* executes when the none of the above condition is true */ }
示例
using System; namespace DecisionMaking { class Program { static void Main(string[] args) { /* local variable definition */ int a = 100; /* check the boolean condition */ if (a == 10) { /* if condition is true then print the following */ Console.WriteLine("Value of a is 10"); } else if (a == 20) { /* if else if condition is true */ Console.WriteLine("Value of a is 20"); } else if (a == 30) { /* if else if condition is true */ Console.WriteLine("Value of a is 30"); } else { /* if none of the conditions is true */ Console.WriteLine("None of the values is matching"); } Console.WriteLine("Exact value of a is: {0}", a); Console.ReadLine(); } } }
编译并执行上述代码后,将产生以下结果:
None of the values is matching Exact value of a is: 100
csharp_decision_making.htm
广告