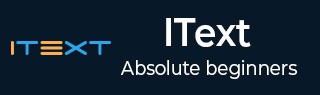
- iText 教程
- iText - 首页
- iText 画布
- iText - 绘制圆弧
- iText - 绘制线条
- iText - 绘制圆形
- iText 有用资源
- iText - 快速指南
- iText - 有用资源
- iText - 讨论
iText - 链接注释
在本章中,我们将了解如何使用 iText 库向 PDF 文档添加链接注释。
在 PDF 中创建链接注释
您可以通过实例化 **Document** 类来创建一个空的 PDF 文档。在实例化此类时,您需要将 **PdfDocument** 对象作为参数传递给其构造函数。
要在您的 PDF 文档中使用文本注释,您需要创建一个 PdfTextAnnotation 类的对象,并将其添加到 PdfPage 中。
以下是 PDF 文档中使用文本注释的步骤。
步骤 1:创建 PdfWriter 对象
**PdfWriter** 类表示 PDF 的 DocWriter。此类属于 **com.itextpdf.kernel.pdf** 包。此类的构造函数接受一个字符串,表示要创建 PDF 文件的路径。
通过将字符串值(表示您需要在其中创建 PDF 的路径)传递给其构造函数来实例化 **PdfWriter** 类,如下所示。
// Creating a PdfWriter String dest = "C:/itextExamples/linkAnnotation.pdf"; PdfWriter writer = new PdfWriter(dest);
当此类型的对象传递给 PdfDocument(类)时,添加到此文档中的每个元素都将写入指定的文件。
步骤 2:创建 PdfDocument 对象
**PdfDocument** 类是表示 iText 中 PDF 文档的类。此类属于 **com.itextpdf.kernel.pdf** 包。要实例化此类(在写入模式下),您需要将 **PdfWriter** 类的对象传递给其构造函数。
通过将 **PdfWriter** 对象传递给其构造函数来实例化 PdfDocument 类,如下所示。
// Creating a PdfDocument PdfDocument pdfDoc = new PdfDocument(writer);
创建 PdfDocument 对象后,您可以使用其类提供的相应方法添加各种元素,例如页面、字体、文件附件和事件处理程序。
步骤 3:创建 Document 对象
**com.itextpdf.layout** 包的 **Document** 类是创建自包含 PDF 时的根元素。此类的一个构造函数接受 **PdfDocument** 类的对象。
通过将上一步中创建的 PdfDocument 类的对象传递给构造函数来实例化 **Document** 类,如下所示。
// Creating a Document Document document = new Document(pdfDoc);
步骤 4:创建 PdfAnnotation 对象
**com.itextpdf.kernel.pdf.annot** 包的 **PdfAnnotation** 类表示所有注释的超类。
在其派生类中,**PdfLinkAnnotation** 类表示链接注释。创建此类的对象,如下所示。
// Creating a PdfLinkAnnotation object Rectangle rect = new Rectangle(0, 0); PdfLinkAnnotation annotation = new PdfLinkAnnotation(rect);
步骤 5:设置注释的操作
使用 **PdfLinkAnnotation** 类的 **setAction()** 方法将操作设置为注释,如下所示。
// Setting action of the annotation PdfAction action = PdfAction.createURI("http: // www.tutorialspoint.com/"); annotation.setAction(action);
步骤 6:创建链接
通过实例化 **com.itextpdf.layout.element** 包的 **Link** 类来创建链接,如下所示。
// Creating a link Link link = new Link("Click here", annotation);
步骤 7:将链接注释添加到段落
通过实例化 **Paragraph** 类创建一个新段落,并使用此类的 **add()** 方法添加上一步中创建的链接,如下所示。
// Creating a paragraph Paragraph paragraph = new Paragraph("Hi welcome to Tutorialspoint "); // Adding link to paragraph paragraph.add(link.setUnderline());
步骤 8:将段落添加到文档
使用 **Document** 类的 **add()** 方法将段落添加到文档,如下所示。
// Adding paragraph to document document.add(paragraph);
步骤 9:关闭文档
使用 **Document** 类的 **close()** 方法关闭文档,如下所示。
// Closing the document document.close();
示例
以下 Java 程序演示了如何使用 iText 库向 PDF 文档添加链接注释。
它创建一个名为 **linkAnnotation.pdf** 的 PDF 文档,向其中添加链接注释,并将其保存在 **C:/itextExamples/** 路径中。
将此代码保存在名为 **LinkAnnotation.java** 的文件中。
import com.itextpdf.kernel.geom.Rectangle; import com.itextpdf.kernel.pdf.PdfDocument; import com.itextpdf.kernel.pdf.PdfWriter; import com.itextpdf.kernel.pdf.action.PdfAction; import com.itextpdf.kernel.pdf.annot.PdfLinkAnnotation; import com.itextpdf.layout.Document; import com.itextpdf.layout.element.Link; import com.itextpdf.layout.element.Paragraph; public class LinkAnnotation { public static void main(String args[]) throws Exception { // Creating a PdfWriter String dest = "C:/itextExamples/linkAnnotation.pdf"; PdfWriter writer = new PdfWriter(dest); // Creating a PdfDocument PdfDocument pdf = new PdfDocument(writer); // Creating a Document Document document = new Document(pdf); // Creating a PdfLinkAnnotation object Rectangle rect = new Rectangle(0, 0); PdfLinkAnnotation annotation = new PdfLinkAnnotation(rect); // Setting action of the annotation PdfAction action = PdfAction.createURI("http:// www.tutorialspoint.com/"); annotation.setAction(action); // Creating a link Link link = new Link("Click here", annotation); // Creating a paragraph Paragraph paragraph = new Paragraph("Hi welcome to Tutorialspoint "); // Adding link to paragraph paragraph.add(link.setUnderline()); // Adding paragraph to document document.add(paragraph); // Closing the document document.close(); System.out.println("Annotation added successfully"); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件:
javac LinkAnnotation.java java LinkAnnotation
执行后,上述程序将创建一个 PDF 文档,显示以下消息。
Annotation added successfully
如果验证指定的路径,则可以找到创建的 PDF 文档,如下所示。
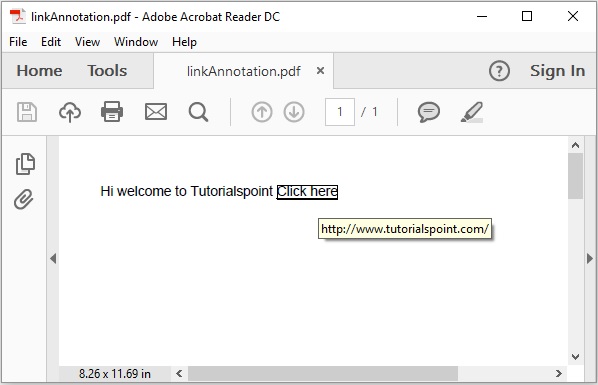