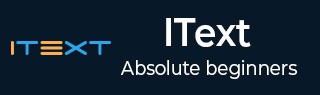
- iText 教程
- iText - 首页
- iText 画布
- iText - 绘制弧线
- iText - 绘制直线
- iText - 绘制圆形
- iText 有用资源
- iText - 快速指南
- iText - 有用资源
- iText - 讨论
iText - 标记注释
在本章中,我们将学习如何使用 iText 库向 PDF 文档添加文本标记注释。
在 PDF 中创建标记注释
您可以通过实例化 **Document** 类来创建一个空的 PDF 文档。在实例化此类时,您需要将 **PdfDocument** 对象作为参数传递给其构造函数。要在 PDF 文档中使用文本注释,您需要创建一个 **PdfTextAnnotation** 类的对象,并将其添加到 **PdfPage** 中。
以下是如何在 PDF 文档中使用文本注释的步骤。
步骤 1:创建 PdfWriter 对象
**PdfWriter** 类表示 PDF 的 DocWriter。此类属于 **com.itextpdf.kernel.pdf** 包。此类的构造函数接受一个字符串,表示要创建 PDF 文件的路径。
通过将字符串值(表示您需要创建 PDF 的路径)传递给其构造函数来实例化 PdfWriter 类,如下所示。
// Creating a PdfWriter String dest = "C:/itextExamples/markupAnnotation.pdf"; PdfWriter writer = new PdfWriter(dest);
当此类型的对象传递给 PdfDocument(类)时,添加到此文档的每个元素都将写入指定的文件。
步骤 2:创建 PdfDocument 对象
**PdfDocument** 类是表示 iText 中 PDF 文档的类。此类属于 **com.itextpdf.kernel.pdf** 包。要实例化此类(在写入模式下),您需要将 **PdfWriter** 类的对象传递给其构造函数。
通过将 PdfWriter 对象传递给其构造函数来实例化 PdfDocument 类,如下所示。
// Creating a PdfDocument PdfDocument pdfDoc = new PdfDocument(writer);
创建 PdfDocument 对象后,您可以使用其类提供的相应方法添加各种元素,如页面、字体、文件附件和事件处理程序。
步骤 3:创建 Document 对象
**com.itextpdf.layout** 包的 **Document** 类是创建自包含 PDF 时的根元素。此类的一个构造函数接受 **PdfDocument** 类的对象。
通过将前面步骤中创建的 **PdfDocument** 类对象传递给构造函数来实例化 **Document** 类,如下所示。
// Creating a Document Document document = new Document(pdfDoc);
步骤 4:创建 PdfAnnotation 对象
**com.itextpdf.kernel.pdf.annot** 包的 **PdfAnnotation** 类表示所有注释的超类。
在其派生类中,**PdfTextMarkupAnnotation** 类表示文本标记注释。创建此类的对象,如下所示。
// Creating a PdfTextMarkupAnnotation object Rectangle rect = new Rectangle(105, 790, 64, 10); float[] floatArray = new float[]{169, 790, 105, 790, 169, 800, 105, 800}; PdfAnnotation annotation = PdfTextMarkupAnnotation.createHighLight(rect,floatArray);
步骤 5:设置注释的颜色
使用 **PdfAnnotation** 类的 **setColor()** 方法设置注释的颜色。此方法将表示注释 **颜色** 的颜色对象作为参数传递。
// Setting color to the annotation annotation.setColor(Color.YELLOW);
步骤 6:设置注释的标题和内容
分别使用 **PdfAnnotation** 类的 **setTitle()** 和 **setContents()** 方法设置注释的标题和内容。
// Setting title to the annotation annotation.setTitle(new PdfString("Hello!")); // Setting contents to the annotation annotation.setContents(new PdfString("Hi welcome to Tutorialspoint"));
步骤 7:将注释添加到页面
使用 **PdfDocument** 类的 **addNewPage()** 方法创建一个新的 **PdfPage** 类,并使用 PdfPage 类的 **addAnnotation()** 方法添加上面创建的注释,如下所示。
// Creating a new Pdfpage PdfPage pdfPage = pdfDoc.addNewPage(); // Adding annotation to a page in a PDF pdfPage.addAnnotation(annotation);
步骤 8:关闭文档
使用 **Document** 类的 **close()** 方法关闭文档,如下所示。
// Closing the document document.close();
示例
以下 Java 程序演示了如何使用 iText 库向 PDF 文档添加文本标记注释。它创建一个名为 **markupAnnotation.pdf** 的 PDF 文档,向其中添加文本标记注释,并将其保存到 **C:/itextExamples/** 路径中。
将此代码保存在名为 **MarkupAnnotation.java** 的文件中。
import com.itextpdf.kernel.color.Color; import com.itextpdf.kernel.geom.Rectangle; import com.itextpdf.kernel.pdf.PdfDocument; import com.itextpdf.kernel.pdf.PdfPage; import com.itextpdf.kernel.pdf.PdfString; import com.itextpdf.kernel.pdf.PdfWriter; import com.itextpdf.kernel.pdf.annot.PdfAnnotation; import com.itextpdf.kernel.pdf.annot.PdfTextMarkupAnnotation; import com.itextpdf.layout.Document; public class MarkupAnnotation { public static void main(String args[]) throws Exception { // Creating a PdfDocument object String file = "C:/itextExamples/markupAnnotation.pdf"; PdfDocument pdfDoc = new PdfDocument(new PdfWriter(file)); // Creating a Document object Document doc = new Document(pdfDoc); // Creating a PdfTextMarkupAnnotation object Rectangle rect = new Rectangle(105, 790, 64, 10); float[] floatArray = new float[]{169, 790, 105, 790, 169, 800, 105, 800}; PdfAnnotation annotation = PdfTextMarkupAnnotation.createHighLight(rect,floatArray); // Setting color to the annotation annotation.setColor(Color.YELLOW); // Setting title to the annotation annotation.setTitle(new PdfString("Hello!")); // Setting contents to the annotation annotation.setContents(new PdfString("Hi welcome to Tutorialspoint")); // Creating a new Pdfpage PdfPage pdfPage = pdfDoc.addNewPage(); // Adding annotation to a page in a PDF pdfPage.addAnnotation(annotation); // Closing the document doc.close(); System.out.println("Annotation added successfully"); } }
使用以下命令从命令提示符编译并执行保存的 Java 文件:
javac MarkupAnnotation.java java MarkupAnnotation
执行后,上述程序将创建一个 PDF 文档,显示以下消息。
Annotation added successfully
如果您验证指定的路径,则可以找到如下所示的创建的 PDF 文档。
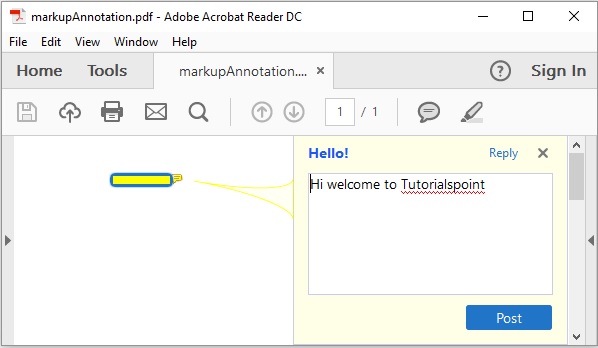