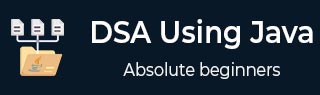
- 使用 Java 的 DSA 教程
- 使用 Java 的 DSA - 首页
- 使用 Java 的 DSA - 概述
- 使用 Java 的 DSA - 环境设置
- 使用 Java 的 DSA - 算法
- 使用 Java 的 DSA - 数据结构
- 使用 Java 的 DSA - 数组
- 使用 Java 的 DSA - 链表
- 使用 Java 的 DSA - 双向链表
- 使用 Java 的 DSA - 循环链表
- 使用 Java 的 DSA - 堆栈
- DSA - 解析表达式
- 使用 Java 的 DSA - 队列
- 使用 Java 的 DSA - 优先级队列
- 使用 Java 的 DSA - 树
- 使用 Java 的 DSA - 哈希表
- 使用 Java 的 DSA - 堆
- 使用 Java 的 DSA - 图
- 使用 Java 的 DSA - 搜索技术
- 使用 Java 的 DSA - 排序技术
- 使用 Java 的 DSA - 递归
- 使用 Java 的 DSA 有用资源
- 使用 Java 的 DSA - 快速指南
- 使用 Java 的 DSA - 有用资源
- 使用 Java 的 DSA - 讨论
使用 Java 的 DSA - 选择排序
概述
选择排序是一种简单的排序算法。此排序算法是一种基于就地比较的算法,其中列表分为两部分,左侧为已排序部分,右侧为未排序部分。最初,已排序部分为空,未排序部分为整个列表。
从未排序数组中选择最小元素,并与最左元素交换,该元素成为已排序数组的一部分。此过程通过将未排序数组边界向右移动一个元素来继续。
此算法不适用于大型数据集,因为其平均和最坏情况下时间复杂度为 O(n2),其中 n 是元素的数量。
伪代码
Selection Sort ( A: array of item) procedure selectionSort( A : array of items ) int indexMin for i = 1 to length(A) - 1 inclusive do: /* set current element as minimum*/ indexMin = i /* check the element to be minimum */ for j = i+1 to length(A) - 1 inclusive do: if(intArray[j] < intArray[indexMin]){ indexMin = j; } end for /* swap the minimum element with the current element*/ if(indexMin != i) then swap(A[indexMin],A[i]) end if end for end procedure
演示程序
package com.tutorialspoint.simplesort; import java.util.Arrays; public class SelectionSortDemo { public static void main(String[] args){ int[] sourceArray = {4,6,3,2,1,9,7}; System.out.println("Input Array: " + Arrays.toString(sourceArray)); printline(50); System.out.println("Output Array: " + Arrays.toString(selectionSort(sourceArray))); printline(50); } public static void printline(int count){ for(int i=0;i <count-1;i++){ System.out.print("="); } System.out.println("="); } public static int[] selectionSort(int[] intArray){ int indexMin; // loop through all numbers for(int i=0; i < intArray.length-1; i++){ // set current element as minimum indexMin = i; // check the element to be minimum for(int j=i+1;j<intArray.length;j++){ if(intArray[j] < intArray[indexMin]){ indexMin = j; } } if(indexMin != i){ System.out.println(" Items swapped: [ " + intArray[i] + ", " + intArray[indexMin] +" ]" ); // swap the numbers int temp=intArray[indexMin]; intArray[indexMin] = intArray[i]; intArray[i] = temp; } System.out.println("iteration "+(i+1) +"#: " + Arrays.toString(intArray)); } return intArray; } }
如果我们编译并运行以上程序,则会产生以下结果 −
Input Array: [4, 6, 3, 2, 1, 9, 7] ================================================== Items swapped: [ 4, 1 ] iteration 1#: [1, 6, 3, 2, 4, 9, 7] Items swapped: [ 6, 2 ] iteration 2#: [1, 2, 3, 6, 4, 9, 7] iteration 3#: [1, 2, 3, 6, 4, 9, 7] Items swapped: [ 6, 4 ] iteration 4#: [1, 2, 3, 4, 6, 9, 7] iteration 5#: [1, 2, 3, 4, 6, 9, 7] Items swapped: [ 9, 7 ] iteration 6#: [1, 2, 3, 4, 6, 7, 9] Output Array: [1, 2, 3, 4, 6, 7, 9] ==================================================
广告