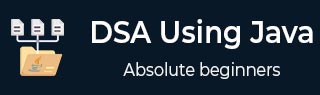
- 使用 Java 的 DSA 教程
- 使用 Java 进行 DSA - 主页
- 使用 Java 进行 DSA - 概览
- 使用 Java 进行 DSA - 环境设置
- 使用 Java 进行 DSA - 算法
- 使用 Java 进行 DSA - 数据结构
- 使用 Java 进行 DSA - 数组
- 使用 Java 进行 DSA - 链表
- 使用 Java 进行 DSA - 双向链表
- 使用 Java 进行 DSA - 循环链表
- 使用 Java 进行 DSA - 堆栈
- DSA - 解析表达式
- 使用 Java 进行 DSA - 队列
- 使用 Java 进行 DSA - 优先级队列
- 使用 Java 进行 DSA - 树
- 使用 Java 进行 DSA - 哈希表
- 使用 Java 进行 DSA - 堆
- 使用 Java 进行 DSA - 图
- 使用 Java 进行 DSA - 搜索技术
- 使用 Java 进行 DSA - 排序技术
- 使用 Java 进行 DSA - 递归
- 使用 Java 进行 DSA 的有用资源
- 使用 Java 进行 DSA - 快速指南
- 使用 Java 进行 DSA - 有用资源
- 使用 Java 进行 DSA - 讨论
使用 Java 进行 DSA - 二分搜索
概览
二分搜索是一种非常快速的搜索算法。此搜索算法遵循分而治之的原理。为了正确执行此算法,数据收集应以排序形式。
二分搜索通过比较集合的中间项搜索特定项。如果匹配,则返回该项的索引。如果中间项大于项,则在中间项右边的子数组中搜索该项,否则在中间项左边的子数组中搜索该项。此过程也将继续进行子数组,直到子数组的大小减小到零。
二分搜索将可搜索项减半,从而将所需的比较次数减少到非常少。
算法
Binary Search ( A: array of item, n: total no. of items ,x: item to be searched) Step 1: Set lowerBound = 1 Step 2: Set upperBound = n Step 3: if upperBound < lowerBound go to step 12 Step 4: set midPoint = ( lowerBound + upperBound ) / 2 Step 5: if A[midPoint] < x Step 6: set lowerBound = midPoint + 1 Step 7: if A[midPoint] > x Step 8: set upperBound = midPoint - 1 Step 9 if A[midPoint] = x go to step 11 Step 10: Go to Step 3 Step 11: Print Element x Found at index midPoint and go to step 13 Step 12: Print element not found Step 13: Exit
演示程序
package com.tutorialspoint.simplesearch; import java.util.Arrays; public class BinarySearchDemo { public static void main(String args[]){ int[] sourceArray = {1,2,3,4,6,7,9,11,12,14,15, 16,17,19,33,34,43,45,55,66,76,88}; System.out.println("Input Array: " +Arrays.toString(sourceArray)); printline(50); // find location of 55 int location = find(sourceArray, 55); if(location != -1){ System.out.println("Element found at location: " +(location+1)); }else { System.out.println("Element not found."); } } public static int find(int[] intArray, int data){ int lowerBound = 0; int upperBound = intArray.length -1; int midPoint = -1; int comparisons = 0; int index = -1; while(lowerBound <= upperBound){ System.out.println("Comparison " + (comparisons +1) ) ; System.out.println("lowerBound : "+lowerBound + " , intArray[" + lowerBound+"] = " + intArray[lowerBound]) ; System.out.println("upperBound : "+upperBound + " , intArray[" + upperBound+"] = " + intArray[upperBound]) ; comparisons++; // compute the mid point midPoint = (lowerBound + upperBound) / 2; // data found if(intArray[midPoint] == data){ index = midPoint; break; } else { // if data is larger if(intArray[midPoint] < data){ // data is in upper half lowerBound = midPoint + 1; } // data is smaller else{ // data is in lower half upperBound = midPoint -1; } } } System.out.println("Total comparisons made: " + comparisons); return index; } public static void printline(int count){ for(int i=0;i <count-1;i++){ System.out.print("="); } System.out.println("="); } }
如果我们编译并运行上述程序,它将产生以下结果
Input Array: [1, 2, 3, 4, 6, 7, 9, 11, 12, 14, 15, 16, 17, 19, 33, 34, 43, 45, 55, 66, 76, 88] ================================================== Comparison 1 lowerBound : 0 , intArray[0] = 1 upperBound : 21 , intArray[21] = 88 Comparison 2 lowerBound : 11 , intArray[11] = 16 upperBound : 21 , intArray[21] = 88 Comparison 3 lowerBound : 17 , intArray[17] = 45 upperBound : 21 , intArray[21] = 88 Comparison 4 lowerBound : 17 , intArray[17] = 45 upperBound : 18 , intArray[18] = 55 Comparison 5 lowerBound : 18 , intArray[18] = 55 upperBound : 18 , intArray[18] = 55 Total comparisons made: 5 Element found at location: 19
广告