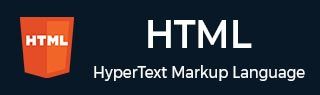
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - Iframes
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用的标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 属性指定的属性
HTML DOM 属性的 **specified** 属性用于检查是否指定了提到的属性。如果指定了提到的属性,则返回 true,否则会抛出错误。
语法
attribute.specified
返回值
如果在该标签中提到了该属性,则 `specified` 属性返回 true,否则程序停止运行。
HTML DOM 属性“specified”属性的示例
以下示例说明如何在 JavaScript 和 HTML 中使用 `specified` 属性。
检查是否指定了 onclick 属性
以下代码演示了如何使用 `specified` 属性来检查 HTML 元素的属性是否被显式指定。
<!DOCTYPE html> <html> <head> <title>HTML DOM Attribute specified Property</title> </head> <body> <h3>HTML DOM Attribute specified Property</h3> <div id="Div" style="background-color:blue;height:50px" onclick=""> </div> <p>Is the onclick attribute specified?</p> <p id="par"></p> <script> document.getElementById("par").innerHTML = document.getElementById("Div").getAttributeNode("onclick").specified; </script> </body> </html>
未提及属性时使程序崩溃
以下代码显示了当我们尝试检查不存在的属性的存在时会发生什么。它在显示两个结果后崩溃。
<!DOCTYPE html> <html> <head> <title>HTML DOM Attribute specified Property</title> </head> <body> <h3>HTML DOM Attribute specified Property</h3> <div id="TestDiv" style="background-color:red;height:50px" onclick=""> </div> <p> Are the following attributes specified for the element? </p> <p id="onclickResult"></p> <p id="idResult"></p> <p id="classResult"></p> <p id="styleResult"></p> <script> var element = document.getElementById("TestDiv"); // Check if the 'onclick' attribute is specified document.getElementById("onclickResult").innerHTML = "onclick: " + element.getAttributeNode("onclick").specified; // Check if the 'id' attribute is specified document.getElementById("idResult").innerHTML = "id: " + element.getAttributeNode("id").specified; // Check if the 'class' attribute is specified // We didnt mentioned class attribute for div // tag, hence program crashes here, you can't. // see result of class and style attributes. document.getElementById("classResult").innerHTML = "class: " + element.getAttributeNode("class").specified; // Check if the 'style' attribute is specified document.getElementById("styleResult").innerHTML = "style: " + element.getAttributeNode("style").specified; </script> </body> </html>
处理程序崩溃
在这里,我们将了解如何处理程序崩溃。在尝试使用 三元运算符 访问 specified 属性之前,我们将检查属性是否存在。
<!DOCTYPE html> <html> <head> <title>HTML DOM Attribute specified Property</title> </head> <body> <h3>HTML DOM Attribute specified Property</h3> <div id="TestDiv" style="background-color:red;height:50px" onclick=""> </div> <p> Are the following attributes specified for the element? </p> <p id="onclickResult"></p> <p id="idResult"></p> <p id="classResult"></p> <p id="styleResult"></p> <script> var element = document.getElementById("TestDiv"); // Check if the 'onclick' attribute is specified var onclickAttr = element.getAttributeNode("onclick"); document.getElementById("onclickResult").innerHTML = "onclick: " + (onclickAttr ? onclickAttr.specified : false); // Check if the 'id' attribute is specified var idAttr = element.getAttributeNode("id"); document.getElementById("idResult").innerHTML = "id: " + (idAttr ? idAttr.specified : false); // Check if the 'class' attribute is specified var classAttr = element.getAttributeNode("class"); document.getElementById("classResult").innerHTML = "class: " + (classAttr ? classAttr.specified : false); // Check if the 'style' attribute is specified var styleAttr = element.getAttributeNode("style"); document.getElementById("styleResult").innerHTML = "style: " + (styleAttr ? styleAttr.specified : false); </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
specified | 是 | 是 | 是 | 是 | 是 |
广告