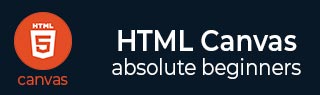
- HTML Canvas 教程
- HTML Canvas - 首页
- HTML Canvas - 简介
- 环境设置
- HTML Canvas - 第一个应用程序
- HTML Canvas - 绘制 2D 形状
- HTML Canvas - 路径元素
- 使用路径元素绘制 2D 形状
- HTML Canvas - 颜色
- HTML Canvas - 添加样式
- HTML Canvas - 添加文本
- HTML Canvas - 添加图像
- HTML Canvas - Canvas 时钟
- HTML Canvas - 变换
- 合成和剪辑
- HTML Canvas - 基本动画
- 高级动画
- HTML Canvas API 函数
- HTML Canvas - 元素
- HTML Canvas - 矩形
- HTML Canvas - 线
- HTML Canvas - 路径
- HTML Canvas - 文本
- HTML Canvas - 颜色和样式
- HTML Canvas - 图像
- HTML Canvas - 阴影和变换
- HTML Canvas 有用资源
- HTML Canvas - 快速指南
- HTML Canvas - 有用资源
- HTML Canvas - 讨论
HTML Canvas - 过滤器属性
HTML Canvas 的filter 属性用于应用颜色和对比度效果(例如灰度、棕褐色等)到使用上下文对象绘制到画布元素上的图像。
它的工作原理与 CSS filter 属性完全相同,并采用相同的输入值。它将提供的过滤器与确切的值应用于 Canvas 元素内的图像。
可能的输入值
filter属性接受“none”值,该值不返回任何内容。参数接受的值如下所示。
序号 | 值和描述 |
---|---|
1 | url()
当需要 CSS URL 来访问过滤器数据并将其应用于CanvasRenderingContext2D对象时,将给出此值。 |
2 | blur()
当此值作为对象过滤器给出时,对象会变得模糊。输入值为整数,当给出零时,对象保持不变。 |
3 | brightness()
此内置方法用于使对象更亮或更暗。如果给定的值小于 100%,则对象变暗,如果值大于 100%,则对象变亮。 |
4 | contrast()
filter属性的此值用于调整绘图的对比度。它以百分比给出,0% 会在画布上生成一个完全黑色的对象。 |
5 | drop-shadow()
它将阴影应用于对象。它需要四个值来执行此操作,即阴影与对象的距离、半径和阴影的颜色。 |
6 | grayscale()
filter属性的此值将对象转换为灰度。它接受百分比值作为输入。 |
7 | hue-rotate()
此值将色调旋转应用于绘图。它以度数给出,0deg 不会改变绘图。 |
8 | invert()
此方法反转画布中的对象,并采用百分比值来执行操作。 |
9 | opacity()
要使绘图透明,会调用此过滤器属性的方法。它也采用百分比值作为输入。 |
10 | saturation()
要使绘图饱和,我们将此过滤器属性应用于CanvasRenderingContext2D对象,方法是提供百分比值。 |
11 | sepia()
当通过传递表示过滤器需求的百分比值调用时,这会向绘图添加棕褐色过滤器。 |
12 | none
不应用任何过滤器,绘图保持不变。 |
示例
以下程序将“brightness”值应用于创建的上下文对象的 HTML Canvas filter 属性。
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="500" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'brightness(150%)' context.fillStyle = 'cyan'; context.fill(); context.fillRect(50, 20, 150, 100); context.filter = 'brightness(50%)' context.fillStyle = 'cyan'; context.fill(); context.fillRect(250, 20, 150, 100); } </script> </body> </html>
输出
上述代码在网页上返回的输出为:
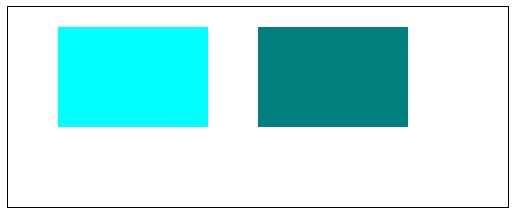
示例
以下程序将模糊过滤器属性应用于创建的上下文对象。
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="400" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'blur(3px)'; var image = new Image(); image.onload = function() { context.drawImage(image, 50, 50); }; image.src = 'https://tutorialspoint.com/html5/images/logo.png'; } </script> </body> </html>
输出
上述代码在网页上返回的输出为:
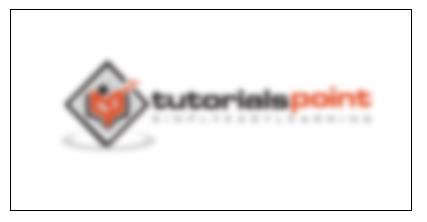
示例
以下程序将对比度过滤器属性应用于创建的上下文对象。
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="200" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'contrast(30%)'; context.beginPath(); context.arc(100, 100, 50, 1 * Math.PI, 5 * Math.PI); context.fill(); context.closePath(); } </script> </body> </html>
输出
上述代码在网页上返回的输出为:
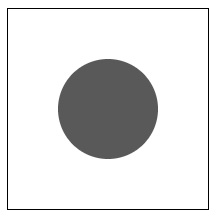
示例
以下程序将阴影过滤器属性应用于创建的上下文对象。
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="400" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'drop-shadow(20px 10px grey)'; var image = new Image(); image.onload = function() { context.drawImage(image, 50, 50); }; image.src = 'https://tutorialspoint.com/html5/images/logo.png'; } </script> </body> </html>
输出
上述代码在网页上返回的输出为:

示例
以下程序将灰度过滤器属性应用于创建的上下文对象。
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="400" height="200" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'grayscale(125%)'; var image = new Image(); image.onload = function() { context.drawImage(image, 50, 50); }; image.src = 'https://tutorialspoint.com/html5/images/logo.png'; } </script> </body> </html>
输出
上述代码在网页上返回的输出为:

示例
以下程序将色调旋转过滤器属性应用于创建的上下文对象。
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="625" height="425" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'hue-rotate(45deg)'; var image = new Image(); image.onload = function() { context.drawImage(image, 10, 10); }; image.src = '../../images/image3.png'; } </script> </body> </html>
输出
此程序中使用的图像为:
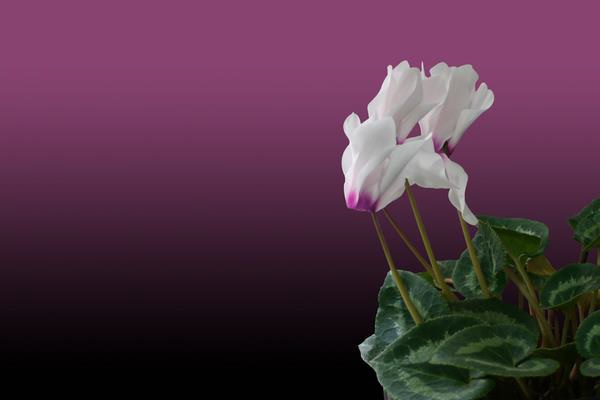
上述代码在网页上返回的输出为:
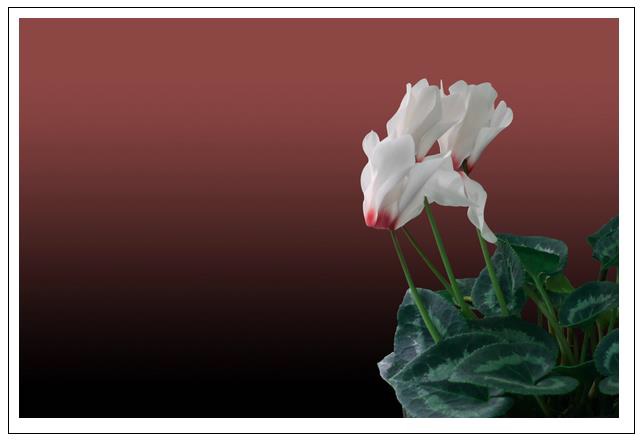
示例
以下程序将反转过滤器属性应用于创建的上下文对象。
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="625" height="425" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'invert(100%)'; var image = new Image(); image.onload = function() { context.drawImage(image, 10, 10); }; image.src = '../../images/image7.png'; } </script> </body> </html>
输出
此程序中使用的图像为:
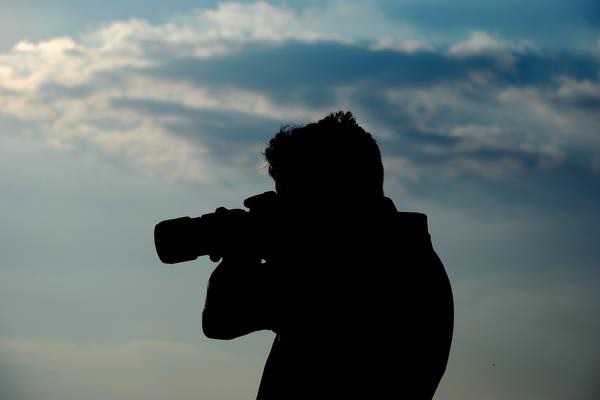
上述代码在网页上返回的输出为:
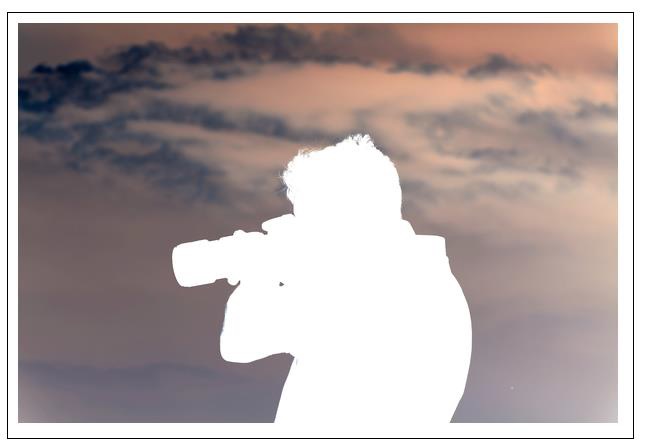
示例
以下程序将不透明度过滤器属性应用于创建的上下文对象。
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload = "Context();"> <canvas id="canvas" width="625" height="425" style="border: 1px solid black;"></canvas> <script> function Context(){ var canvas=document.getElementById('canvas'); var context=canvas.getContext('2d'); context.filter = 'opacity(75%)'; var image = new Image(); image.onload = function() { context.drawImage(image, 10, 10); }; image.src = '../../images/image33.png'; } </script> </body> </html>
输出
此程序中使用的图像为:
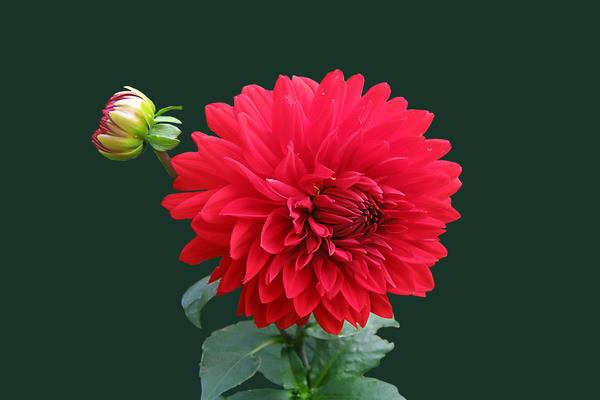
上述代码在网页上返回的输出为:
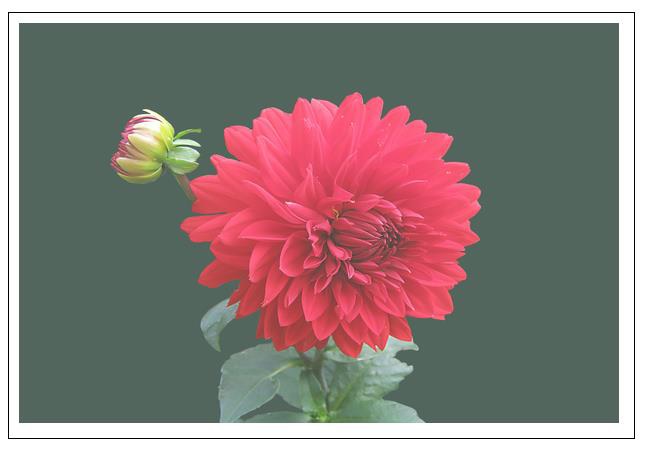
示例
以下程序将饱和度过滤器属性应用于创建的上下文对象。
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload = "Context();"> <canvas id="canvas" width="625" height="425" style="border: 1px solid black;"></canvas> <script> function Context(){ var canvas=document.getElementById('canvas'); var context=canvas.getContext('2d'); context.filter = 'saturate(250%)'; var image = new Image(); image.onload = function() { context.drawImage(image, 10, 10); }; image.src = '../../images/image66.png'; } </script> </body> </html>
输出
此程序中使用的图像为
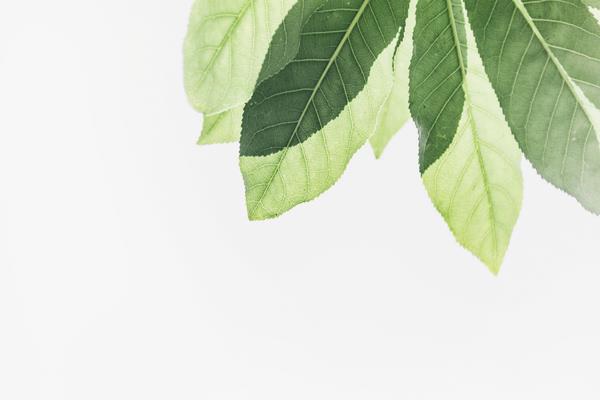
上述代码在网页上返回的输出为:
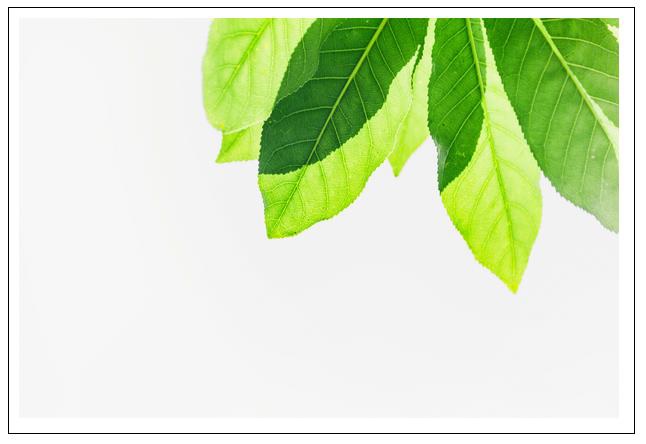
示例
以下程序将棕褐色过滤器属性应用于创建的上下文对象。
<!DOCTYPE html> <html lang="en"> <head> <title>Reference API</title> <style> body { margin: 10px; padding: 10px; } </style> </head> <body onload="Context();"> <canvas id="canvas" width="625" height="425" style="border: 1px solid black;"></canvas> <script> function Context() { var canvas = document.getElementById('canvas'); var context = canvas.getContext('2d'); context.filter = 'sepia(95%)'; var image = new Image(); image.onload = function() { context.drawImage(image, 10, 10); }; image.src = '../../images/image77.png'; } </script> </body> </html>
输出
此程序中使用的图像为:
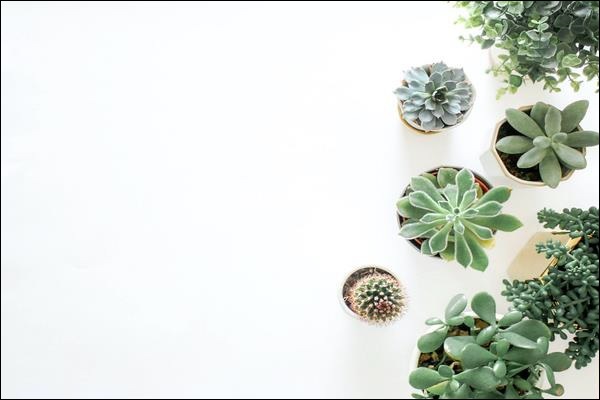
上述代码在网页上返回的输出为:
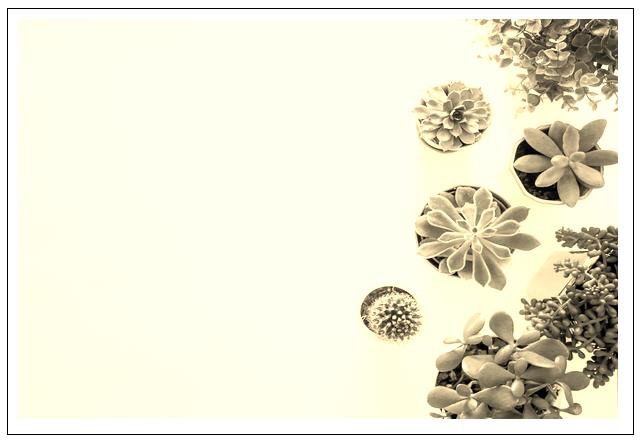