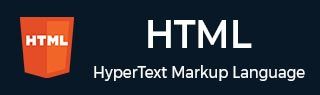
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与演变
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图像地图
- HTML - Iframes
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮件链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 页眉
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 杂项
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity Draw
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 表格查找
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 scrollHeight 属性
**scrollHeight** 属性以像素为单位提供元素内容的总垂直高度。它包括溢出和填充,但不包括边框、边距和滚动条。
语法
element.scrollHeight;
返回值
scrollHeight 属性返回一个整数,该整数包含元素内容的总垂直高度(以像素为单位)。
HTML DOM 元素“scrollHeight”属性的示例
以下是一些示例,这些示例显示了如何使用“scrollHeight”属性来获取元素内容的总垂直高度(以像素为单位)。
确定可滚动容器的高度
此示例显示了如何使用 scrollHeight 属性来确定可滚动容器内内容的总高度。代码会在 **<span>** 元素中动态更新显示的高度。
<!DOCTYPE html> <html lang="en"> <head> <style> #container { height: 100px; overflow: auto; border: 1px solid #ccc; } #content { height: 200px; background-color: #f0f0f0; padding: 10px; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>scrollHeight Property</h2> <div> <p>Determines the height of content inside a scrollable container: </p> <div id="container"> <div id="content"> This is the content inside the div element. <br>scroll me!! </div> </div> <p> Scrollable content height:<span id="r1"></span> </p> </div> <script> const c1 = document.getElementById('container'); const res = document.getElementById('r1'); res.textContent = c1.scrollHeight; </script> </body> </html>
动态显示可滚动内容的高度
此示例显示了一个可滚动框(**<div>**),用户可以通过在其中单击来编辑内容。它包含一个按钮,该按钮使用 scrollHeight 属性动态显示框的可滚动总高度。
<!DOCTYPE html> <html lang="en"> <head> <style> #cont{ height: 30px; overflow: auto; border: 1px solid black; padding: 10px; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>scrollHeight Property</h2> <div> <p>Click the button to display the total scrollable height of the container: </p> <p>Try editing the content inside the container</p> <div id="cont" contenteditable="true"> Click here to edit!! Content that exceeds the container height. </div> <br> <button onclick="showScrollHeight()"> Show Scrollable Height </button> <p>Scrollable content height: <span id="r"></span> </p> </div> <script> function showScrollHeight() { const con = document.getElementById('cont'); const res = document.getElementById('r'); res.textContent = con.scrollHeight + 'px'; } </script> </body> </html>
动态调整 Textarea 高度
此示例显示了当您键入时 scrollHeight 属性如何调整 Textarea 的高度。Textarea 的高度会更新以适应文本,并且显示的“当前高度”会根据其内容以像素为单位显示实际高度。
<!DOCTYPE html> <html lang="en"> <head> <style> #textcon{ width: 300px; margin: 20px; } textarea { width: 100%; min-height: 50px; border: 1px solid #ccc; padding: 10px; box-sizing: border-box; transition: height 0.3s; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>scrollHeight Property</h2> <h4>Adjusts height based on scrollable content.</h4> <div id="textcon"> <p>Type in the textarea to see it expand or compress height dynamically: </p> <textarea id="m" oninput="autoAdjustTextarea(this)"> </textarea> <p>Current height: <span id="ch">50px</span></p> </div> <script> function autoAdjustTextarea(textarea) { textarea.style.height = 'auto'; textarea.style.height = textarea.scrollHeight + 'px'; document.getElementById('ch').textContent = textarea.style.height; } </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
scrollHeight | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告