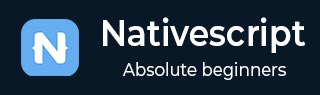
- NativeScript 教程
- NativeScript - 首页
- NativeScript - 简介
- NativeScript - 安装
- NativeScript - 架构
- NativeScript - Angular 应用
- NativeScript - 模板
- NativeScript - 小部件
- NativeScript - 布局容器
- NativeScript - 导航
- NativeScript - 事件处理
- NativeScript - 数据绑定
- NativeScript - 模块
- NativeScript - 插件
- NativeScript - 使用 JavaScript 的原生 API
- NativeScript - 在 Android 中创建应用
- NativeScript - 在 iOS 中创建应用
- NativeScript - 测试
- NativeScript 有用资源
- NativeScript - 快速指南
- NativeScript - 有用资源
- NativeScript - 讨论
NativeScript - 模块
一个 NativeScript 模块包含一组相关的功能,打包成单个库。让我们学习 NativeScript 框架提供的模块。
它包含 NativeScript 框架的核心功能。让我们在本章中了解核心模块。
应用
Application 包含移动应用程序的平台特定实现。下面定义了一个简单的核心模块:
const applicationModule = require("tns-core-modules/application");
控制台
Console 模块用于记录消息。它具有以下方法:
console.log("My FirstApp project"); console.info("Native apps!"); console.warn("Warning message!"); console.error("Exception occurred");
application-settings
application-settings 模块包含管理应用程序设置的方法。要添加此模块,我们需要添加以下代码:
const appSettings = require("tns-core-modules/application-settings");
application-setting 中的一些可用方法如下:
setBoolean(key: string, value: boolean) - 设置布尔对象
setNumber(key: string, value: number) - 设置数字对象
setString(key: string, value: string) - 设置字符串对象
getAllKeys() - 包含所有存储的键
hasKey(key: string) - 检查键是否存在
clear - 清除存储的值
remove - 基于键删除任何条目。
使用应用程序设置的一个简单示例如下:
function onNavigatingTo(args) { appSettings.setBoolean("isTurnedOff", false); appSettings.setString("name", "nativescript"); appSettings.setNumber("locationX", 54.321); const isTurnedOn = appSettings.getBoolean("isTurnedOn"); const username = appSettings.getString("username"); const locationX = appSettings.getNumber("locationX"); // Will return "not present" if there is no value for "noKey" const someKey = appSettings.getString("noKey", "not present"); } exports.onNavigatingTo = onNavigatingTo; function onClear() { // Removing a single entry via its key name appSettings.remove("isTurnedOff"); // Clearing the whole settings appSettings.clear(); }
http
此模块用于处理http请求和响应。要在您的应用程序中添加此模块,请添加以下代码:
const httpModule = require("tns-core-modules/http");
我们可以使用以下方法发送数据:
getString − 它用于发出请求并从 URL 下载数据作为字符串。其定义如下:
httpModule.getString("https://.../get").then( (r) => { viewModel.set("getStringResult", r); }, (e) => { } );
getJSON − 它用于访问 JSON 中的数据。其定义如下:
httpModule.getJSON("https://.../get").then((r) => { }, (e) => { });
getImage − 从指定的 URL 下载内容并返回 ImageSource 对象。其定义如下:
httpModule.getImage("https://.../image/jpeg").then((r) => { }, (e) => { });
getFile − 它有两个参数 URL 和文件路径。
URL − 下载数据。
文件路径 − 将 URL 数据保存到文件。其定义如下:
httpModule.getFile("https://").then((resultFile) => { }, (e) => { });
request − 它具有 options 参数。它用于请求选项并返回 HttpResponse 对象。其定义如下:
httpModule.request({ url: "https://.../get", method: "GET" }).then((response) => { }, (e) => { });
Image-source
image-source 模块用于保存图像。我们可以使用以下语句添加此模块:
const imageSourceModule = require("tns-core-modules/image-source");
如果要从资源加载图像,请使用以下代码:
const imgFromResources = imageSourceModule.fromResource("icon");
要从本地文件添加图像,请使用以下命令:
const folder = fileSystemModule.knownFolders.currentApp(); const path = fileSystemModule.path.join(folder.path, "images/sample.png"); const imageFromLocalFile = imageSourceModule.fromFile(path);
要将图像保存到文件路径,请使用以下命令:
const img = imageSourceModule.fromFile(imagePath); const folderDest = fileSystemModule.knownFolders.documents(); const pathDest = fileSystemModule.path.join(folderDest.path, "sample.png"); const saved = img.saveToFile(pathDest, "png"); if (saved) { console.log(" sample image saved successfully!"); }
计时器
此模块用于在特定时间间隔执行代码。要添加它,我们需要使用require:
const timerModule = require("tns-core-modules/timer");
它基于两种方法:
setTimeout − 用于延迟执行。它以毫秒表示。
setInterval − 用于以特定间隔重复应用。
跟踪
此模块对于调试很有用。它提供日志信息。此模块可以表示为:
const traceModule = require("tns-core-modules/trace");
如果要在您的应用程序中启用它,请使用以下命令:
traceModule.enable();
ui/image-cache
image-cache 模块用于处理图像下载请求和缓存下载的图像。此模块可以表示如下:
const Cache = require("tns-core-modules/ui/image-cache").Cache;
连接性
此模块用于接收已连接网络的连接信息。它可以表示为:
const connectivityModule = require("tns-core-modules/connectivity");
功能模块
功能模块包括许多特定于系统/平台的模块。一些重要的模块如下:
platform − 用于显示有关您的设备的信息。它可以定义为如下所示:
const platformModule = require("tns-core-modules/platform");
fps-meter − 用于捕获每秒帧数。它可以定义为如下所示:
const fpsMeter = require("tns-core-modules/fps-meter");
file-system − 用于处理您的设备文件系统。其定义如下:
const fileSystemModule = require("tns-core-modules/file-system");
ui/gestures − 用于处理 UI 手势。
UI 模块
UI 模块包含 UI 组件及其相关功能。一些重要的 UI 模块如下:
框架
页面
颜色
text/formatted-string
xml
样式
动画