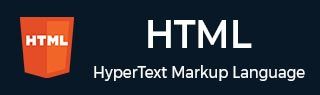
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - Iframes
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 页眉
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用的标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM lastChild 属性
HTML DOM 的lastChild 属性用于访问和检索 HTML DOM 中指定父元素的最后一个子节点。
此属性对于操作 DOM 很有用,因为它允许开发人员轻松识别和交互最后一个子节点,无论它是元素节点、文本节点还是注释。
如果父元素没有子元素,则 lastChild 将返回null。
语法
以下是 HTML DOM lastChild 属性的语法:
element.lastChild;
参数
因为它是一个属性,所以它不接受任何参数。
返回值
此属性返回一个指向特定父元素的最后一个子节点的节点。如果不存在最后一个子节点,则返回“null”。
示例 1:访问 Div 元素的 lastChild
以下是 HTML DOM lastChild 属性的基本示例。它检索<div> 元素的最后一个子元素:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM lastChild</title> </head> <body> <div id="parent"> <span>First Span element</span> <div>Second div</div> <p>Last paragraph</p> </div> <p>Click the below button to get the last child of div element.</p> <button onclick="showLastChild()">Click me</button> <div id="output"></div> <script> function showLastChild() { // Selecting the parent div const parentDiv = document.getElementById('parent'); // Retrieving the last child node const lastChildNode = parentDiv.lastElementChild; // Displaying the result const outputDiv = document.getElementById('output'); outputDiv.innerHTML = `Last child node: ${lastChildNode.textContent}`; } </script> </body> </html>
上面的程序返回“div”元素的最后一个子元素。
示例 2:“ul”元素的最后一个子节点
以下是 HTML DOM lastChild 元素的另一个示例。我们使用此属性来访问<ul> 元素的最后一个子元素:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM lastChild</title> <style> ul { padding: 0; } li { border-bottom: 1px solid #ccc; padding: 5px; } </style> </head> <body> <h4>Below are List of ul items :</h4> <ul id="myList"> <li>HTML</li> <li>CSS</li> <li>JavaScript</li> <li>DSA</li> </ul> <button onclick="showLastChild()">Get the last child</button> <div id="output"></div> <script> function showLastChild() { // Selecting the ul element const myList = document.getElementById('myList'); // Displaying the last child content const outputDiv = document.getElementById('output'); outputDiv.textContent = `Last child: ${myList.lastElementChild.textContent}`; } </script> </body> </html>
示例 3:修改 Div 元素的最后一个子元素内容
此示例演示如何使用lastChild 属性动态修改 <div> 元素的最后一个子元素的内容:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM lastChild</title> </head> <body> <h4>This is the div content with their child nodes:</h4> <div id="parent"> <p>First paragraph</p> <div>Second div</div> <span>Span element</span> <p>Last paragraph</p> </div> <h3>Modified last child node:</h3> <div id="output"></div> <script> // Selecting the parent div const parentDiv = document.getElementById('parent'); // Accessing and modifying the last child node const lastChildNode = parentDiv.lastChild; // Displaying the updated content const outputDiv = document.getElementById('output'); outputDiv.textContent = `Updated child node is: ${'Modified last paragraph'}.`; </script> </body> </html>
示例 4:动态插入最后一个子元素
下面的示例演示了如何使用lastChild 属性在单击按钮时将新的最后一个子元素动态插入项目列表中:
<!DOCTYPE html> <html lang="en"> <head> <title>HTML DOM lastChild</title> <style> .container { border: 1px solid #ccc; } .highlight { background-color: #ffffcc; font-weight: bold; } </style> </head> <body> <p>Inserting a new Last Child dynamically..</p> <div id="pt" class="container"> <p>First paragraph</p> <ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> <span>Span element</span> <p>Last paragraph with some whitespace before closing tag</p> </div> <div class="container"> <button onclick="insertLastChild()">Insert Last Child</button></div> <script> // Function to insert a new last child element function insertLastChild() { const pDiv = document.getElementById('pt'); const lastChild = pDiv.lastChild; // Create a new element const newElement = document.createElement('p'); newElement.textContent = 'New last child paragraph'; // Insert the new element as the last child pDiv.insertBefore(newElement, lastChild.nextSibling); newElement.classList.add('highlight'); } </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
lastChild | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告