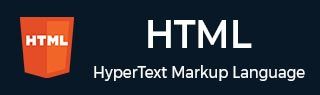
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - Iframes
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - 地理位置 API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 表格查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM firstChild 属性
HTML DOM 的firstChild 属性用于访问和检索指定父元素的第一个子节点。在 HTML DOM 中,除非与其他节点(元素)进行比较,否则元素不能被视为父节点或子节点。
例如,单个<ul> 元素本身并不是父元素或子元素。但是,当与<li> 元素进行比较时,“ul”被视为父元素,而“li”元素被视为其子元素。
语法
以下是 HTML DOM 的firstChild 属性的语法:
element.firstChild;
参数
由于它是一个属性,因此不接受任何参数。
返回值
此属性返回给定父元素的第一个子元素。如果不存在第一个子元素,则返回“null”。
示例 1:访问 Div 元素的第一个子节点
以下程序演示了如何使用 HTML DOM 的firstChild 属性来访问<div> 元素的第一个子节点:
<!DOCTYPE html> <html> <head> <title>HTML DOM firstChild</title> </head> <body> <h4>Displays the first child Node...</h4> <div id="parent"> <p>This is the first child node.</p> <p>This is the second child node.</p> </div> <div id="otput"></div> <script> var parent = document.getElementById("parent"); var firstChild = parent.firstElementChild; function displayResult() { var outputDiv=document.getElementById("otput"); outputDiv.textContent = "First Child Node: " + firstChild.textContent.trim(); } // Call the function to display the result displayResult(); </script> </body> </html>
上述程序访问并返回“div”(即父节点)元素的第一个子节点。
示例 2:处理没有子节点的情况
以下是 HTML DOM 的firstChild 属性的另一个示例。我们使用此属性来检查元素是否有任何子节点:
<!DOCTYPE html> <html> <head> <title>HTML DOM firstChild</title> </head> <body> <h4>This example does not have any child nodes...</h4> <div id="pt"></div> <div id="output"></div> <script> var parent = document.getElementById("pt"); var firstChild = parent.firstChild; var resultMessage; if (firstChild === null) { resultMessage="The element has no child nodes."; } else { resultMessage = firstChild.textContent.trim(); } // Function to display result in output div function displayResult() { var odiv = document.getElementById("output"); odiv.textContent = resultMessage; } displayResult(); </script> </body> </html>
示例 3:显示 Body 元素的第一个子节点
此示例显示了firstChild 属性的使用。它检索 body 元素的第一个子节点,这是一个 <p> 元素:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM firstChild</title> </head> <body> <p>Displays first child node of body</p> <h4>Welcome to Tutorialspoint</h4> <div id="ot"></div> <script> // Selecting the body element var bodyElement = document.body; // Retrieving and displays the first child node var firstChild = bodyElement.firstChild; function displayResult() { var otdiv = document.getElementById("ot"); otdiv.innerHTML = "First Child Node of Body:" + firstChild.nodeName; } displayResult(); </script> </body> </html>
示例 4:获取 ul 元素的第一个子元素
此示例演示了如何使用firstChild 属性访问 ID 为“myList”的 <ul> 元素的第一个子节点:
<!DOCTYPE html> <html lang="en"> <head> <title>Retrieving First Child Node HTML</title> </head> <body> <p>Displays HTML content of first child node of "ul" element.</p> <ul id="myList"> <li>Item1</li> <li>Item2</li> <li>Item3</li> </ul> <button onclick="getFirstChild()">Get First Child Node</button> <div id="ot"></div> <script> function getFirstChild() { // Accessing the ul element const ul = document.getElementById('myList'); const firstChildNode = ul.firstChild; if(firstChildNode.nodeType === Node.TEXT_NODE){ const firstChildElement = firstChildNode.nextSibling; const otDiv = document.getElementById('ot'); otDiv.innerHTML = `<p>HTML content of first child node: </p>${firstChildElement.outerHTML}`; } else{ const otDiv = document.getElementById('ot'); otDiv.innerHTML = 'No child node found..!'; } } </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
firstChild | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告