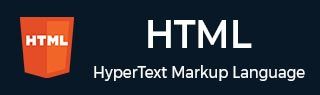
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - 内嵌框架
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - 地理位置 API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 表格查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - 地理位置 API
HTML 地理位置 API 用于 Web 应用程序访问用户的地理位置。大多数现代浏览器和移动设备都支持 地理位置 API。
JavaScript 可以捕获您的纬度和经度,并可以发送到后端 Web 服务器,并执行诸如查找本地企业或在地图上显示您的位置等复杂的基于位置的功能。
语法
var geolocation = navigator.geolocation;
地理位置对象是一个服务对象,允许小部件检索有关设备地理位置的信息。
地理位置 API 方法
地理位置 API 提供以下方法
方法 | 描述 |
---|---|
getCurrentPosition() |
此方法检索用户的当前地理位置。 |
watchPosition() |
此方法检索有关设备当前地理位置的定期更新。 |
clearWatch() |
此方法取消正在进行的 watchPosition 调用。 |
示例
以下是使用上述任何方法的示例代码
function getLocation() { var geolocation = navigator.geolocation; geolocation.getCurrentPosition(showLocation, errorHandler); watchId = geolocation.watchPosition(showLocation, errorHandler, { enableHighAccuracy: true, timeout: 5000, maximumAge: 0 }); navigator.geolocation.clearWatch(watchId); }
这里,showLocation
和 errorHandler
是回调方法,将用于获取实际位置(如下一节所述)以及处理可能出现的错误。
位置属性
地理位置方法 getCurrentPosition() 和 getPositionUsingMethodName() 指定检索位置信息的回调方法。这些方法与存储完整位置信息的 Position
对象异步调用。
Position 对象指定设备的当前地理位置。位置表示为一组地理坐标以及有关航向和速度的信息。
下表描述了 Position 对象的属性。对于可选属性,如果系统无法提供值,则该属性的值设置为 null。
属性 | 类型 | 描述 |
---|---|---|
coords | 对象 | 指定设备的地理位置。位置表示为一组地理坐标以及有关航向和速度的信息。 |
coords.latitude | 数字 | 指定以十进制度表示的纬度估计值。值范围为 [-90.00,+90.00]。 |
coords.longitude | 数字 | 指定以十进制度表示的经度估计值。值范围为 [-180.00,+180.00]。 |
coords.altitude | 数字 | [可选] 指定相对于 WGS 84 参考椭球面的海拔高度估计值(以米为单位)。 |
coords.accuracy | 数字 | [可选] 指定纬度和经度估计值的精度(以米为单位)。 |
coords.altitudeAccuracy | 数字 | [可选] 指定海拔高度估计值的精度(以米为单位)。 |
coords.heading | 数字 | [可选] 指定设备当前运动方向(以度为单位),相对于正北方向顺时针方向计算。 |
coords.speed | 数字 | [可选] 指定设备当前地面速度(以米/秒为单位)。 |
timestamp | 日期 | 指定检索位置信息和创建 Position 对象的时间。 |
示例
以下是使用“position
”对象的示例代码。这里,showLocation()
方法是一个回调方法
function showLocation( position ) { var latitude = position.coords.latitude; var longitude = position.coords.longitude; ... }
处理错误
地理位置很复杂,非常需要捕获任何错误并优雅地处理它。
地理位置方法 getCurrentPosition()
和 watchPosition()
使用错误处理回调方法,该方法提供 PositionError 对象。此对象具有以下两个属性
属性 | 类型 | 描述 |
---|---|---|
code | 数字 | 包含错误的数字代码。 |
message | 字符串 | 包含错误的人类可读描述。 |
下表描述了 PositionError 对象中返回的可能错误代码。
代码 | 常量 | 描述 |
---|---|---|
0 | UNKNOWN_ERROR | 由于未知错误,该方法无法检索设备的位置。 |
1 | PERMISSION_DENIED | 该方法无法检索设备的位置,因为应用程序没有权限使用位置服务。 |
2 | POSITION_UNAVAILABLE | 无法确定设备的位置。 |
3 | TIMEOUT | 该方法无法在指定的最大超时间隔内检索位置信息。 |
示例
以下是使用 PositionError
对象的示例代码。这里 errorHandler
方法是一个回调方法
function errorHandler( err ) { if (err.code == 1) { // access is denied } ... }
位置选项
以下是 getCurrentPosition()
方法的实际语法
getCurrentPosition(callback, ErrorCallback, options)
这里,第三个参数是 PositionOptions
对象,它指定了一组用于检索设备地理位置的选项。
以下是可以作为第三个参数指定的选项
属性 | 类型 | 描述 |
---|---|---|
enableHighAccuracy | 布尔值 | 指定小部件是否希望接收尽可能准确的位置估计。默认情况下,此值为 false。 |
timeout | 数字 | timeout 属性是 Web 应用程序愿意等待位置的毫秒数。 |
maximumAge | 数字 | 指定缓存位置信息的过期时间(以毫秒为单位)。 |
示例
以下是显示如何使用上述方法的示例代码
function getLocation() { var geolocation = navigator.geolocation; geolocation.getCurrentPosition(showLocation, errorHandler, {maximumAge: 75000}); }
HTML 地理位置 API 示例
以下是一些示例,展示了如何在 HTML 中访问地理位置
获取当前位置
以下代码显示了如何使用 JavaScript 和 HTML 访问设备的当前位置。
<!DOCTYPE html> <html> <head> <title> Geolocation API Example </title> </head> <body> <h2>Geolocation API Example</h2> <p id="demo"> Click the button to get your coordinates: </p> <button onclick="getLocation()"> Show Location </button> <script> var x = document.getElementById("demo"); function getLocation() { if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(showPosition); } else { x.innerHTML = "Geolocation is not supported by this browser."; } } function showPosition(position) { x.innerHTML = "Latitude: " + position.coords.latitude + "<br>Longitude: " + position.coords.longitude; } </script> </body> </html>
地理位置中的错误处理
以下是显示如何使用上述方法的示例代码
<!DOCTYPE html> <html> <head> <title>Geolocation API Example</title> </head> <body> <h2>Geolocation API Example</h2> <p id="demo"> Turn off location service of your device, See how the error is handled. </p> <button onclick="getLocation()"> Show Location </button> <script> var x = document.getElementById("demo"); function getLocation() { if (navigator.geolocation) { navigator.geolocation.getCurrentPosition(showPosition, showError); } else { x.innerHTML = "Geolocation is not supported by this browser."; } } function showPosition(position) { x.innerHTML = "Latitude: " + position.coords.latitude + "<br>Longitude: " + position.coords.longitude; } function showError(error) { switch(error.code) { case error.PERMISSION_DENIED: x.innerHTML = "User denied the request for Geolocation."; break; case error.POSITION_UNAVAILABLE: x.innerHTML = "Location information is unavailable."; break; case error.TIMEOUT: x.innerHTML = "The request to get user location timed out."; break; case error.UNKNOWN_ERROR: x.innerHTML = "An unknown error occurred."; break; } } </script> </body> </html>
支持的浏览器
API | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
地理位置 | 是 | 9.0 | 3.5 | 5.0 | 16.0 |