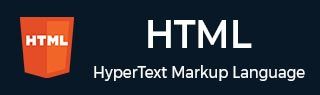
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - 内联框架 (iframe)
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表格标题与字幕
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称与值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - WebRTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity 绘图
- HTML - 二维码
- HTML - Modernizr
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查询
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用的标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 children 属性
元素的 **children** 属性返回其子元素的集合,表示为一个 HTMLCollection 对象,不包括文本节点和其他非元素节点。
语法
element.children;
返回值
children 属性返回一个 HTMLCollection 对象,其中包含父元素的子元素集合。
HTML DOM 元素 'children' 属性示例
以下是一些示例,展示了 'children' 属性的使用,以便更好地理解。
检索 Div 元素的子元素
此示例演示如何使用 children 属性检索 ID 为 "pE" 的 **<div>** 元素的子元素。
<!DOCTYPE html> <html lang="en"> <head> <title>Children Example</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>children Property</h2> <p>It retrieves child elements of the parent ...</p> <div id="parent"> <p>Child 1</p> <p>Child 2</p> <p>Child 3</p> </div> <div id="output"></div> <script> const pE=document.getElementById('parent'); const children = pE.children; // Displaying child elements in the output div let res="<p><strong>Child Elements:</strong></p>"; for (let i = 0; i < children.length; i++) { res += `<p>${children[i].textContent}</p>`; } document.getElementById('output').innerHTML=res; </script> </body> </html>
计算 Div 元素的子元素数量
此示例演示了 children 属性的基本用法,用于计算并显示 **<div>** 元素中子元素的数量。
<!DOCTYPE html> <html> <head> <title>Count Children Example</title> </head> <body> <h2>HTML - DOM Element</h2> <h1>children Property</h1> <p>Displays the number of childrens...</p> <div id="myDIV"> <p>Paragraph 1</p> <p>Paragraph 2</p> <p>Paragraph 3</p> </div> <button onclick="countChildren()"> Count Children </button> <div class="output"> <p>Number of children:<span id="Cc">-</span></p> </div> <script> function countChildren() { let myDiv = document.getElementById("myDIV"); let childC = myDiv.children.length; document.getElementById("Cc").textContent = childC; } </script> </body> </html>
更改子元素的背景颜色
此示例演示如何使用 parent.children 属性动态更改 <div> 内所有子元素的背景颜色。
<!DOCTYPE html> <html lang="en"> <head> <title>Change Children Background Example</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>children Property</h2> <p>It Changes background colour for the Children</p> <div id="pt"> <div>Child 1</div> <div>Child 2</div> <div>Child 3</div> </div> <button onclick="changeChildrenBackground()"> Change Children Background </button> <script> function changeChildrenBackground() { const parentDiv=document.getElementById('pt'); const children = parentDiv.children; for (let i = 0; i < children.length; i++) { const child = children[i]; child.style.backgroundColor = '#ff80f0'; } } </script> </body> </html>
检索选择框中第三个子元素的文本
此示例有效地展示了如何使用 children 属性与下拉菜单交互并根据其索引检索特定元素的内容。它显示第三个子元素的文本。
<!DOCTYPE html> <html lang="en"> <head> <title>Get Text of Third Child Element in Select</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>children Property</h2> <p>Get Text of Third Child Element in Select</p> <select id="mySelect"> <option value="1">Option 1</option> <option value="2">Option 2</option> <option value="3">Option 3</option> </select> <button onclick="getThirdChild()"> Get Text of Third Child </button> <p id="result"></p> <script> function getThirdChild() { const s=document.getElementById('mySelect'); const thirdText=s.options[2].textContent; document.getElementById('result').textContent= `Text of third child element: ${thirdText}`; } </script> </body> </html>
更改第二个子元素的背景
此示例演示了如何使用 children 属性动态更改 <div> 元素中第二个子元素的背景颜色。
<!DOCTYPE html> <html lang="en"> <head> <title>Changes Background for Second Child</title> <style> .child { padding: 10px; margin: 5px; border: 1px solid #ccc; } </style> </head> <body> <h2>Change Background of Second Child Element</h2> <div id="myDIV"> <div class="child">Child 1</div> <div class="child">Child 2</div> <div class="child">Child 3</div> </div> <button onclick="changeBackground()"> Change Background of Second Child </button> <script> function changeBackground() { const pdiv = document.getElementById('myDIV'); // Index 1 for the second child element const secondChild = pdiv.children[1]; pdiv.children[1].style.backgroundColor='violet'; } </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
children | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告