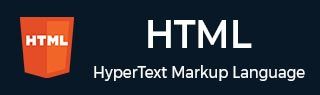
- HTML 教程
- HTML - 首页
- HTML - 路线图
- HTML - 简介
- HTML - 历史与发展
- HTML - 编辑器
- HTML - 基本标签
- HTML - 元素
- HTML - 属性
- HTML - 标题
- HTML - 段落
- HTML - 字体
- HTML - 块
- HTML - 样式表
- HTML - 格式化
- HTML - 引用
- HTML - 注释
- HTML - 颜色
- HTML - 图片
- HTML - 图片地图
- HTML - Iframes
- HTML - 短语元素
- HTML - 元标签
- HTML - 类
- HTML - ID
- HTML - 背景
- HTML 表格
- HTML - 表格
- HTML - 表头和标题
- HTML - 表格样式
- HTML - 表格 Colgroup
- HTML - 嵌套表格
- HTML 列表
- HTML - 列表
- HTML - 无序列表
- HTML - 有序列表
- HTML - 定义列表
- HTML 链接
- HTML - 文本链接
- HTML - 图片链接
- HTML - 邮箱链接
- HTML 颜色名称和值
- HTML - 颜色名称
- HTML - RGB
- HTML - HEX
- HTML - HSL
- HTML 表单
- HTML - 表单
- HTML - 表单属性
- HTML - 表单控件
- HTML - 输入属性
- HTML 媒体
- HTML - 视频元素
- HTML - 音频元素
- HTML - 嵌入多媒体
- HTML 头部
- HTML - Head 元素
- HTML - 添加 Favicon
- HTML - Javascript
- HTML 布局
- HTML - 布局
- HTML - 布局元素
- HTML - 使用 CSS 进行布局
- HTML - 响应式设计
- HTML - 符号
- HTML - 表情符号
- HTML - 样式指南
- HTML 图形
- HTML - SVG
- HTML - Canvas
- HTML API
- HTML - Geolocation API
- HTML - 拖放 API
- HTML - Web Workers API
- HTML - WebSocket
- HTML - Web 存储
- HTML - 服务器发送事件
- HTML 其他
- HTML - 文档对象模型 (DOM)
- HTML - MathML
- HTML - 微数据
- HTML - IndexedDB
- HTML - Web 消息传递
- HTML - Web CORS
- HTML - Web RTC
- HTML 演示
- HTML - 音频播放器
- HTML - 视频播放器
- HTML - 网页幻灯片
- HTML 工具
- HTML - Velocity 绘图
- HTML - 二维码
- HTML - Modernizer
- HTML - 验证
- HTML - 颜色拾取器
- HTML 参考
- HTML - 速查表
- HTML - 标签参考
- HTML - 属性参考
- HTML - 事件参考
- HTML - 字体参考
- HTML - ASCII 码
- ASCII 码表查询
- HTML - 颜色名称
- HTML - 实体
- MIME 媒体类型
- HTML - URL 编码
- 语言 ISO 代码
- HTML - 字符编码
- HTML - 已弃用的标签
- HTML 资源
- HTML - 快速指南
- HTML - 有用资源
- HTML - 颜色代码生成器
- HTML - 在线编辑器
HTML - DOM 元素 scrollLeft 属性
scrollLeft 属性允许您访问和更新元素内容水平滚动的距离,以像素为单位测量元素左边缘的距离。
语法
设置 scrollLeft 属性值element.scrollLeft = pixelValue;获取 scrollLeft 属性值
element.scrollLeft;
属性值
值 | 描述 |
---|---|
pixelValue | 设置元素水平滚动位置的像素值。
|
返回值
scrollLeft 属性返回一个整数,表示以像素为单位的水平滚动位置。
HTML DOM 元素 'scrollLeft' 属性示例
以下是一些示例,展示了如何使用 'scrollLeft' 属性调整 HTML DOM 中元素的水平滚动位置。
'scrollLeft' 属性的基本用法
此示例演示了 scrollLeft 属性的基本用法,以编程方式控制 HTML 中水平可滚动容器的初始滚动位置。页面加载时,容器将默认向右滚动 200 像素。
<!DOCTYPE html> <html lang="en"> <head> <style> #con { width: 300px; overflow-x: scroll; white-space: nowrap; border: 1px solid #ccc; } .box { display: inline-block; width: 350px; height: 200px; margin: 30px; background-color: Ffb6c1; text-align: center; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>scrollLeft property</h2> <p>Scroll left and right using arrows!</p> <div id="con"> <div class="box">1</div> <div class="box">2</div> <div class="box">3</div> </div> <script> var co = document.getElementById('con'); // Set scrollLeft to 200px on load co.scrollLeft = 200; </script> </body> </html>
使用 scrollLeft 属性创建水平滚动
此示例包含一个具有固定宽度和高度的 <a href="/html/html_div_tag.htm" target="_blank"><div>,用于创建水平项目行。定义了用于处理左右滚动的函数。“向左滚动”按钮单击时,可见内容将向左移动;“向右滚动”按钮单击时,可见内容将向右移动,都使用 scrollLeft 属性。
<!DOCTYPE html> <html lang="en"> <head> <style> .scroll-container { width: 400px; overflow-x: scroll; white-space: nowrap; } .scroll-item { display: inline-block; width: 200px; height: 150px; margin-right: 10px; background-color: #00fff0; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>scrollLeft Property</h2> <div class="scroll-container" id="sccon"> <div class="scroll-item">Item 1</div> <div class="scroll-item">Item 2</div> <div class="scroll-item">Item 3</div> <div class="scroll-item">Item 4</div> <div class="scroll-item">Item 5</div> </div> <button onclick="scrollLeft()"> Scroll Left </button> <button onclick="scrollRight()"> Scroll Right </button> <script> function scrollLeft() { var con = document.getElementById("sccon"); con.scrollLeft -= 50; } function scrollRight() { var con = document.getElementById("sccon"); con.scrollLeft += 50; } </script> </body> </html>
动态水平滚动控制
此代码示例包含一个具有固定宽度和水平滚动条的可滚动容器。它包含一个函数,用于将容器滚动到开头和结尾,并动态更新滚动位置。
<!DOCTYPE html> <html lang="en"> <head> <style> #scroll { width: 300px; overflow-x: scroll; white-space: nowrap; border: 1px solid #bbb; } .box { display: inline-block; width: 100px; margin: 10px; background-color: #f0fff0; text-align: center; line-height: 100px; } #scrollInfo { font-weight: bold; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>scrollLeft property</h2> <div id="scroll"> <div class="box">1</div> <div class="box">2</div> <div class="box">3</div> <div class="box">4</div> <div class="box">5</div> </div> <div id="scInfo">Scroll Left: 0</div> <div> <button onclick="scrollToStart()"> Scroll to Start </button> <button onclick="scrollToEnd()"> Scroll to End </button> </div> <script> var scd=document.getElementById('scroll'); var sInfo=document.getElementById('scInfo'); function updateScrollInfo() { sInfo.textContent = 'Scroll Left: ' + scd.scrollLeft; } function scrollToStart() { scd.scrollLeft = 0; updateScrollInfo(); } function scrollToEnd() { scd.scrollLeft = scd.scrollWidth - scd.clientWidth; updateScrollInfo(); } // Update scroll info initially updateScrollInfo(); // Update scroll info on scroll scd.addEventListener ('scroll',updateScrollInfo); </script> </body> </html>
使用输入进行向左滚动
此示例演示如何使用 scrollLeft 属性在固定宽度容器内启用交互式水平滚动。用户可以在输入字段中输入像素值,然后单击按钮,使容器向左滚动该数量的像素。
<!DOCTYPE html> <html lang="en"> <head> <style> #container { width: 300px; overflow-x: scroll; white-space: nowrap; border: 1px solid #ccc; } .box { display: inline-block; width: 100px; height: 100px; margin: 10px; background-color: #f9f0f9; text-align: center; line-height: 100px; } </style> </head> <body> <div id="container"> <div class="box">1</div> <div class="box">2</div> <div class="box">3</div> <div class="box">4</div> <div class="box">5</div> </div> <div> <label for="scrollAmount"> Scroll Left by: </label> <input type="number" id="scrollAmount"value="50"> <button onclick="scrollLeftByAmount()"> Scroll </button> </div> <script> var container = document.getElementById ('container'); var scrollAmountInput = document.getElementById('scrollAmount'); function scrollLeftByAmount() { var amount = parseInt (scrollAmountInput.value, 10) || 0; container.scrollLeft -= amount; } </script> </body> </html>
带有滚动位置跟踪器的水平可滚动容器
此示例演示如何使用 CSS 创建水平可滚动容器,并使用 scrollLeft 属性动态跟踪滚动位置。以下代码包含一个具有固定宽度的 <div> 元素,以启用水平滚动。
<!DOCTYPE html> <html lang="en"> <head> <style> #con { width: 300px; overflow-x: scroll; white-space: nowrap; border: 1px solid black; } .box { display: inline-block; width: 100px; margin: 10px; background-color: #fff0ff; text-align: center; line-height: 100px; } </style> </head> <body> <h1>HTML - DOM Element</h1> <h2>scrollLeft property</h2> <p>scroll to get the real-time pixel value..</p> <div id="con"> <div class="box">1</div> <div class="box">2</div> <div class="box">3</div> <div class="box">4</div> <div class="box">5</div> </div> <div id="scrI">Scroll Left: 0</div> <script> var ct= document.getElementById('con'); var scrI = document.getElementById('scrI'); // Update scroll info on scroll ct.addEventListener('scroll', function(){ scrI.textContent = 'Scroll Left: ' + ct.scrollLeft; }); </script> </body> </html>
支持的浏览器
属性 | ![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
scrollLeft | 是 | 是 | 是 | 是 | 是 |
html_dom_element_reference.htm
广告