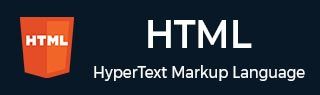
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - Iframes
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表格标题和说明
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 页眉
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 表格查询
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 offsetWidth 属性
元素的 offsetWidth 属性会返回该元素在网页上可见的总宽度,包括内容宽度、水平填充和边框,以像素为单位。
语法
element.offsetWidth
返回值
offsetWidth 属性返回一个整数,表示元素的总像素宽度,包括其填充、边框和可选的滚动条宽度。
HTML DOM 元素 'offsetWidth' 属性示例
以下是一些示例,展示了 'offsetWidth' 属性的使用,以便更好地理解。
点击按钮时的偏移宽度
此示例演示了如何使用 offsetWidth 属性获取元素的宽度。以下代码包含一个具有类 element 的<div> 元素和一个按钮。点击按钮时,它会显示计算出的元素宽度。
<!DOCTYPE html> <html lang="en"> <head> <style> #exampleElement { width: 200px; padding: 10px; border: 1px solid black; background-color: lightblue; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>offsetHeight Property</h2> <div id="exampleElement">Example Element</div> <button onclick="showWidth()"> Show Width </button> <script> function showWidth() { const element = document.getElementById('exampleElement'); const width = element.offsetWidth; alert(`Width of the element: ${width}px`); } </script> </body> </html>
带有填充和边框的偏移宽度
此示例演示了如何使用 offsetWidth 属性获取网页上元素的总计算宽度,包括其填充和边框。
<!DOCTYPE html> <html lang="en"> <head> <style> #ex{ width: 200px; padding: 20px; border: 5px solid black; background-color: lightblue; text-align: center; } #wi { color: green; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>offsetWidth Property</h2> <div id="ex">Example Element</div> <br> <button onclick="showWidth()"> Show Width including Padding and Border </button> <div id="wi"></div> <script> const exampleElement=document.getElementById('ex'); const widthDisplay = document.getElementById('wi'); function showWidth() { const widthWithPaddingBorder = exampleElement.offsetWidth; widthDisplay.textContent = `Width including padding and border: ${widthWithPaddingBorder}px`; } </script> </body> </html>
动态计算滚动条宽度
此示例演示了如何使用 offsetWidth 属性动态计算网页上滚动条元素的宽度,包括其填充和边框。
<!DOCTYPE html> <html lang="en"> <head> <style> #scroll { width: 200px; height: 200px; overflow: scroll; border: 1px solid black; padding: 20px; } #cont { width: 100%; height: 400px; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>offsetHeight Property</h2> <div id="scroll"> <div id="cont">Scrollable Content</div> </div> <button onclick="calculate()"> Calculate Scrollbar Width </button> <div id="scrollbar"></div> <script> function calculate () { const scCon = document.getElementById('scroll'); const co = document.getElementById('content'); const scwid = scCon.offsetWidth-cont.clientWidth; const scD= document.getElementById('scrollbar'); scD.textContent = `Scrollbar width: ${scwid}px`; } </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
offsetWidth | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告