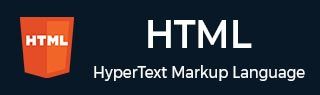
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - Iframes
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 页眉
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 表格查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 clientWidth 属性
**clientWidth** 属性返回元素的宽度,包括内边距,但不包括边距、边框或滚动条宽度。它是一个只读属性,但对于响应式设计和布局计算很有用。
语法
element.clientWidth;
返回值
此属性返回一个数字(以像素为单位),表示元素的可视宽度。
HTML DOM 元素“clientWidth”属性的示例
以下是一些示例,说明了在各种场景中使用 clientWidth 属性。
获取元素的宽度
此示例检索元素的 clientWidth 以显示其内部宽度,不包括边框和滚动条宽度。
<!DOCTYPE html> <html lang="en"> <head> <style> .example-element { width: 390px; border: 2px solid #333; padding: 10px; background-color: lightblue; } </style> </head> <body> <div class="example-element" id="exampleElement"> <h2>Example Element</h2> <p>This element has some content.</p> </div> <div id="output"> <h2>Element Client Width:</h2> <p>Width: <span id="width"></span>pixels</p> </div> <script> document.addEventListener ("DOMContentLoaded", function() { var element = document.getElementById ("exampleElement"); var width = element.clientWidth; document.getElementById("width").textContent= width; }); </script> </body> </html>
获取特定元素的宽度
此示例演示如何访问第一个**<header>** 元素的 clientWidth 以确定其内部宽度,不包括边框和滚动条。
<!DOCTYPE html> <html lang="en"> <head> <style> h1 { border-top: 10px solid black; padding: 10px; } </style> </head> <body> <h1 id="firstHeader">First Header Element</h1> <div id="output"> <h2> Top Border Width of the First Header Element: </h2> <p>Width: <span id="width"></span> pixels</p> </div> <script> document.addEventListener ("DOMContentLoaded", function() { var firstHeader = document.getElementById("firstHeader"); var outputWidth = document.getElementById("width"); function updateHeaderWidth() { var width = firstHeader.clientWidth; outputWidth.textContent = width; } // Initial update updateHeaderWidth(); // Update on window resize window.addEventListener ("resize", updateHeaderWidth); }); </script> </body> </html>
动态更新元素的宽度
此示例演示了当光标移动到容器上时,clientWidth 属性如何动态调整和显示元素在视口上的宽度。然后元素恢复到其原始宽度。
<!DOCTYPE html> <html lang="en"> <head> <style> .box { background-color: lightblue; border: 2px solid blue; text-align: center; line-height: 100px; transition: width 0.3s ease; } </style> </head> <body> <div class="box" id="box">Resize Me!</div> <div id="output">Client Width: <span id="width"> </span> pixels</div> <script> document.addEventListener ("DOMContentLoaded", function() { var box = document.getElementById("box"); var outputWidth = document.getElementById ("width"); // Function to update client width function updateClientWidth() { var width = box.clientWidth; outputWidth.textContent = width; } // Initial update updateClientWidth(); // Update on box resize (simulated) box.addEventListener ("mouseover", function() { box.style.width = (box.clientWidth + 20)+ "px"; updateClientWidth(); }); box.addEventListener("mouseout", function(){ box.style.width = "200px"; updateClientWidth(); }); }); </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
clientWidth | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告