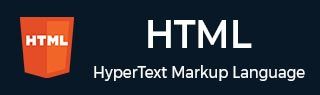
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - Iframes
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 多媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity 绘图
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用的标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM innerHTML 属性
HTML DOM innerHTML 属性用于检索(读取)元素的现有 HTML 内容并使用新的 HTML 内容更新它。
当您访问 element.innerHTML(例如,document.getElementById('id').innerHTML 或任何其他方法)时,它会检索该元素的当前内容。如果您为其分配新内容(例如,element.innerHTML = "new_content"),它会将现有内容更新为新的 HTML。
以下交互式示例演示了innerHTML 方法在不同场景下的用法:
欢迎来到 Tutorialspoint
您来对地方学习了……
语法
以下是 HTML DOM innerHTML(读取内容)属性的语法:
element.innerHTML
要使用新内容更新现有 HTML 内容,请使用以下语法:
element.innerHTML = text
其中“text”是元素的文本内容。
参数
此属性不接受任何参数。
返回值
此属性返回一个包含元素内 HTML 内容的字符串。
示例 1:使用 innerHTML 检索 HTML 内容
以下是 HTML DOM innerHTML 属性的基本示例:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM innerHTML</title> <style> button{ padding: 8px 12px; } </style> </head> <body> <h3>Click the below button to retrieve the "p" element content.</h3> <p id="my_ele">Welcome to Tutorialspoint</p> <button onclick="read()">Read</button> <script> function read(){ let ele = document.getElementById("my_ele"); alert("The 'p' element content is: " + ele.innerHTML); } </script> </body> </html>
以上程序检索“p”元素的 HTML 内容。
示例 2:使用 innerHTML 更新 HTML 内容
以下是 HTML DOM innerHTML 的另一个示例。我们使用此属性在运行时动态更改或更新 HTML 元素中的内容:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM innerHTML</title> <style> button{ padding: 8px 12px; } </style> </head> <body> <p>Click the button below to dynamically update the content of the 'div' element.</p> <p id="heading">Heading</p> <div id="content">Welcome to TP, you are at the right place to learn..</div> <br> <button onclick="changeContent()">Change Content</button> <script> function changeContent() { // Update the heading and content using innerHTML document.getElementById('heading').innerHTML = '<b>New Heading</b>'; document.getElementById('content').innerHTML = '<b>New content</b> has been updated!'; } </script> </body> </html>
以上程序更新了 id 为“heading”和“content”的元素的现有内容。
示例 3:使用“innerHTML”属性清除内容
此示例演示了如何在单击“清除内容”按钮时使用innerHTML 属性动态清除<div> 元素内的内容:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM innerHTML</title> <style> button{ padding: 8px 12px; } </style> </head> <body> <p>Click the below to clear the content.</p> <div id="myDiv">Content to be cleared</div><br> <button onclick="clearContent()">Clear Content</button> <script> function clearContent() { document.getElementById('myDiv').innerHTML = ''; } </script> </body> </html>
示例 4:动态生成内容
下面的示例在单击“生成内容”按钮时使用 JavaScript 和innerHTML 属性动态生成内容:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM innerHTML</title> <style> button{ padding: 8px 10px; } </style> </head> <body> <p>Click the below button to generate content dynamically.</p> <div id="contentArea">Welcome to TP, you are at the right place to learn..</div> <br> <button onclick="generateContent()">Generate Content</button> <script> function generateContent() { let count = 5; let html = ''; for (let i = 1; i <= count; i++) { html += '<p>Paragraph ' + i + '</p>'; } document.getElementById('contentArea').innerHTML = html; } </script> </body> </html>
示例 5:显示条件内容
以下示例显示了在单击“显示内容”按钮时如何使用innerHTML 属性有条件地显示内容:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM innerHTML</title> <style> button{ padding: 8px 12px; } </style> </head> <body> <p>Click the below button to show content.</p> <div id="contentArea">Initial content</div> <br> <button onclick="showContent()">Show Content</button> <script> function showContent() { let condition = true; let content = condition ? '<p>Content is visible</p>' : ''; document.getElementById('contentArea').innerHTML = content; } </script> </body> </html>
示例 6:动态突出显示文本
以下示例帮助我们了解如何使用innerHTML 属性突出显示 HTML 元素中的特定文本:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM innerHTML</title> <style> .highlight { background-color: yellow; font-weight: bold; } button{ padding: 8px 12px; } </style> </head> <body> <p>It Highlights the Text dynamically</p> <div id="myDiv">This text will be highlighted where "inner" occurs.</div> <br> <button onclick="highlightText()">Highlight Text</button> <script> function highlightText() { let searchText = 'inner'; let content = document.getElementById('myDiv').innerHTML; let highlightedContent = content.replace(new RegExp(searchText,'gi'),'<span class="highlight">$&</span>'); document.getElementById('myDiv').innerHTML = highlightedContent; } </script> </body> </html>
示例 7:动态创建表格
以下示例演示了如何使用 HTML 结构动态创建一个 HTML 表格,并在构建后使用innerHTML 属性显示该表格:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM innerHTML</title> <style> button{ padding: 8px 12px; } table, th, td{ width: 33%; text-align: center; padding: 10px; } </style> </head> <body> <p>Click the below button to create an HTML table.</p> <div id="tableContainer"> </div> <br> <button onclick="createTable()">Create Table</button> <script> function createTable() { let tableHTML = '<table border="1"><tr><th>Name</th><th>Age</th></tr>'; let data = [ { name: 'Alice', age: 25 }, { name: 'Bob', age: 30 }, { name: 'Carol', age: 28 } ]; data.forEach(item => { tableHTML += `<tr><td>${item.name}</td><td> ${item.age}</td></tr>`; }); tableHTML += '</table>'; document.getElementById('tableContainer').innerHTML = tableHTML; } </script> </body> </html>
示例 8:根据用户输入创建消息
此示例显示了如何使用innerHTML 属性根据用户输入动态创建消息:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM innerHTML</title> <style> body { text-align: center; } label { font-size: 18px; } input[type="text"] { padding: 8px; font-size: 16px; border: 1px solid #ccc; } button { padding: 10px 20px; font-size: 16px; background-color: #4CAF50; border: none; cursor: pointer; margin-top :10px; } button:hover { background-color: #45a049; } #messageBox { margin-top: 20px; padding: 20px; background-color: #f0f0f0; border: 1px solid #ccc; } </style> </head> <body> <h3>Creates Message based on User Input</h3> <h1>Welcome to Tutorialspoint</h1> <label for="nameInput">Enter your name:</label> <input type="text" id="nameInput"> <button onclick="createMessage()">Create Message</button> <div id="messageBox"></div> <script> function createMessage() { let name = document.getElementById("nameInput").value; let message = `<p>Hello <b>${name}</b> ! Welcome to Tutorialspoint.</p>`; document.getElementById("messageBox").innerHTML = message; } </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
innerHTML | 是 | 是 | 是 | 是 | 是 |