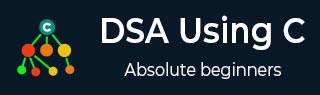
- 使用C语言实现数据结构教程
- 使用C语言实现数据结构 - 首页
- 使用C语言实现数据结构 - 概述
- 使用C语言实现数据结构 - 环境配置
- 使用C语言实现数据结构 - 算法
- 使用C语言实现数据结构 - 概念
- 使用C语言实现数据结构 - 数组
- 使用C语言实现数据结构 - 链表
- 使用C语言实现数据结构 - 双向链表
- 使用C语言实现数据结构 - 循环链表
- 使用C语言实现数据结构 - 栈
- 使用C语言实现数据结构 - 表达式解析
- 使用C语言实现数据结构 - 队列
- 使用C语言实现数据结构 - 优先队列
- 使用C语言实现数据结构 - 树
- 使用C语言实现数据结构 - 哈希表
- 使用C语言实现数据结构 - 堆
- 使用C语言实现数据结构 - 图
- 使用C语言实现数据结构 - 搜索技术
- 使用C语言实现数据结构 - 排序技术
- 使用C语言实现数据结构 - 递归
- 使用C语言实现数据结构 - 有用资源
- 使用C语言实现数据结构 - 快速指南
- 使用C语言实现数据结构 - 有用资源
- 使用C语言实现数据结构 - 讨论
使用C语言实现数据结构 - 栈
概述
栈是一种数据结构,它只允许在一端进行数据操作。它只允许访问最后插入的数据。栈也被称为后进先出 (LIFO) 数据结构,并且入栈和出栈操作以这样一种方式相关联:只有最后入栈(添加到栈中)的项才能出栈(从栈中移除)。
栈的表示
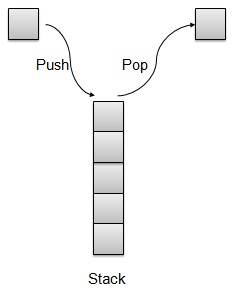
在本文中,我们将使用数组来实现栈。
基本操作
以下是栈的两个主要操作。
入栈 (Push) − 将元素压入栈顶。
出栈 (Pop) − 将元素弹出栈顶。
栈还支持一些其他操作,如下所示。
取顶 (Peek) − 获取栈顶元素。
是否已满 (isFull) − 检查栈是否已满。
是否为空 (isEmpty) − 检查栈是否为空。
入栈操作
每当一个元素被压入栈时,栈会将该元素存储在存储区的顶部,并增加顶部索引以供后续使用。如果存储区已满,通常会显示错误消息。
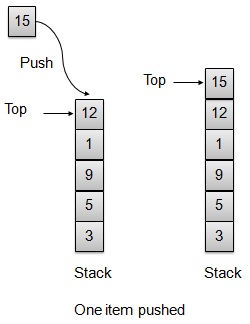
// Operation : Push // push item on the top of the stack void push(int data) { if(!isFull()){ // increment top by 1 and insert data intArray[++top] = data; } else { printf("Cannot add data. Stack is full.\n"); } }
出栈操作
每当需要从栈中弹出元素时,栈会从存储区的顶部检索该元素,并减少顶部索引以供后续使用。
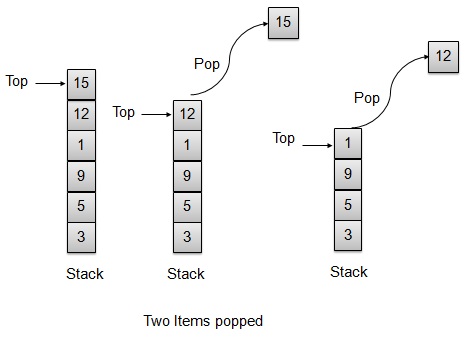
// Operation : Pop // pop item from the top of the stack int pop() { //retrieve data and decrement the top by 1 return intArray[top--]; }
示例
StackDemo.c
#include <stdio.h> #include <string.h> #include <stdlib.h> #include <stdbool.h> // size of the stack int size = 8; // stack storage int intArray[8]; // top of the stack int top = -1; // Operation : Pop // pop item from the top of the stack int pop() { //retrieve data and decrement the top by 1 return intArray[top--]; } // Operation : Peek // view the data at top of the stack int peek() { //retrieve data from the top return intArray[top]; } //Operation : isFull //return true if stack is full bool isFull(){ return (top == size-1); } // Operation : isEmpty // return true if stack is empty bool isEmpty(){ return (top == -1); } // Operation : Push // push item on the top of the stack void push(int data) { if(!isFull()){ // increment top by 1 and insert data intArray[++top] = data; } else { printf("Cannot add data. Stack is full.\n"); } } main() { // push items on to the stack push(3); push(5); push(9); push(1); push(12); push(15); printf("Element at top of the stack: %d\n" ,peek()); printf("Elements: \n"); // print stack data while(!isEmpty()){ int data = pop(); printf("%d\n",data); } printf("Stack full: %s\n" , isFull()?"true":"false"); printf("Stack empty: %s\n" , isEmpty()?"true":"false"); }
输出
如果我们编译并运行上述程序,它将产生以下输出:
Element at top of the stack: 15 Elements: 15 12 1 9 5 3 Stack full: false Stack empty: true
广告