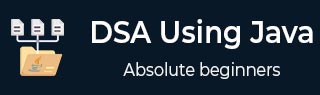
- 使用 Java 的 DSA 教程
- Java 中的 DSA - 主页
- Java 中的 DSA - 概览
- Java 中的 DSA - 环境设置
- Java 中的 DSA - 算法
- Java 中的 DSA - 数据结构
- Java 中的 DSA - 数组
- Java 中的 DSA - 链表
- Java 中的 DSA - 双向链表
- Java 中的 DSA - 循环链表
- Java 中的 DSA - 栈
- DSA - 解析表达式
- Java 中的 DSA - 队列
- Java 中的 DSA - 优先级队列
- Java 中的 DSA - 树
- Java 中的 DSA - 哈希表
- Java 中的 DSA - 堆
- Java 中的 DSA - 图
- Java 中的 DSA - 搜索技术
- Java 中的 DSA - 排序技术
- Java 中的 DSA - 递归
- Java 中的 DSA 有用资源
- Java 中的 DSA - 快速指南
- Java 中的 DSA - 有用资源
- Java 中的 DSA - 讨论
Java 中的 DSA - 线性搜索
概览
线性搜索是一种非常简单的搜索算法。在此类搜索中,对所有项逐个进行顺序搜索。检查每一项,如果找到匹配项,则返回该特定项,否则,搜索将继续到数据收集结束。
算法
Linear Search ( A: array of item, n: total no. of items ,x: item to be searched) Step 1: Set i to 1 Step 2: if i > n then go to step 7 Step 3: if A[i] = x then go to step 6 Step 4: Set i to i + 1 Step 5: Go to Step 2 Step 6: Print Element x Found at index i and go to step 8 Step 7: Print element not found Step 8: Exit
演示程序
package com.tutorialspoint.simplesearch; import java.util.Arrays; public class LinearSearchDemo { public static void main(String args[]){ // array of items on which linear search will be conducted. int[] sourceArray = {1,2,3,4,6,7,9,11,12,14,15, 16,17,19,33,34,43,45,55,66,76,88}; System.out.println("Input Array: " +Arrays.toString(sourceArray)); printline(50); //find location of 1 int location = find(sourceArray, 55); // if element was found if(location != -1) System.out.println("Element found at location: " +(location+1)); else System.out.println("Element not found."); } // this method makes a linear search. public static int find(int[] intArray, int data){ int comparisons = 0; int index= -1; // navigate through all items for(int i=0;i<intArray.length;i++){ // count the comparisons made comparisons++; // if data found, break the loop if(data == intArray[i]){ index = i; break; } } System.out.println("Total comparisons made: " + comparisons); return index; } public static void printline(int count){ for(int i=0;i <count-1;i++){ System.out.print("="); } System.out.println("="); } }
如果我们编译并运行上述程序,它将产生以下结果 −
Input Array: [1, 2, 3, 4, 6, 7, 9, 11, 12, 14, 15, 16, 17, 19, 33, 34, 43, 45, 55, 66, 76, 88] ================================================== Total comparisons made: 19 Element found at location: 19
广告