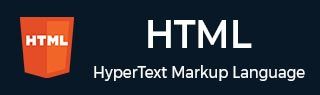
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引号
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - Iframes
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 表格查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 cloneNode() 方法
**cloneNode()** 方法复制一个节点,包括其属性和子节点,这允许动态内容生成,并支持对文档结构进行各种操作。
cloneNode 中的 **“deep”** 参数是可选的,它控制克隆深度:将其值设置为 'true' 执行深度克隆;将其值设置为 'false' 执行浅克隆。
语法
originalNode.cloneNode(deep);
参数
此方法接受如下所示的单个参数。
参数 | 描述 |
---|---|
deep | 布尔值,指示是否在克隆中包含所有子元素,它是可选参数。 |
返回值
此方法返回克隆节点。
HTML DOM 元素 'cloneNode()' 方法示例
以下是一些说明如何使用 cloneNode 复制各种 HTML DOM 元素的示例。
克隆按钮元素
此示例创建按钮的副本,并允许在每次点击 **按钮** 时,按钮元素被克隆并追加到文档主体。
<!DOCTYPE html> <html> <body> <button id="originalButton">Click me</button> <script> var originalButton = document.getElementById('originalButton'); var cloneCount = 0; // Function to handle button click and clone originalButton.addEventListener ('click', function() { // Increment the clone counter cloneCount++; // Clone the original button var clonedButton = originalButton.cloneNode(true); clonedButton.textContent = 'Click me (Clone ' + cloneCount + ')'; document.body.appendChild(clonedButton); }); </script> </body> </html>
克隆列表元素
此示例克隆 **列表** 元素及其子列表项。
<!DOCTYPE html> <html> <head> <style> ul { border: 1px solid #ccc; width: 200px; } button { cursor: pointer; } </style> </head> <body> <ul id="originalList"> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> <button id="cloneButton">Clone List</button> <script> var originalList = document.getElementById('originalList'); var cloneButton = document.getElementById('cloneButton'); cloneButton.addEventListener ('click', function() { var clonedList = originalList.cloneNode(true); // Optional: Assign an id to cloned list clonedList.id = 'clonedList'; document.body.appendChild(clonedList); }); </script> </body> </html>
克隆 div 元素
此示例克隆 **<div>** 元素及其所有子元素,包括文本和其他元素。
<!DOCTYPE html> <html> <head> <title>Clone DIV Element</title> <style> .original { padding: 20px; margin: 10px; border: 1px solid #ccc; text-align: center; } .cloned { padding: 20px; margin: 10px; border: 1px solid #999; text-align: center; } </style> </head> <body> <div class="original" id="originalDiv"> Original DIV (click me to clone) </div> <script> var originalDiv = document.getElementById('originalDiv'); var cloneCount = 0; originalDiv.addEventListener ('click', function() { // Increment the clone counter cloneCount++; // Clone the original div var clonedDiv = originalDiv.cloneNode(true); // Customize the cloned div content clonedDiv.textContent = 'Cloned DIV ' + cloneCount; clonedDiv.classList.add('cloned'); document.body.appendChild(clonedDiv); }); </script> </body> </html>
克隆表格元素
此示例克隆 **表格** 元素及其行和列。
<!DOCTYPE html> <html> <head> <style> table { border-collapse: collapse; margin-bottom: 20px; } th, td { border: 1px solid #ccc; padding: 8px; } button { cursor: pointer; } </style> </head> <body> <table id="originalTable"> <tr> <th>Name</th> <th>Salary</th> </tr> <tr> <td>Nilesh</td> <td>43,000,000</td> </tr> <tr> <td>Nikki</td> <td>43,101,000</td> </tr> </table> <button id="cloneButton">Clone Table</button> <br><br> <script> var originalTable = document.getElementById('originalTable'); var cloneButton = document.getElementById('cloneButton'); cloneButton.addEventListener ('click', function() { var clonedTable = originalTable.cloneNode(true); document.body.appendChild(clonedTable); }); </script> </body> </html>
支持的浏览器
方法 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
cloneNode() | 是 1 | 是 12 | 是 1 | 是 1 | 是 7 |
html_dom_element_reference.htm
广告