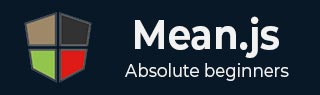
- MEAN.JS 教程
- MEAN.JS - 首页
- MEAN.JS - 概述
- MEAN.JS - 架构
- 构建 Node Web 应用
- MEAN.JS - Mean 项目设置
- 构建静态路由 Node Express
- MEAN.JS - 数据模型构建
- MEAN.JS - REST API
- 使用 Angular 的前端
- 应用中的 Angular 组件
- 使用 Angular 构建单页应用
- 构建单页应用:进阶
- MEAN.JS 有用资源
- MEAN.JS - 快速指南
- MEAN.JS - 有用资源
- MEAN.JS - 讨论
MEAN.JS - 数据模型构建
本章将演示如何在我们的 Node-express 应用中使用数据模型。
MongoDB 是一个开源的 NoSQL 数据库,它以 JSON 格式保存数据。它使用面向文档的数据模型来存储数据,而不是像关系型数据库那样使用表和行。本章将使用 Mongodb 来构建数据模型。
数据模型指定文档中存在哪些数据,以及文档中应该有哪些数据。请参考MongoDB 官方安装文档安装 MongoDB。
我们将使用上一章的代码。您可以从此链接下载源代码。下载 zip 文件;将其解压到您的系统中。打开终端并运行以下命令来安装 npm 模块依赖项。
$ cd mean-demo $ npm install
向应用程序添加 Mongoose
Mongoose 是一个数据建模库,它通过增强 MongoDB 的功能来指定数据的环境和结构。您可以通过命令行将 Mongoose 安装为 npm 模块。转到您的根文件夹并运行以下命令:
$ npm install --save mongoose
以上命令将下载新包并将其安装到node_modules文件夹中。--save标志会将此包添加到package.json文件中。
{ "name": "mean_tutorial", "version": "1.0.0", "description": "this is basic tutorial example for MEAN stack", "main": "server.js", "scripts": { "test": "test" }, "keywords": [ "MEAN", "Mongo", "Express", "Angular", "Nodejs" ], "author": "Manisha", "license": "ISC", "dependencies": { "express": "^4.17.1", "mongoose": "^5.5.13" } }
设置连接文件
为了使用数据模型,我们将使用app/models文件夹。让我们创建如下所示的students.js模型:
var mongoose = require('mongoose'); // define our students model // module.exports allows us to pass this to other files when it is called module.exports = mongoose.model('Student', { name : {type : String, default: ''} });
您可以通过创建文件并在应用程序中使用它来设置连接文件。在config/db.js中创建一个名为db.js的文件。文件内容如下:
module.exports = { url : 'mongodb://127.0.0.1:27017/test' }
这里test是数据库名称。
这里假设您已在本地安装了 MongoDB。安装完成后,启动 Mongo 并创建一个名为 test 的数据库。这个数据库将有一个名为 students 的集合。向这个集合中插入一些数据。在本例中,我们使用 `db.students.insertOne( { name: 'Manisha' , place: 'Pune', country: 'India'} );` 插入了一条记录。
将db.js文件引入应用程序,即在server.js中。文件内容如下所示:
// modules ================================================= const express = require('express'); const app = express(); var mongoose = require('mongoose'); // set our port const port = 3000; // configuration =========================================== // config files var db = require('./config/db'); console.log("connecting--",db); mongoose.connect(db.url); //Mongoose connection created // frontend routes ========================================================= app.get('/', (req, res) ⇒ res.send('Welcome to Tutorialspoint!')); //defining route app.get('/tproute', function (req, res) { res.send('This is routing for the application developed using Node and Express...'); }); // sample api route // grab the student model we just created var Student = require('./app/models/student'); app.get('/api/students', function(req, res) { // use mongoose to get all students in the database Student.find(function(err, students) { // if there is an error retrieving, send the error. // nothing after res.send(err) will execute if (err) res.send(err); res.json(students); // return all students in JSON format }); }); // startup our app at https://127.0.0.1:3000 app.listen(port, () ⇒ console.log(`Example app listening on port ${port}!`));
接下来,使用以下命令运行应用程序:
$ npm start
您将看到如下所示的确认信息:

现在,转到浏览器并输入https://127.0.0.1:3000/api/students。您将看到如下所示的页面:
