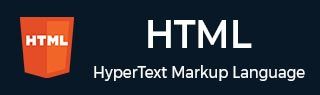
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图像地图
- HTML - 内联框架
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 页眉
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - 地理位置 API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 表格查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用的标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 nodeName 属性
nodeName 属性根据节点的内容识别节点的类型和名称。它以字符串的形式给出节点的名称,该名称会随着内容类型的变化而变化。
- 对于元素节点,它以大写形式给出标签名称。
- 对于注释节点,它给出 #comment。
- 对于文本节点,它给出 #text。
语法
element.nodeName;
返回值
nodeName 属性返回一个包含节点名称的字符串,该名称基于其内容类型:对于元素节点,它返回大写形式的标签名称;对于文本节点,它返回 #text;对于注释节点,它返回 #comment。
HTML DOM 元素 'nodeName' 属性示例
以下是一些示例,用于更好地理解 'nodeName' 属性的用法。
显示 HTML 元素的节点名称
此示例演示如何使用 nodeName 属性访问和显示各种 HTML 元素的节点名称。
<!DOCTYPE html> <html lang="en"> <head> <style> .highlight { color: red; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>nodeName Property</h2> <p>Displays the node names of various elements.</p> <div id="otpt"></div> <script> // Selecting h1, h2, and p elements const ele=document.querySelectorAll('h1, h2,p'); const outputDiv=document.getElementById('otpt'); ele.forEach(element => { const nodeName = element.nodeName; const nodeDescription = document.createElement('p'); nodeDescription.textContent = `Node name of <${nodeName}> element: ${nodeName}`; nodeDescription.classList.add('highlight'); outputDiv.appendChild(nodeDescription); }); </script> </body> </html>
根据节点类型访问和设置样式
此示例演示了 nodeName 属性在访问具有 id “pt” 的父节点的<div>元素及其元素类型方面的用法,然后通过显示其节点类型相应地设置其样式。
<!DOCTYPE html> <html lang="en"> <head> <style> .highlight { color: blue; font-weight: bold; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>nodeName Property</h2> <p>Updates element's style based on node type.</p> <p>Contents of div element with id = "pt" with their different node types: </p> <div id="pt"> <div>First div</div> <span>Span element</span> </div> <div id="ot"></div> <script> const parentDiv =document.getElementById('pt'); const childNodes = parentDiv.childNodes; const outputDiv =document.getElementById('ot'); childNodes.forEach(node => { const nodeName = node.nodeName; //New element for displaying node information const nodeDes=document.createElement('p'); // Customize style based on node type if (nodeName === 'DIV') { nodeDes.textContent=`Div element found: ${node.textContent}`; nodeDes.classList.add('highlight'); } else if (nodeName === 'SPAN') { nodeDes.textContent=`Span element found: ${node.textContent}`; } outputDiv.appendChild(nodeDes); }); </script> </body> </html>
显示 body 元素的节点名称
此示例演示了如何使用 'nodeName' 属性显示<body>元素内的所有元素。
<!DOCTYPE html> <html lang="en"> <head> <title>nodeName Property Example</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>nodeName Property</h2> <p>Displaying the node names of all <body> elements. </p> <div id="ot"></div> <script> // Selecting the <body> element const bodyElement = document.body; // Getting all child nodes of <body> const childNodes=bodyElement.childNodes; // Creating a div element for output const outputDiv=document.getElementById('ot'); // Iterating over child nodes childNodes.forEach(node => { if (node.nodeType === Node.ELEMENT_NODE) { const noNDes=document.createElement('p'); noNDes.textContent=`Node name: ${node.nodeName}`; outputDiv.appendChild(noNDes); } }); </script> </body> </html>
访问节点名称、值和类型
此示例通过使用 HTML DOM 元素的 nodeName 属性,帮助我们获取具有 ID “myDiv” 的<div>元素中第一个子节点的节点名称、值和类型。
<!DOCTYPE html> <html lang="en"> <head> <title>Get Node Name, Value, and Type Example</title> </head> <body> <h1>HTML - DOM Element</h1> <h2>Get Node Name, Value, and Type</h2> <p>Displaying node name, value, and type of first child. </p> <div id="myDIV"> <p>This is the first child node.</p> </div> <div id="ot"></div> <script> // Selecting the element with id "myDIV" const myDiv = document.getElementById('myDIV'); // Getting the first child node const firstChild = myDiv.firstChild; // Creating elements to display results const outputDiv = document.getElementById('ot'); // Node name const nodeDes = document.createElement('p'); nodeDes.textContent = `Node name of first child node: ${firstChild.nodeName}`; outputDiv.appendChild(nodeDes); // Node value (for text nodes) if (firstChild.nodeType === Node.TEXT_NODE) { const nodeval = document.createElement('p'); nodeval.textContent = `Node value of first child node:This is "myDIV".`; outputDiv.appendChild(nodeval); } // Node type const nodedes = document.createElement('p'); nodedes.textContent=`Node type of first child node: ${firstChild.nodeType}`; outputDiv.appendChild(nodedes); </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
nodeName | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告