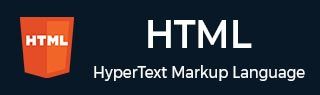
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引号
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - 内联框架
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表格头部和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 表格查询
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM className 属性
HTML DOM 的className 属性用于访问或修改(更改)元素的 class 属性的值,表示为以空格分隔的字符串。
语法
以下是 HTML DOM 的className(设置类名)属性的语法:
element.className = class_name;
要检索类名属性,请使用以下语法:
element.className;
其中,class_name 是您要应用于元素的类名。对于多个类,请在字符串中用空格分隔它们。
参数
由于它是一个属性,因此它不接受任何参数。
返回值
此属性返回一个字符串,其中包含分配给元素的所有类,每个类之间用空格分隔。
示例 1:使用 className 应用样式
以下示例演示了 HTML DOM 的className 属性的用法。它将多个 CSS 类应用于 HTML 元素:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM className</title> <style> .highlight { color: green; font-weight: bold; font-style: italic; } </style> </head> <body> <p>Click the below button to style with className...</p> <p id="ex">A paragraph with default styling.</p> <button onclick="applyStyles()">Apply Styles</button> <script> var paraElement = document.getElementById("ex"); function applyStyles() { paraElement.className = "highlight"; } </script> </body> </html>
示例 2:切换 Div 元素的样式
这是另一个使用 HTML DOM 的className 属性的示例。我们使用此属性向<div> 元素添加一个类名,并在 CSS 中使用该类名对其进行样式设置:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM className</title> <style> .highlight { background-color: pink; color: blue; padding: 10px; border: 1px solid black; } .italic { font-style: italic; } </style> </head> <body> <p>Click the below button to style with className...</p> <button onclick="toggleStyles()">Toggle Styles</button> <br><br> <div id="ex" class="highlight">This is a div with default styling.</div> <script> function toggleStyles() { var divElement = document.getElementById("ex"); divElement.classList.toggle("italic"); } </script> </body> </html>
示例 3:检索元素的 Class 属性
下面的示例显示了如何使用className 属性访问和显示 HTML 元素的 class 属性:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM className</title> <style> .highlight { background-color: AquaMarine; color: blue; padding: 10px; font-style: italic; } </style> </head> <body> <div id="myDIV" class="highlight italic">This is a div with classes "highlight" and "italic".</div> <br> <button onclick="getClassAttribute()">Get Class Attribute</button> <p id="cl"></p> <script> function getClassAttribute() { var divEle = document.getElementById("myDIV"); var clat = divEle.getAttribute("class"); document.getElementById("cl").textContent = "Class attribute value: " + clat; } </script> </body> </html>
示例 4:检索具有多个类的 Class 属性
此示例显示了如何使用className 属性获取具有多个类的元素的 class 属性:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM className</title> <style> .highlight { background-color: yellow; } .italic { font-style: italic; } .bold { font-weight: bold; } </style> </head> <body> <p>Retrieves the class attribute of an element with multiple classes.</p> <div id="myDiv" class="highlight italic bold">Sample Div Element</div> <br> <button onclick="getClassAttribute()">Get Class Attribute</button> <p id="Res"></p> <script> function getClassAttribute() { const ele = document.getElementById('myDiv'); const classAttr = ele.className; document.getElementById('Res').textContent = `Class Attribute: ${classAttr}`; } </script> </body> </html>
示例 5:在多个类之间切换
此示例向我们展示了如何在不同方式之间切换多个 CSS 类以设置特定段落 (<p>) 元素的样式时使用className 属性:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM className</title> <style> .highlight { background-color: lightpink; } .large { font-size: 24px; } .underline { text-decoration: underline; } </style> </head> <body> <p>Click the below button to toggle between the classes</p> <p id="myPara" class="highlight">I am a default paragraph with default styling.!!</p> <button onclick="toggleClass()">Toggle Classes</button> <script> function toggleClass() { const para=document.getElementById('myPara'); if (para.classList.contains('highlight')) { para.className = 'large'; } else if (para.classList.contains('large')){ para.className = 'underline'; } else { para.className = 'highlight'; } } </script> </body> </html>
示例 6:覆盖 Class 属性
以下示例使用className 属性将现有 class 属性覆盖为新的 class 属性:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM className</title> <style> .blue { color: blue; } .red { color: red; } .underline { text-decoration: underline; } </style> </head> <body> <p>Click the buttons to apply different class attributes to this paragraph:</p> <p id="my" class="blue">This paragraph has the class "blue".</p> <button onclick="applyClass('red')">Apply Red Class</button> <button onclick="applyClass('underline')">Apply Underline Class</button> <script> function applyClass(newClass) { const para = document.getElementById('my'); para.className = newClass; } </script> </body> </html>
示例 7:添加类而不覆盖
此示例显示了className 属性的用法。以下代码包括两个按钮,用于向段落 (<p>) 元素添加突出显示和下划线:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM className</title> <style> .highlight { background-color: yellow; } .underline { text-decoration: underline; } </style> </head> <body> <p>Click the buttons to toggle between adding classes to this paragraph:</p> <p id="myP" class="highlight">This paragraph has the class "highlight".</p> <button onclick="addClass('underline')">Add Underline Class</button> <button onclick="addClass('highlight')">Toggle Highlight Class</button> <script> function addClass(newClass) { const para=document.getElementById('myP'); // Check if the class is already present if (!para.classList.contains(newClass)) { // Append class without overwriting para.className += ' ' + newClass; } else { para.classList.remove(newClass); } } </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
className | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告